backup
@ -0,0 +1,3 @@
|
||||
/static/img
|
||||
/static/html
|
||||
/static/video
|
@ -0,0 +1,122 @@
|
||||
# IMPORT
|
||||
import os
|
||||
|
||||
from flask import Flask, render_template, request, url_for, redirect, jsonify, abort, send_from_directory
|
||||
import markdown
|
||||
import frontmatter
|
||||
from datetime import datetime
|
||||
|
||||
|
||||
# FUNCTIONS
|
||||
|
||||
|
||||
def list_files(folder, remove_ext=False):
|
||||
''' Read all the functions in a folder '''
|
||||
names = []
|
||||
for entry in os.scandir(folder):
|
||||
# add to the list only proper files
|
||||
if entry.is_file(follow_symlinks=False):
|
||||
# remove the extension from the filename
|
||||
n = os.path.splitext(entry.name)[0]
|
||||
if remove_ext:
|
||||
n = entry.name
|
||||
names.append(n)
|
||||
return names
|
||||
|
||||
|
||||
def list_folders(folder):
|
||||
''' Return all the folders in a folder '''
|
||||
names = []
|
||||
for entry in os.scandir(folder):
|
||||
# add to the list only proper files
|
||||
if not entry.name.startswith('.') and entry.is_dir():
|
||||
# remove the extension from the filename
|
||||
names.append(entry.name)
|
||||
return names
|
||||
|
||||
|
||||
def get_md_contents(filename, directory='./contents'):
|
||||
''' Return contents from a filename as frontmatter handler '''
|
||||
with open(f"{directory}/{filename}", "r") as f:
|
||||
metadata, content = frontmatter.parse(f.read())
|
||||
html_content = markdown.markdown(content, extensions=['markdown.extensions.attr_list','markdown.extensions.codehilite','markdown.extensions.fenced_code'])
|
||||
return metadata, html_content
|
||||
|
||||
|
||||
# FLASK APP
|
||||
base_url = "~kamo"
|
||||
projects = 'projects'
|
||||
|
||||
# create flask application
|
||||
app = Flask(__name__,
|
||||
static_url_path=f'/soupboat/{base_url}/static',
|
||||
static_folder=f'/soupboat/{base_url}/static')
|
||||
# Markdown(app, extensions=['extra'])
|
||||
# app.jinja_env.extend(jinja2_highlight_cssclass = 'codehilite')
|
||||
|
||||
|
||||
# add the base_url variable to all the flask templates
|
||||
@app.context_processor
|
||||
def set_base_url():
|
||||
return dict(base_url=base_url)
|
||||
|
||||
|
||||
# Homepage
|
||||
@app.route(f"/{base_url}/")
|
||||
def home_page():
|
||||
|
||||
# get the basic info of the website from the /contents/home.md file
|
||||
meta, content = get_md_contents("home.md")
|
||||
projects_list = []
|
||||
for project in list_folders("./projects"):
|
||||
project_info = get_md_contents("documentation.md",
|
||||
f"./{projects}/{project}")[0]
|
||||
project_date = datetime.strptime(project_info['date'], '%d/%m/%Y')
|
||||
project_info['date'] = datetime.strftime(project_date, '%d %b, %y')
|
||||
project_info['categories'].sort()
|
||||
|
||||
project_info['slug'] = project
|
||||
projects_list.append(project_info)
|
||||
|
||||
projects_list.sort(reverse=True, key=lambda project: datetime.strptime(
|
||||
project['date'], '%d %b, %y'))
|
||||
|
||||
# get the list of the projects, the functions, and the corpora
|
||||
home = {
|
||||
**meta,
|
||||
"content": content,
|
||||
"projects": projects_list
|
||||
}
|
||||
return render_template("home.html", **home)
|
||||
|
||||
|
||||
# For generic pages we can include a common template and change only the contents
|
||||
@app.route(f"/{base_url}/<slug>/")
|
||||
def dynamic_page(slug=None):
|
||||
# meta is a dictionary that contains all the attributes in the markdown file (ex: title, description, soup, etc)
|
||||
# content is the body of the md file aka the text content
|
||||
# in this way we can access those frontmatter attributes in jinja simply using the variables title, description, soup, etc
|
||||
meta, content = get_md_contents(f"{slug}.md")
|
||||
return render_template("page.html", **meta, content=content)
|
||||
|
||||
|
||||
# Single project
|
||||
@app.route(f"/{base_url}/projects/<project>/")
|
||||
def p_info(project=None):
|
||||
meta, content = get_md_contents("documentation.md",
|
||||
f"./{projects}/{project}")
|
||||
template = 'project.html'
|
||||
if 'template' in meta:
|
||||
template = meta['template']
|
||||
return render_template(template, **meta, content=content)
|
||||
|
||||
|
||||
|
||||
|
||||
@app.route(f'/{base_url}/projects/<project>/<path:filename>')
|
||||
def sendStaticFiles(project, filename):
|
||||
return send_from_directory(app.root_path + f'/projects/{project}/', filename, conditional=True)
|
||||
|
||||
|
||||
# RUN
|
||||
app.run(port="3132")
|
@ -1,35 +0,0 @@
|
||||
let background = document.getElementById("background");
|
||||
let stanze = document.getElementsByClassName("stanza");
|
||||
let reset = document.getElementById("reset");
|
||||
|
||||
reset.addEventListener("click", (e) => {
|
||||
quotes = getQuotes();
|
||||
lifeboat = lifeboats();
|
||||
offset = Math.floor((Math.random() * background.innerHTML.length) / 2);
|
||||
});
|
||||
|
||||
let sea = background.innerHTML;
|
||||
let quotes = getQuotes();
|
||||
let lifeboat = lifeboats();
|
||||
let offset = 0;
|
||||
|
||||
setInterval(() => moveText(), 100);
|
||||
|
||||
function getQuotes() {
|
||||
let stanza = stanze[Math.floor(Math.random() * stanze.length)];
|
||||
return stanza.getElementsByClassName("quote");
|
||||
}
|
||||
|
||||
function lifeboats() {
|
||||
let boats = "";
|
||||
Array.from(quotes).forEach((quote) => {
|
||||
boats += quote.outerHTML;
|
||||
});
|
||||
return boats;
|
||||
}
|
||||
|
||||
function moveText() {
|
||||
let text = sea.slice(0, offset) + lifeboat + sea.slice(offset);
|
||||
background.innerHTML = text;
|
||||
offset++;
|
||||
}
|
@ -1,111 +0,0 @@
|
||||
html,
|
||||
body {
|
||||
margin: 0;
|
||||
font-size: 1.125rem;
|
||||
line-height: 1.4;
|
||||
}
|
||||
|
||||
.title {
|
||||
text-align: center;
|
||||
margin-top: 32px;
|
||||
margin-bottom: 0;
|
||||
}
|
||||
|
||||
.meta {
|
||||
text-align: center;
|
||||
margin-bottom: 32px;
|
||||
}
|
||||
|
||||
.contents {
|
||||
padding: 0 32px;
|
||||
}
|
||||
|
||||
.contents > * {
|
||||
max-width: 800px;
|
||||
margin: 0 auto;
|
||||
}
|
||||
|
||||
.intro {
|
||||
background-color: tomato;
|
||||
border-radius: 16px;
|
||||
padding: 16px;
|
||||
margin: 16px auto;
|
||||
}
|
||||
.intro h2 {
|
||||
margin-top: 0;
|
||||
margin-bottom: 16px;
|
||||
font-weight: normal;
|
||||
}
|
||||
|
||||
.process h2,
|
||||
.process p {
|
||||
text-align: center;
|
||||
}
|
||||
|
||||
ul {
|
||||
margin: 0;
|
||||
padding: 0;
|
||||
list-style: none;
|
||||
}
|
||||
|
||||
li + li {
|
||||
margin-top: 1em;
|
||||
}
|
||||
|
||||
a {
|
||||
color: tomato;
|
||||
}
|
||||
|
||||
a::after {
|
||||
content: "⤴";
|
||||
}
|
||||
|
||||
.process {
|
||||
margin: 32px auto;
|
||||
}
|
||||
|
||||
.demo {
|
||||
margin: 0 auto;
|
||||
max-width: 1200px;
|
||||
margin-top: 32px;
|
||||
font-size: 1.5rem;
|
||||
}
|
||||
|
||||
#reset {
|
||||
display: block;
|
||||
margin: 0 auto;
|
||||
border: none;
|
||||
background: none;
|
||||
text-align: center;
|
||||
font-size: 1.125rem;
|
||||
color: tomato;
|
||||
text-decoration: underline wavy tomato 2px;
|
||||
|
||||
cursor: pointer;
|
||||
|
||||
transition: width 10s ease-out;
|
||||
width: 100px;
|
||||
}
|
||||
|
||||
#reset:hover {
|
||||
width: 400px;
|
||||
}
|
||||
|
||||
.background {
|
||||
color: currentColor;
|
||||
margin: 32px;
|
||||
line-height: 1.6;
|
||||
text-align: justify;
|
||||
word-break: break-all;
|
||||
}
|
||||
|
||||
.quote {
|
||||
background-color: white;
|
||||
color: tomato;
|
||||
padding: 0 0.3em;
|
||||
border-radius: 1em;
|
||||
}
|
||||
|
||||
.hidden {
|
||||
display: none;
|
||||
}
|
@ -1,21 +0,0 @@
|
||||
html {
|
||||
font-size: 2rem;
|
||||
}
|
||||
|
||||
.text {
|
||||
text-align: justify;
|
||||
max-width: 60ch;
|
||||
margin: 50px auto;
|
||||
}
|
||||
|
||||
span::after {
|
||||
content: " ";
|
||||
}
|
||||
|
||||
.text1 {
|
||||
color: red;
|
||||
}
|
||||
|
||||
.text2 {
|
||||
color: blue;
|
||||
}
|
@ -1 +0,0 @@
|
||||
Violence lands. Hundreds of troops break into a city. At that moment the city starts recording its pain. Bodies are torn and punctured. Inhabitants memorise the assault in stutters and fragments refracted by trauma. Before the Internet is switched off, thousands of phone cameras light up. People risk their lives to record the hell surrounding them. As they frantically call and text each other, their communication erupts into hundreds of star-shaped networks. Others throw signals into the void of social media and encrypted messaging, hoping they will be picked up by someone. Meanwhile, the environment captures traces. Unpaved ground registers the tracks of long columns of armoured vehicles. Leaves on vegetation receive the soot of their exhaust while the soil absorbs and retains the identifying chemicals released by banned ammunition. The broken concrete of shattered homes records the hammering collision of projectiles. Pillars of smoke and debris are sucked up into the atmosphere, rising until they mix with the clouds, anchoring this strange weather at the places the bombs hit
|
@ -1,88 +0,0 @@
|
||||
import nltk
|
||||
from nltk.tokenize import word_tokenize
|
||||
|
||||
# open two text files as text1 and text2
|
||||
with open('./rosas.txt', 'r') as result1:
|
||||
text1 = result1.read()
|
||||
|
||||
with open('./unthought.txt', 'r') as result2:
|
||||
text2 = result2.read()
|
||||
|
||||
|
||||
# HTML TOKENIZER (word + span)
|
||||
# return a list of tag from a text
|
||||
# each item in the list is transformed into a html <span> tag,
|
||||
# with the class defined by the text_class argument
|
||||
#
|
||||
# es: to_html('Lorem ipsum dolor', 'test')
|
||||
# return
|
||||
# [
|
||||
# "<span class='test'>Lorem</span>",
|
||||
# "<span class='test'>ipsum</span>",
|
||||
# "<span class='test'>dolor</span>"
|
||||
# ]
|
||||
|
||||
def to_html(text, text_class):
|
||||
text_html = []
|
||||
text_list = word_tokenize(text)
|
||||
for word in text_list:
|
||||
text_html += ['<span class="' + text_class + '">' + word + '</span>']
|
||||
return text_html
|
||||
|
||||
|
||||
# WEAVER
|
||||
# weave two texts following a pattern structured as a string of A and B
|
||||
# es: ABABAAAABBBB
|
||||
# the repetition argument specifies how many times the pattern is repeated
|
||||
# the start1 and start2 arguments specify the starting point in the texts' array
|
||||
# it returns a string
|
||||
|
||||
def weave(text1, text2, pattern, repetition, start1=0, start2=0):
|
||||
embroidery = ''
|
||||
text1_cursor = start1
|
||||
text2_cursor = start2
|
||||
repeated_pattern = pattern * repetition
|
||||
for choice in repeated_pattern:
|
||||
if choice == 'A':
|
||||
embroidery += text1[text1_cursor]
|
||||
text1_cursor += 1
|
||||
if choice == 'B':
|
||||
embroidery += text2[text2_cursor]
|
||||
text2_cursor += 1
|
||||
return embroidery
|
||||
|
||||
# DEMO
|
||||
|
||||
|
||||
text_a = to_html(text1, 'text1')
|
||||
text_b = to_html(text2, 'text2')
|
||||
text_embroidery = weave(text_a, text_b, 'AAAABBBBABABAAABBB', 16)
|
||||
|
||||
# BASIC HTML5 boilerplate
|
||||
html_boilerplate = '''
|
||||
<!DOCTYPE html >
|
||||
<html lang="en" >
|
||||
<head >
|
||||
<meta charset="UTF-8" />
|
||||
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
|
||||
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
|
||||
<title > Document </title >
|
||||
<link rel="stylesheet" href="style.css" />
|
||||
</head >
|
||||
<body >
|
||||
<div class= "text">
|
||||
|
||||
{{{contents}}}
|
||||
|
||||
</div>
|
||||
</body>
|
||||
</html>
|
||||
'''
|
||||
|
||||
|
||||
# the {{{contents}}} line will be replaced with our text_embroidery
|
||||
html_out = html_boilerplate.replace('{{{contents}}}', text_embroidery)
|
||||
|
||||
# Write the results in the index.html file
|
||||
with open('result.html', 'w') as index:
|
||||
index.write(html_out)
|
@ -1,21 +0,0 @@
|
||||
html {
|
||||
font-size: 2rem;
|
||||
}
|
||||
|
||||
.text {
|
||||
text-align: justify;
|
||||
max-width: 60ch;
|
||||
margin: 50px auto;
|
||||
}
|
||||
|
||||
span::after {
|
||||
content: " ";
|
||||
}
|
||||
|
||||
.text1 {
|
||||
color: red;
|
||||
}
|
||||
|
||||
.text2 {
|
||||
color: blue;
|
||||
}
|
@ -1 +0,0 @@
|
||||
Violence lands. Hundreds of troops break into a city. At that moment the city starts recording its pain. Bodies are torn and punctured. Inhabitants memorise the assault in stutters and fragments refracted by trauma. Before the Internet is switched off, thousands of phone cameras light up. People risk their lives to record the hell surrounding them. As they frantically call and text each other, their communication erupts into hundreds of star-shaped networks. Others throw signals into the void of social media and encrypted messaging, hoping they will be picked up by someone. Meanwhile, the environment captures traces. Unpaved ground registers the tracks of long columns of armoured vehicles. Leaves on vegetation receive the soot of their exhaust while the soil absorbs and retains the identifying chemicals released by banned ammunition. The broken concrete of shattered homes records the hammering collision of projectiles. Pillars of smoke and debris are sucked up into the atmosphere, rising until they mix with the clouds, anchoring this strange weather at the places the bombs hit
|
@ -1,88 +0,0 @@
|
||||
import nltk
|
||||
from nltk.tokenize import word_tokenize
|
||||
|
||||
# open two text files as text1 and text2
|
||||
with open('./rosas.txt', 'r') as result1:
|
||||
text1 = result1.read()
|
||||
|
||||
with open('./unthought.txt', 'r') as result2:
|
||||
text2 = result2.read()
|
||||
|
||||
|
||||
# HTML TOKENIZER (word + span)
|
||||
# return a list of tag from a text
|
||||
# each item in the list is transformed into a html <span> tag,
|
||||
# with the class defined by the text_class argument
|
||||
#
|
||||
# es: to_html('Lorem ipsum dolor', 'test')
|
||||
# return
|
||||
# [
|
||||
# "<span class='test'>Lorem</span>",
|
||||
# "<span class='test'>ipsum</span>",
|
||||
# "<span class='test'>dolor</span>"
|
||||
# ]
|
||||
|
||||
def to_html(text, text_class):
|
||||
text_html = []
|
||||
text_list = word_tokenize(text)
|
||||
for word in text_list:
|
||||
text_html += ['<span class="' + text_class + '">' + word + '</span>']
|
||||
return text_html
|
||||
|
||||
|
||||
# WEAVER
|
||||
# weave two texts following a pattern structured as a string of A and B
|
||||
# es: ABABAAAABBBB
|
||||
# the repetition argument specifies how many times the pattern is repeated
|
||||
# the start1 and start2 arguments specify the starting point in the texts' array
|
||||
# it returns a string
|
||||
|
||||
def weave(text1, text2, pattern, repetition, start1=0, start2=0):
|
||||
embroidery = ''
|
||||
text1_cursor = start1
|
||||
text2_cursor = start2
|
||||
repeated_pattern = pattern * repetition
|
||||
for choice in repeated_pattern:
|
||||
if choice == 'A':
|
||||
embroidery += text1[text1_cursor]
|
||||
text1_cursor += 1
|
||||
if choice == 'B':
|
||||
embroidery += text2[text2_cursor]
|
||||
text2_cursor += 1
|
||||
return embroidery
|
||||
|
||||
# DEMO
|
||||
|
||||
|
||||
text_a = to_html(text1, 'text1')
|
||||
text_b = to_html(text2, 'text2')
|
||||
text_embroidery = weave(text_a, text_b, 'AAAABBBBABABAAABBB', 16)
|
||||
|
||||
# BASIC HTML5 boilerplate
|
||||
html_boilerplate = '''
|
||||
<!DOCTYPE html >
|
||||
<html lang="en" >
|
||||
<head >
|
||||
<meta charset="UTF-8" />
|
||||
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
|
||||
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
|
||||
<title > Document </title >
|
||||
<link rel="stylesheet" href="style.css" />
|
||||
</head >
|
||||
<body >
|
||||
<div class= "text">
|
||||
|
||||
{{{contents}}}
|
||||
|
||||
</div>
|
||||
</body>
|
||||
</html>
|
||||
'''
|
||||
|
||||
|
||||
# the {{{contents}}} line will be replaced with our text_embroidery
|
||||
html_out = html_boilerplate.replace('{{{contents}}}', text_embroidery)
|
||||
|
||||
# Write the results in the index.html file
|
||||
with open('result.html', 'w') as index:
|
||||
index.write(html_out)
|
@ -1,87 +0,0 @@
|
||||
html,
|
||||
body {
|
||||
margin: 0;
|
||||
font-size: 1.125rem;
|
||||
line-height: 1.4;
|
||||
}
|
||||
|
||||
.title {
|
||||
text-align: center;
|
||||
margin-top: 32px;
|
||||
margin-bottom: 0;
|
||||
}
|
||||
|
||||
.meta {
|
||||
text-align: center;
|
||||
margin-bottom: 32px;
|
||||
}
|
||||
|
||||
.contents {
|
||||
max-width: 800px;
|
||||
margin: 0 auto;
|
||||
padding: 0 32px;
|
||||
}
|
||||
|
||||
.intro {
|
||||
background-color: tomato;
|
||||
border-radius: 16px;
|
||||
padding: 16px;
|
||||
}
|
||||
|
||||
h2 {
|
||||
margin-top: 0;
|
||||
margin-bottom: 16px;
|
||||
font-weight: normal;
|
||||
}
|
||||
|
||||
ul {
|
||||
margin: 0;
|
||||
padding: 0;
|
||||
list-style: none;
|
||||
}
|
||||
|
||||
li + li {
|
||||
margin-top: 1em;
|
||||
}
|
||||
|
||||
a {
|
||||
color: tomato;
|
||||
}
|
||||
|
||||
a::after {
|
||||
content: "⤴";
|
||||
}
|
||||
|
||||
.process {
|
||||
margin: 32px auto;
|
||||
}
|
||||
|
||||
pre.prettyprint {
|
||||
padding: 8px !important;
|
||||
font-size: 14px;
|
||||
white-space: pre-wrap;
|
||||
}
|
||||
|
||||
.result {
|
||||
margin: 16px auto;
|
||||
width: 100%;
|
||||
text-align: justify;
|
||||
padding: 8px;
|
||||
border: 2px dotted currentColor;
|
||||
}
|
||||
|
||||
.text1 {
|
||||
color: currentColor;
|
||||
}
|
||||
|
||||
.text2 {
|
||||
color: dodgerblue;
|
||||
}
|
||||
|
||||
.result span::after {
|
||||
content: " ";
|
||||
}
|
||||
|
||||
.demo {
|
||||
margin-top: 32px;
|
||||
}
|
|
Before Width: | Height: | Size: 19 KiB |
Before Width: | Height: | Size: 45 KiB |
Before Width: | Height: | Size: 229 KiB |
Before Width: | Height: | Size: 1.1 MiB |
Before Width: | Height: | Size: 14 KiB |
Before Width: | Height: | Size: 11 KiB |
Before Width: | Height: | Size: 83 KiB |
Before Width: | Height: | Size: 26 KiB |
Before Width: | Height: | Size: 588 KiB |
Before Width: | Height: | Size: 74 KiB |
@ -1,172 +0,0 @@
|
||||
html,
|
||||
body {
|
||||
font-size: 1.125rem;
|
||||
font-family: Arial, Helvetica, sans-serif;
|
||||
}
|
||||
|
||||
.meta {
|
||||
margin: 16px;
|
||||
}
|
||||
|
||||
.meta .back {
|
||||
display: inline-block;
|
||||
}
|
||||
|
||||
.info {
|
||||
border: 1px solid tomato;
|
||||
border-radius: 16px;
|
||||
display: none;
|
||||
margin: 16px;
|
||||
padding: 16px;
|
||||
}
|
||||
|
||||
.info.show {
|
||||
display: inline-block;
|
||||
}
|
||||
|
||||
#info-button {
|
||||
border: 1px solid tomato;
|
||||
border-radius: 16px;
|
||||
display: inline-block;
|
||||
width: 32px;
|
||||
height: 32px;
|
||||
margin: 16px;
|
||||
color: tomato;
|
||||
background-color: transparent;
|
||||
font-size: 1.125rem;
|
||||
cursor: pointer;
|
||||
transform: translate(0);
|
||||
transition: transform 0.4s ease-out;
|
||||
}
|
||||
|
||||
#info-button.active {
|
||||
background-color: tomato;
|
||||
color: white;
|
||||
}
|
||||
|
||||
input[type="file"] {
|
||||
display: none;
|
||||
}
|
||||
|
||||
.info .file-upload,
|
||||
input[type="submit"] {
|
||||
cursor: pointer;
|
||||
background-color: transparent;
|
||||
font-size: 16px;
|
||||
padding: 0;
|
||||
line-height: 1;
|
||||
border: none;
|
||||
border-bottom: 1px solid tomato;
|
||||
color: tomato;
|
||||
}
|
||||
|
||||
#categories {
|
||||
border-radius: 16px;
|
||||
display: block;
|
||||
margin: 16px;
|
||||
margin-top: 32px;
|
||||
margin-bottom: 64px;
|
||||
}
|
||||
|
||||
.tag {
|
||||
display: inline-block;
|
||||
border: 1px solid currentColor;
|
||||
padding: 0 0.5ch;
|
||||
border-radius: 1em;
|
||||
margin: 4px;
|
||||
transform: translate(0);
|
||||
transition: transform 0.4s ease-out;
|
||||
text-transform: capitalize;
|
||||
user-select: none;
|
||||
}
|
||||
|
||||
.tag:hover,
|
||||
#show-info:hover {
|
||||
transition: transform 0.1s ease-in;
|
||||
transform: translateY(-4px);
|
||||
cursor: pointer;
|
||||
}
|
||||
|
||||
.tag.active {
|
||||
background-color: tomato;
|
||||
color: white;
|
||||
}
|
||||
|
||||
.tag.all {
|
||||
background-color: transparent;
|
||||
color: currentColor;
|
||||
}
|
||||
|
||||
.all-button {
|
||||
font-size: 1.125rem;
|
||||
display: inline-block;
|
||||
background-color: transparent;
|
||||
border: 1px solid currentColor;
|
||||
padding: 0 0.5ch;
|
||||
border-radius: 1em;
|
||||
margin: 4px;
|
||||
cursor: pointer;
|
||||
}
|
||||
|
||||
.all-button.active {
|
||||
background-color: tomato;
|
||||
color: white;
|
||||
}
|
||||
|
||||
#chat-container {
|
||||
padding: 0 8px;
|
||||
margin: 0 auto;
|
||||
display: flex;
|
||||
justify-content: flex-start;
|
||||
align-items: center;
|
||||
flex-wrap: wrap;
|
||||
}
|
||||
|
||||
.baloon {
|
||||
display: none;
|
||||
position: relative;
|
||||
background-color: #fff;
|
||||
border-radius: 16px;
|
||||
padding: 16px;
|
||||
margin: 16px;
|
||||
max-width: 40ch;
|
||||
line-height: 1.4;
|
||||
}
|
||||
|
||||
.baloon.active {
|
||||
display: inline-block;
|
||||
}
|
||||
|
||||
.baloon .author {
|
||||
font-family: "Times New Roman", Times, serif;
|
||||
position: absolute;
|
||||
top: 0;
|
||||
margin: 0;
|
||||
transform: translateY(-24px);
|
||||
color: tomato;
|
||||
white-space: pre;
|
||||
}
|
||||
|
||||
.baloon .contents {
|
||||
margin: 0;
|
||||
}
|
||||
|
||||
.baloon .category {
|
||||
color: #fcc;
|
||||
}
|
||||
|
||||
.category::after {
|
||||
content: ", ";
|
||||
}
|
||||
|
||||
/* .category::before {
|
||||
content: "#";
|
||||
} */
|
||||
|
||||
.category:first-of-type::before {
|
||||
content: " ";
|
||||
}
|
||||
|
||||
.category:last-of-type::after {
|
||||
content: "";
|
||||
}
|
@ -1,36 +0,0 @@
|
||||
<!DOCTYPE html>
|
||||
<html lang="en">
|
||||
<head>
|
||||
<meta http-equiv="content-type" content="text/html; charset=UTF-8" />
|
||||
<meta charset="UTF-8" />
|
||||
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
|
||||
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
|
||||
<title>Chat a pad - WIP</title>
|
||||
<script src="./csv_to_chat.js" defer="defer"></script>
|
||||
<link rel="stylesheet" href="../global.css" />
|
||||
<link rel="stylesheet" href="./chat.css" />
|
||||
</head>
|
||||
<body>
|
||||
<div class="meta">
|
||||
<a href="." class="back">back</a>
|
||||
<button id="info-button">?</button> <br />
|
||||
<div class="info" id="info">
|
||||
You can also upload a CSV file with the following headers:
|
||||
<em>text; author; image; tag;</em>
|
||||
<form id="myForm">
|
||||
<label for="csvFile" class="file-upload">
|
||||
<input type="file" id="csvFile" accept=".csv" />
|
||||
Select File
|
||||
</label>
|
||||
<br />
|
||||
<input type="submit" value="Upload" />
|
||||
</form>
|
||||
</div>
|
||||
</div>
|
||||
|
||||
<div id="categories">
|
||||
<button class="all-button active" id="active-all">All Tags</button>
|
||||
</div>
|
||||
<div id="chat-container"></div>
|
||||
</body>
|
||||
</html>
|
@ -1,11 +0,0 @@
|
||||
reference:
|
||||
https://pad.xpub.nl/p/mix_of_sauces
|
||||
|
||||
original text
|
||||
https://pad.xpub.nl/p/SP_16_0610
|
||||
|
||||
chat archive
|
||||
https://cryptpad.fr/sheet/#/3/sheet/edit/16a0948f1c482b711c81808a853ff566/
|
||||
|
||||
in order to make Generative Baloon.vl works you need to download vvvv gamma !
|
||||
https://visualprogramming.net/
|
@ -1,96 +0,0 @@
|
||||
html,
|
||||
body {
|
||||
margin: 0;
|
||||
font-size: 1.125rem;
|
||||
line-height: 1.4;
|
||||
}
|
||||
|
||||
.title {
|
||||
text-align: center;
|
||||
margin-top: 32px;
|
||||
margin-bottom: 0;
|
||||
}
|
||||
|
||||
.meta {
|
||||
text-align: center;
|
||||
margin-bottom: 32px;
|
||||
}
|
||||
|
||||
.contents {
|
||||
max-width: 800px;
|
||||
margin: 0 auto;
|
||||
margin-bottom: 64px;
|
||||
padding: 0 32px;
|
||||
}
|
||||
|
||||
.intro {
|
||||
background-color: tomato;
|
||||
border-radius: 16px;
|
||||
padding: 16px;
|
||||
}
|
||||
|
||||
h2 {
|
||||
margin-top: 0;
|
||||
margin-bottom: 16px;
|
||||
font-weight: normal;
|
||||
}
|
||||
|
||||
ul {
|
||||
margin: 16px 0;
|
||||
padding: 0;
|
||||
list-style: none;
|
||||
}
|
||||
|
||||
li + li {
|
||||
margin-top: 0.25em;
|
||||
}
|
||||
|
||||
a {
|
||||
color: tomato;
|
||||
}
|
||||
|
||||
a::after {
|
||||
content: "⤴";
|
||||
}
|
||||
|
||||
.process {
|
||||
margin: 32px auto;
|
||||
}
|
||||
|
||||
pre.prettyprint {
|
||||
padding: 8px !important;
|
||||
font-size: 14px;
|
||||
white-space: pre-wrap;
|
||||
}
|
||||
|
||||
.result {
|
||||
margin: 32px auto;
|
||||
width: 100%;
|
||||
text-align: justify;
|
||||
}
|
||||
|
||||
.text1 {
|
||||
color: currentColor;
|
||||
}
|
||||
|
||||
.text2 {
|
||||
color: dodgerblue;
|
||||
}
|
||||
|
||||
.result span::after {
|
||||
content: " ";
|
||||
}
|
||||
|
||||
.demo {
|
||||
margin-top: 32px;
|
||||
}
|
||||
|
||||
.video-demo {
|
||||
width: 100%;
|
||||
}
|
||||
|
||||
video {
|
||||
border: 1px solid tomato;
|
||||
margin: 0 auto;
|
||||
max-width: 100%;
|
||||
}
|
Can't render this file because it has a wrong number of fields in line 20.
|
Before Width: | Height: | Size: 170 KiB |
Before Width: | Height: | Size: 264 KiB |
@ -1,215 +0,0 @@
|
||||
<!DOCTYPE html>
|
||||
<html lang="en">
|
||||
<head>
|
||||
<meta charset="UTF-8" />
|
||||
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
|
||||
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
|
||||
<title>Insecam Transcript</title>
|
||||
<link rel="stylesheet" href="../global.css" />
|
||||
<link rel="stylesheet" href="style.css" />
|
||||
</head>
|
||||
<body>
|
||||
<img src="shoes.jpg" alt="still from a shoes shop in russia" />
|
||||
<div class="episode">
|
||||
<h2>#1</h2>
|
||||
<div class="container">
|
||||
<p>
|
||||
The smell of new cheap shoes <br />
|
||||
The sound of russian in the background <br />
|
||||
People leaving untidy items around holding other shops' bags while checking shoes
|
||||
with the other hand <br />
|
||||
wearing too many clothes but outside it's cold, not worth it to take off the
|
||||
jacket<br />
|
||||
and hold it an employee shows a customer some high leather boots assortment of
|
||||
waterproof padded jackets in different colors<br />
|
||||
long&just washed high quality russian girls' hair
|
||||
</p>
|
||||
<p>
|
||||
Red head woman, indecisive <br />
|
||||
Sales woman, nonchalantly wearing her face-mask right below nose <br />
|
||||
There is 50% sales on a selection of items<br />
|
||||
Small girl shopping for a new pair of shoes, school is starting in a few days<br />
|
||||
Young woman just entered, she is not planning to buy anything<br />
|
||||
But she seems like she will, easy target <br />
|
||||
Her friend is exhausted, she will wait sitted on the bench till she takes a
|
||||
decision<br />
|
||||
Red head is still in the store <br />
|
||||
Girl sitted on the bench is only checking pairs within her arm reach <br />
|
||||
Possible target audience of the shop: small girls, young girls, older women <br />
|
||||
Girl sitted on the bench received a text message
|
||||
</p>
|
||||
<p>
|
||||
The pattern on the floor tiles sets the stage for new sales offer: <br />
|
||||
-50% on some shiny new boots. <br />
|
||||
Yet kids are not intrested: <br/>
|
||||
they prefer to bounce from red tile to red tile <br/>
|
||||
in order to spin the speed of shoes shopping. <br />
|
||||
A woman is not shure of buy a pair of shoes. <br />
|
||||
She focuses on the materials and the quality of construction. <br />
|
||||
She weighs the price and how renowed the firm is. <br />
|
||||
Then she puts the pair back on the shelf. <br />
|
||||
An old lady picks it up again. <br />
|
||||
She feels observed. <br />
|
||||
She feels not confident about her opinions about these shoes. <br />
|
||||
She peeps other people choices, considering other pairs of shoes. <br />
|
||||
She strolls through the shop. <br />
|
||||
Then returns to the initial ones.
|
||||
</p>
|
||||
</div>
|
||||
</div>
|
||||
<img src="barber.jpg" alt="still from a barber shop in france" />
|
||||
<div class="episode">
|
||||
<h2>#2</h2>
|
||||
<div class="container">
|
||||
<p>
|
||||
Noisy space <br />
|
||||
Blow dryer is on <br />
|
||||
Face-mask seem required but the heat under is unbearable <br />
|
||||
A man doesn't seem to have much to do <br />
|
||||
He is chatty <br />
|
||||
Chatting to a girl sitting <br />
|
||||
He doesn't sit, I find it pretty annoying <br />
|
||||
Just sit if you are talking for so long!!! <br />
|
||||
The man crosses and un-crosses his legs, seem nervous <br />
|
||||
Is he nervous because of the sitted girl? <br />
|
||||
I would say so <br />
|
||||
WHY DON'T YOU SIT <br />
|
||||
I can't see the sitted girl's face at all <br />
|
||||
A standing man, turning his back to the camera is talking to a sitted pair of leg
|
||||
<br />
|
||||
I bet the hairdresser is also bothered by him <br />
|
||||
She is eying his reflection in the mirror <br />
|
||||
Her crossed legs on the armchair has not un-crossed, shows how tensed he makes her
|
||||
feel <br />
|
||||
Dude just sit!
|
||||
</p>
|
||||
<p>
|
||||
The smell of shampoo and cleanness <br />
|
||||
The mysterious crossed legs from a girl which we are not able to see more<br />
|
||||
Is the guy standing next to her bending over to kiss her? <br />
|
||||
The deafening sound of the hairdryer <br />
|
||||
The feel of hot air on your neck<br />
|
||||
THe reflection of the hairdresser in the mirror behind<br />
|
||||
The feel of expectation - how am I gonna look? - for sure it was worth the money.<br />
|
||||
The cold, slippery touch of these fake leather seats.<br />
|
||||
The standarization of clothing items such as the square pattern shirt, the denim
|
||||
jeans he wears or the black sneakers and black leggins she wears<br />
|
||||
AH! new customer, new hairdresser in frame.<br />
|
||||
He sits, she starts washing his hair.<br />
|
||||
I wonder when was the last time I went to the hairdresser.<br />
|
||||
Should I get a haircut myself
|
||||
</p>
|
||||
<p>
|
||||
Try to focus on the floor tiles, once again. <br />
|
||||
And think to the worker that has put them in place. <br />
|
||||
Hey, you did a great job under the seats in front of the mirrors, why you messed up
|
||||
everything on the other side of the room? <br />
|
||||
Now there is a guy standing on this feng-shui-wraking-four-tiles-inlays, trying to pickup a girl. <br />
|
||||
She's hidden from our point of view and we just see a pair of legs. <br />
|
||||
You can see how the barber is listening to their conversation, <br />
|
||||
Hidden among the noise of a red blowdrier. <br />
|
||||
She seems to focus on the blond hairs she's drying but that's just a cover. <br />
|
||||
The guy is insicure. <br />
|
||||
He relies more on the infrastructure of the barbershop than on his own body. <br />
|
||||
He tries to seem cool and steady, and then two other people enter the barbershop.<br />
|
||||
Or better: they were outside our field of view. <br />
|
||||
One is a barber, the other one a hair owner.<br />
|
||||
</p>
|
||||
</div>
|
||||
</div>
|
||||
<img src="cows.jpg" alt="still from a cow stable" />
|
||||
<div class="episode">
|
||||
<h2>#3</h2>
|
||||
<div class="container">
|
||||
<p>
|
||||
One cow seem to be full of milk<br />
|
||||
Her milk sack just lies on the hay like a full bag of marbles<br />
|
||||
She got up, wonder how<br />
|
||||
Are they slow or is the camera lagging?<br />
|
||||
Nodding left and right<br />
|
||||
They seem lost in a space that yet hosts them since months<br />
|
||||
Common just cheer up<br />
|
||||
A younger cow is approaching<br />
|
||||
She has more white than brown, makes her look even younger, as if her pattern wasn't
|
||||
yet finished<br />
|
||||
How would it feel to be inside a cow stomach?<br />
|
||||
Warm, dark, and silent<br />
|
||||
Agitation in the stable!<br />
|
||||
One seem especially lazy<br />
|
||||
Hay is wet<br />
|
||||
A farmer passed by<br />
|
||||
The lazy one got up<br />
|
||||
I feel your pressure sister<br />
|
||||
Like when a manager comes and checks you at work
|
||||
</p>
|
||||
<p>
|
||||
Unknown number of cows in an unknown-sized farm<br />
|
||||
Two cows in frame, three bits of cows coming out of the frame.<br />
|
||||
The realisation that whatever you might be doing or busy with, these cows are
|
||||
there.<br />
|
||||
The realisation that whatever you might be busy with is somehow more fun than being
|
||||
a cow?<br />
|
||||
|
||||
The cow has some hay on top. Who put it there? How could it fall there? did it get
|
||||
stuck somehow <br />
|
||||
|
||||
Two new pattern cows come in the frame.<br />
|
||||
New gang? did they know each other already? Do they care about being brown or
|
||||
patterned?<br />
|
||||
|
||||
What is out of the frame? Wondering about that and the sound we cannot hear.<br />
|
||||
Is it cold? well probably, its in Augsburg.<br />
|
||||
|
||||
The cows melt in the background, such a rich brown palette. <br />
|
||||
EH Human in frame. quickly, crossed the frame in diagonal and left through the upper
|
||||
right corner.
|
||||
</p>
|
||||
<p>
|
||||
How do you define a group of cows? <br />
|
||||
A flock of cows?<br />
|
||||
A storm of cows?<br />
|
||||
A crowd of cows?<br />
|
||||
A family of cows?<br />
|
||||
A team of cows?<br />
|
||||
A herd of cows?<br />
|
||||
A drove of cows?<br />
|
||||
|
||||
I will just call them by their name.<br />
|
||||
From this aerial point of view you can recognize flora, in the right bottom corner
|
||||
of the view<br />
|
||||
Luca, relaxed in the left corner, with a concern on her rear right leg,<br />
|
||||
Notalie enter the stage, followed by Sofia. <br />
|
||||
Natalie is mooing about the haircut the farmer did to her.<br />
|
||||
Her black&white pattern should have been taken more in consideration during the grooming.<br />
|
||||
Sofia agrees, but then turns away from Natalie.<br />
|
||||
(I see some not-so-hidden envy here)<br />
|
||||
It happens when you keep so many gracious gracing animals in a such small place.
|
||||
</p>
|
||||
</div>
|
||||
</div>
|
||||
|
||||
<div class="episode outro">
|
||||
<h1 class="title">Cam Transcript</h1>
|
||||
<div class="meta">
|
||||
Kimberley + Carmen + Kamo <br />
|
||||
<a href="https://pad.xpub.nl/p/SP16_1210"> SI16 - with Cristina and Manetta</a>
|
||||
<a href=".." class="back">back</a>
|
||||
</div>
|
||||
|
||||
<div class="intro">
|
||||
<h2> Video Transcribing</h2>
|
||||
<ul>
|
||||
<li>In groups of 2-3:</li>
|
||||
<li>1. Decide on a video to transcribe (max 10 min)</li>
|
||||
<li>
|
||||
2. If you can't decide on one, take 3-5 minutes to think about a subject of everyday
|
||||
knowledge that is particular to a location/group. Record yourself telling the story
|
||||
</li>
|
||||
<li>3. Transcribe individually either the video or your own recording</li>
|
||||
<li>4. Compare the transcriptions</li>
|
||||
</ul>
|
||||
</div>
|
||||
</div>
|
||||
|
||||
</body>
|
||||
</html>
|
Before Width: | Height: | Size: 240 KiB |
@ -1,65 +0,0 @@
|
||||
const table = document.getElementById("table");
|
||||
|
||||
fetch("./cms.json")
|
||||
.then((response) => {
|
||||
return response.json();
|
||||
})
|
||||
.then((data) => populateContents(data));
|
||||
|
||||
function populateContents(data) {
|
||||
data.projects.reverse().forEach((project) => addRow(project));
|
||||
}
|
||||
|
||||
function addRow(project) {
|
||||
let row = document.createElement("tr");
|
||||
|
||||
let title = document.createElement("td");
|
||||
title.classList.add("title");
|
||||
row.appendChild(title);
|
||||
|
||||
let titleUrl = document.createElement("a");
|
||||
titleUrl.href = project.url;
|
||||
titleUrl.innerHTML = project.title;
|
||||
title.appendChild(titleUrl);
|
||||
|
||||
let links = document.createElement("td");
|
||||
links.classList.add("links");
|
||||
if (project.pad) {
|
||||
let padLink = document.createElement("a");
|
||||
padLink.href = project.pad;
|
||||
padLink.innerHTML = "pad";
|
||||
links.appendChild(padLink);
|
||||
}
|
||||
if (project.git) {
|
||||
let gitLink = document.createElement("a");
|
||||
gitLink.href = project.git;
|
||||
gitLink.innerHTML = "git";
|
||||
links.appendChild(gitLink);
|
||||
}
|
||||
if (project.links.length) {
|
||||
project.links.forEach((url) => {
|
||||
let link = document.createElement("a");
|
||||
link.href = url.url;
|
||||
link.innerHTML = url.title;
|
||||
links.appendChild(link);
|
||||
});
|
||||
}
|
||||
row.appendChild(links);
|
||||
|
||||
let categories = document.createElement("td");
|
||||
categories.classList.add("categories");
|
||||
project.categories.forEach((tag) => {
|
||||
let category = document.createElement("span");
|
||||
category.classList.add("tag");
|
||||
category.innerHTML = tag;
|
||||
categories.appendChild(category);
|
||||
});
|
||||
row.appendChild(categories);
|
||||
|
||||
let date = document.createElement("td");
|
||||
date.classList.add("date");
|
||||
date.innerHTML = project.date;
|
||||
row.appendChild(date);
|
||||
|
||||
table.appendChild(row);
|
||||
}
|
@ -1,102 +0,0 @@
|
||||
{
|
||||
"projects": [
|
||||
{
|
||||
"title": "Text Lifeboats",
|
||||
"date": "Sep 28, 2021",
|
||||
"url": "09-28-2021-lifeboats/",
|
||||
"git": "",
|
||||
"pad": "https://pad.xpub.nl/p/collab_week3",
|
||||
"links": [],
|
||||
"categories": [
|
||||
"JS",
|
||||
"Text"
|
||||
]
|
||||
},
|
||||
{
|
||||
"title": "Text Weaving",
|
||||
"date": "Oct 5, 2021",
|
||||
"url": "10-05-2021-weaving/",
|
||||
"git": "https://git.xpub.nl/kamo/text_weaving",
|
||||
"pad": "https://pad.xpub.nl/p/replacing_cats",
|
||||
"links": [],
|
||||
"categories": [
|
||||
"Python",
|
||||
"NLTK",
|
||||
"Text"
|
||||
]
|
||||
},
|
||||
{
|
||||
"title": "Chat Reader",
|
||||
"date": "Oct 6, 2021",
|
||||
"url": "10-06-2021-chat_reader/",
|
||||
"git": "https://git.xpub.nl/kamo/chat-reader",
|
||||
"pad": "https://pad.xpub.nl/p/SP16_0510",
|
||||
"links": [],
|
||||
"categories": [
|
||||
"JS",
|
||||
"Chat",
|
||||
"Text"
|
||||
]
|
||||
},
|
||||
{
|
||||
"title": "Cam Transcript",
|
||||
"date": "Oct 12, 2021",
|
||||
"url": "10-12-2021-cam-transcript/",
|
||||
"git": "",
|
||||
"pad": "https://pad.xpub.nl/p/cam-stranscript",
|
||||
"links": [],
|
||||
"categories": [
|
||||
"Text",
|
||||
"Video"
|
||||
]
|
||||
},
|
||||
{
|
||||
"title": "Rejection 🧠⛈️",
|
||||
"date": "Oct 19, 2021",
|
||||
"url": "../rejection/",
|
||||
"git": "",
|
||||
"pad": "https://pad.xpub.nl/p/GroupMeeting_18102021",
|
||||
"links": [
|
||||
{
|
||||
"url": "https://pad.xpub.nl/p/Rejection_Glossary",
|
||||
"title": "Glossary"
|
||||
},
|
||||
{
|
||||
"url": "https://pzwiki.wdka.nl/mediadesign/Rejection",
|
||||
"title": "Wiki"
|
||||
}
|
||||
],
|
||||
"categories": [
|
||||
"SI16",
|
||||
"Process"
|
||||
]
|
||||
},
|
||||
{
|
||||
"title": "🥣 Soup-gen",
|
||||
"date": "Oct 19, 2021",
|
||||
"url": "../soup-gen/",
|
||||
"git": "https://git.xpub.nl/grgr/soup-gen",
|
||||
"pad": "",
|
||||
"links": [],
|
||||
"categories": [
|
||||
"Web",
|
||||
"CMS",
|
||||
"Long Term"
|
||||
]
|
||||
},
|
||||
{
|
||||
"title": "🎵 K-PUB",
|
||||
"date": "Oct 21, 2021",
|
||||
"url": "../k-pub/",
|
||||
"git": "https://git.xpub.nl/grgr/k-pub",
|
||||
"pad": "",
|
||||
"links": [],
|
||||
"categories": [
|
||||
"Event",
|
||||
"vvvv",
|
||||
"Text",
|
||||
"Long Term"
|
||||
]
|
||||
}
|
||||
]
|
||||
}
|
@ -0,0 +1,70 @@
|
||||
---
|
||||
title: appunti x brera
|
||||
description: proto slide
|
||||
css: brera.css
|
||||
---
|
||||
|
||||
## Ricerca
|
||||
|
||||
Ciao io mi chiamo FRANCESCO e la mia passione è sviluppare _software site specific_, cioè: che rispondono alla forma delle comunità in cui emergono. In questo modo è possibile generare relazioni e strumenti slegati dalle specifiche imposte dall'industria.
|
||||
|
||||
Il mio background è: vario (musica, video, animazione, graphic design, web design, programmazione, danza, ) e questo è il motivo per cui il mio portfolio è: altrettanto vario.
|
||||
|
||||
Ciò che accomuna i lavori è un approccio infrastrutturale: ogni progetto è anche il modo per avviare un discorso sul tipo di strumenti e interfacce che quella particolare situazione richiede o offre.
|
||||
|
||||
A un certo punto questo metodo diventa anche una riflessione sull'ecologia del software: come scegliamo i nostri strumenti? Cosa implica usare un'applicazione, un linguaggio, una tecnologia rispetto ad un'altra? Che relazioni si creano tra sviluppatore e utente, tra artista e pubblico? Ecc.
|
||||
|
||||
## Pratica
|
||||
|
||||
Strategie per affrontare la complessità contemporanea:
|
||||
|
||||
### rappresentare → intuizione e sintesi
|
||||
- interfacce per accedere alla complessità,
|
||||
- forme intuitive contro forme razionali,
|
||||
- sintesi contro semplificazione,
|
||||
|
||||
### documentare → narrazione situata
|
||||
- distanza critica tra il mondo e i mezzi usati per documentarlo.
|
||||
- un approccio che slega il reale dal fotorealistico (decolonizzare l'immagine?)
|
||||
|
||||
### abitare → esplorazione epistemologica
|
||||
- esplorare una condizione dall'interno per rinegoziarne i limiti e il possibile
|
||||
- accettare la complicità coi problemi e farne un punto di forza
|
||||
|
||||
## Lavori
|
||||
|
||||
|
||||
|
||||
### Object Oriented Choreography
|
||||
|
||||
|
||||
È una performance collaborativa: danzatrice nel VR interagisce con il pubblico.
|
||||
|
||||
Concept: Affrontare l'informazione digitale attraverso diversi tipi di intelligenza: corpo e parola. Costruire un modello in scala delle piattaforme digitali contemporanee e usarlo come dispositivo drammaturgico.
|
||||
|
||||

|
||||

|
||||
|
||||
Sviluppo: tesi, Triennale, RomaEuropa, futuro
|
||||
|
||||
Materiali: foto, video, UI, schemi, testo
|
||||
|
||||
### Un \* Salta
|
||||
|
||||
- Ricerca per un internet locale
|
||||
- Progetto e Viaggio in senegal
|
||||
- Pubblicazione e riflessioni
|
||||
|
||||
### Frana Futura
|
||||
|
||||
Ricerche per un documentario 3D che mette in relazione la condizione idrogeologica ligure e le realtà che la abitano.
|
||||
|
||||

|
||||
|
||||

|
||||
|
||||
### XPUB
|
||||
|
||||
- Relazione tra sistemi complessi e ambienti in cui vengono sviluppati
|
||||
- Temi
|
||||
- metodi
|
@ -0,0 +1,14 @@
|
||||
---
|
||||
title: Documentation workout
|
||||
description: hello this is a log of francesco
|
||||
---
|
||||
|
||||
Hello these are some logs from kamo. I like to develop software to inhabit complexity with visual and performative outcomes. You can find here a lot of works in progress and some finished projects. My resolution for these 2 years is to get good in documenting things I do.
|
||||
So this is basically workout.
|
||||
|
||||
Ciao
|
||||
|
||||
## TO DO##
|
||||
|
||||
- Dark mood
|
||||
- Fix chat reader
|
@ -0,0 +1,105 @@
|
||||
---
|
||||
title: Transcript of my assessment from the future
|
||||
css: recap.css
|
||||
cover: recap/cover.jpg
|
||||
cover_alt: dinosaurs
|
||||
---
|
||||
|
||||
## Hello
|
||||
|
||||
This months in XPUB were a lot of fun. At the beginning I was terrorized because of the moving, new people, new places, different language, so probably to cope with the stress I had an initial outburst of energies. Now I'm trying to stay calm.
|
||||
|
||||
## Purpose
|
||||
|
||||
Lately I've been interested in programming. Probably because it's a playful way to interact with reality starting from abstraction. Something like theory but with instant and tangible outputs. Something like incantation or psychokinesis.
|
||||
|
||||
I'm into the development of site-specific software. Codes that inhabit and interact with a community. Coding as a form of care. Programming as a way to facilitate _agency-on_ and _comprehension-of_ complex systems. I'm learning how to approach complexity as an environment. How a work can be complex without forcing the result being complicated.
|
||||
|
||||
## Methods
|
||||
|
||||
I like to propose ideas to the group. Sometimes the idea [does not deliver](/soupboat/~kamo/projects/loot-box-sealing-device/) , sometimes it lands but the results are [catastrophic](/soupboat/~kamo/projects/si16-structure-proposal/) , sometimes the outcomes are [unexpected](/soupboat/~kamo/projects/annotation-compass/) , and sometimes they [unexpectedly work](/soupboat/padliography/) .
|
||||
|
||||
My main interest is the ecology around these ideas. How it can be enriched and transformed by different voices, and the kind of play and space that it offers. I'm trying to shift from _developing crazy things_ to _developing meaningful things_. Meaningful especially in relation to the environment and the other people involved in the process. To be meaningful an idea should stands on its own, but functioning as a starting point, more than a finite result.
|
||||
|
||||
I get easily _bored-of_ or _carried-away-by_ my train of thoughts, so I need to rely on others. That's why I always collaborate with someone else and rarely do things alone.
|
||||
|
||||
|
||||
## Outputs
|
||||
|
||||
### Warm up
|
||||
|
||||
During the first month every day was a fest and we developed everything that came to our mind. To have the Soupboat felt empowering. A place to call home in the internet.   Not really a critical approach maybe, but an ecstatic condition.
|
||||
|
||||
Together with Erica we tried to develop a [shared cookbook](/soupboat/soup-gen/)  , then a [birthday cards collector](/soupboat/b-say/)  to spawn best wishes in the Soupboat homepage at each birthday, some prototypes for the [karaoke as a form of republishing](/soupboat/k-pub/) , a way to track all [our pads](/soupboat/padliography/)  through the media wiki API.
|
||||
|
||||
During the group exercise I collaborated to the [text lifeboats](/soupboat/~kamo/static/html/lifeboats/)  and different ways to [weave](/soupboat/~kamo/static/html/weaving/) texts together  to generate new meanings, transforming a complex writings into an [abstract chat](/soupboat/~kamo/static/html/chat-reader/chat.html) , and the [insecam livestream transcription](/soupboat/~kamo/static/html/cam-transcript/)   .
|
||||
|
||||
### SI16
|
||||
|
||||
I like what we did for the SI16. The concept of using an API as a form of pubblishing was [mindblowing](/soupboat/~kamo/projects/api-worldbuilding/). The approach proposed during the prototyping sessions was extremely stimulating, even if sometimes frightening to [rewind](/soupboat/~kamo/projects/si16-API-express-prototype/) and [rewind](/soupboat/~kamo/projects/si16-API-strapi-nuxt-prototype/) and [redo](/soupboat/~kamo/projects/si16-backend/) things differently . I feel sorry if at some point I pushed for something that someone perceived as a failure. I get that it's a matter of perspective: from my point of view what's important is the process and the ways we work together, while for someone else a concrete outcome is more important. I need to keep in mind that both sides are legit and valid.
|
||||
|
||||
I contributed on the overall [structure](/soupboat/~kamo/projects/si16-structure-proposal/), the [backend](/soupboat/~kamo/projects/si16-backend/) and the [frontend](/soupboat/~kamo/projects/si16-frontend-design/) , and the [visual identity](https://www.are.na/si16-visual-identity). The [Concrete Label](https://pad.xpub.nl/p/AGAINST_FILTERING) project started with Supi to annotate concrete poetry to create vernacular corpora  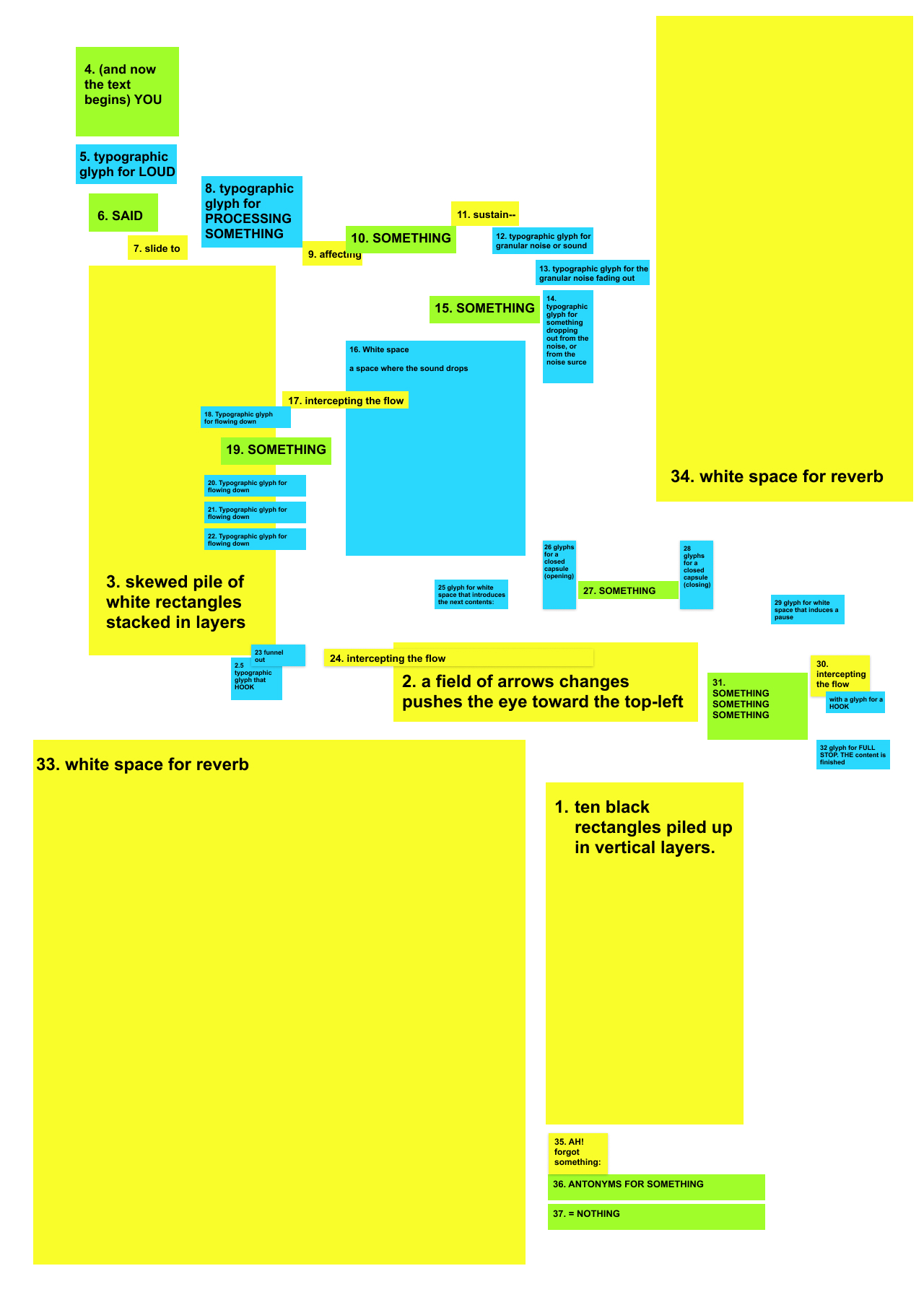 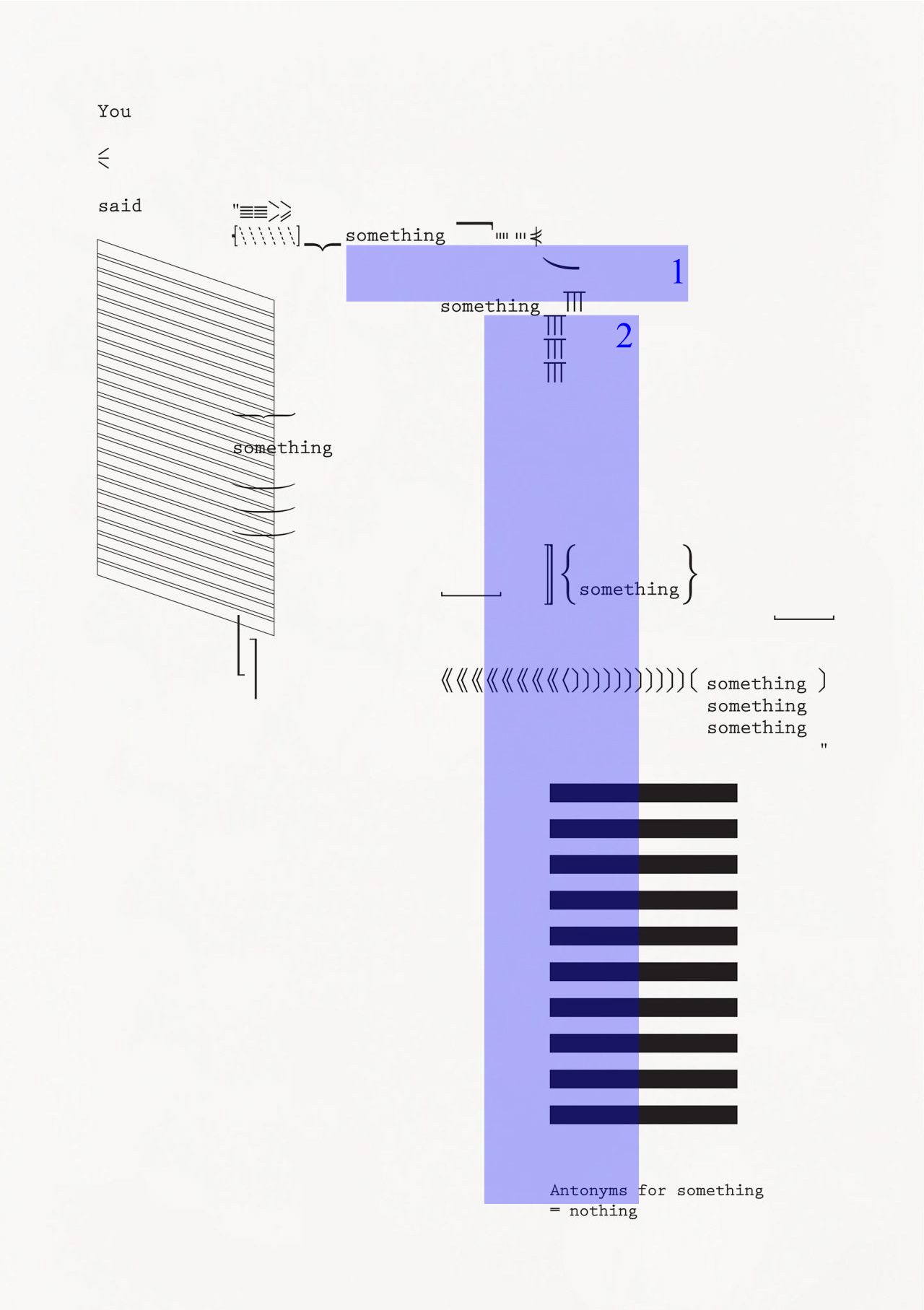 became the [Annotation Compass](/soupboat/~kamo/projects/annotation-compass/) , a tool to annotate images collectively.
|
||||
|
||||
We managed to achieve a lot: a distribuited API, a familiar CMS based on Jupiter Notebooks , a coherent environment with room for [customization](/soupboat/si16/projects/annotation-compass/rejection_map/), and a shared understanding of a complex topic . I tried to document these processes both in the soupboat and directly in the code . This required a lot of effort since I've always had a bad relation with documentation, probably because I'm an over-analitical-critical-shy person.
|
||||
|
||||
We failed in finishing things. The event-mode of the website as well as the API key circulation remain a draft. The workload was crazy, especially considered the technical difficulty of the overall project that led to unbalanced shares of work.
|
||||
|
||||
|
||||
### Slow down
|
||||
|
||||
After the winter break we slowed down, and spent more time studying the special issue's materials. Together with Erica, Mitsa, Chae, and Alex we read through all the text together. The ideology excercise with [noise canceling headphones](https://pad.xpub.nl/p/noice_cancelling_devices_turns_off_also_your_inner), the [critical karaoke](https://hub.xpub.nl/soupboat/postit/generate/karaoke) about gamification 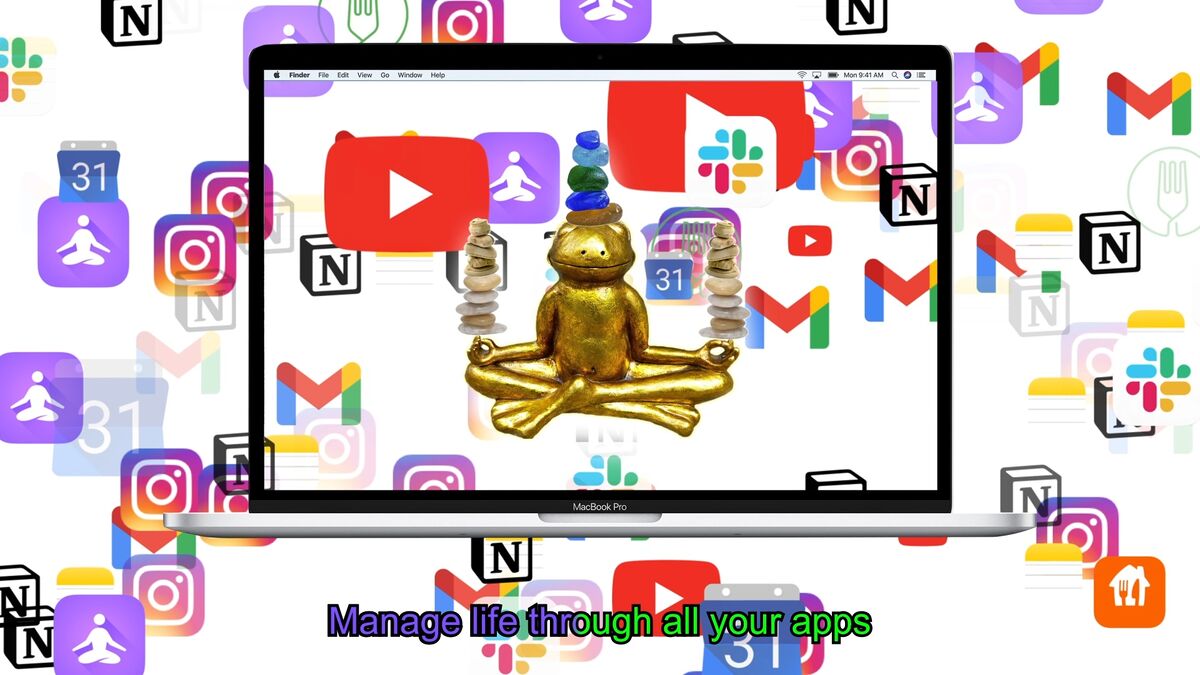, the katamari fanfiction , the [replace('mimic','loot box')](https://hub.xpub.nl/soupboat/postit/generate/mimic) research 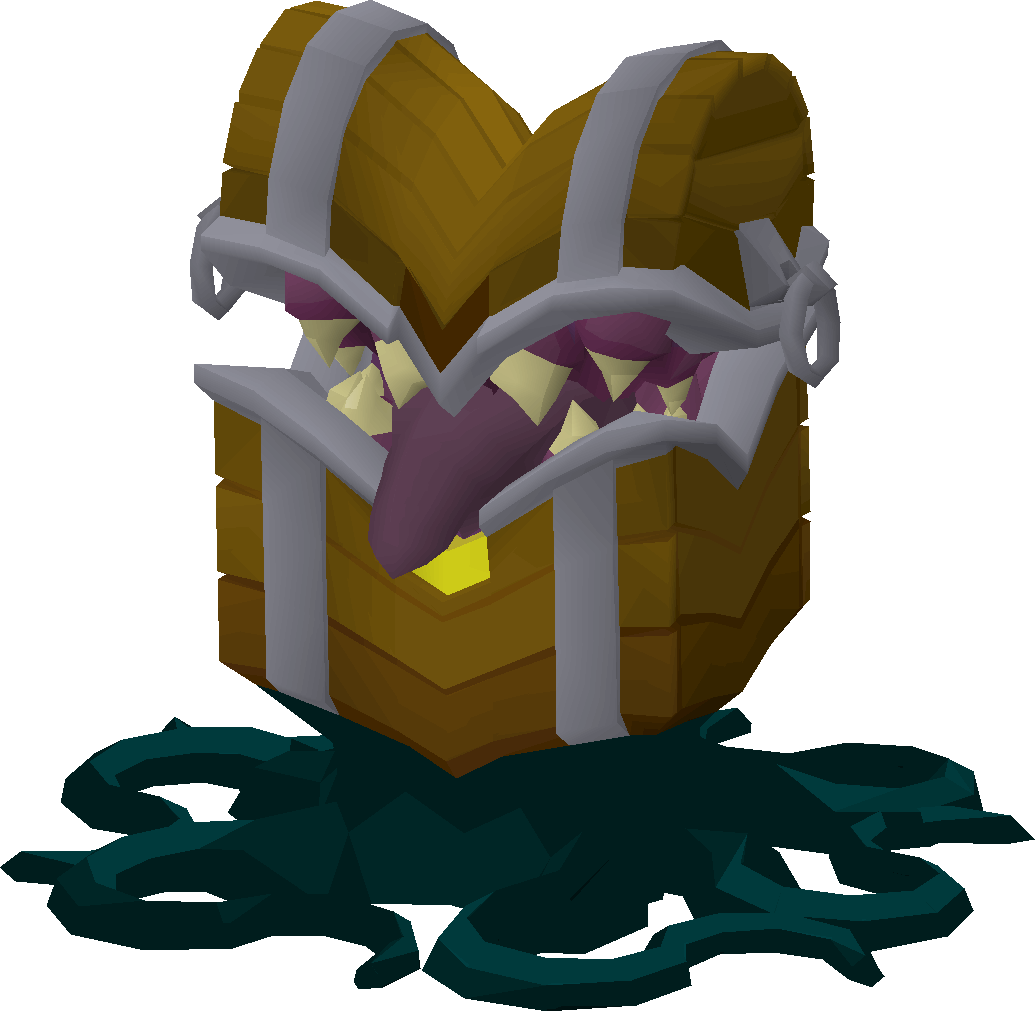, and the gravitational approach to mapping the theme of gamification 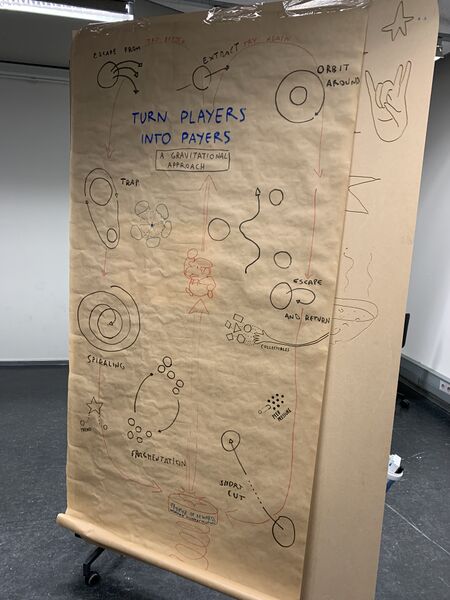 were all results of a moment with more critical focus on the contents and less on technical experiments.
|
||||
|
||||
At the beginning of the accademic year we applied to Room for Sound with the k-pub karaoke project, but timing and covid messed up with our plans, and in february after a couple of day of residency we decided to step back in order to focus more on less things.  Room for Sound understood our needs and proposed us to retry later on this year.
|
||||
|
||||
The plan now is to continue working on the karaoke format as a moment in which different forms of public meet each others: 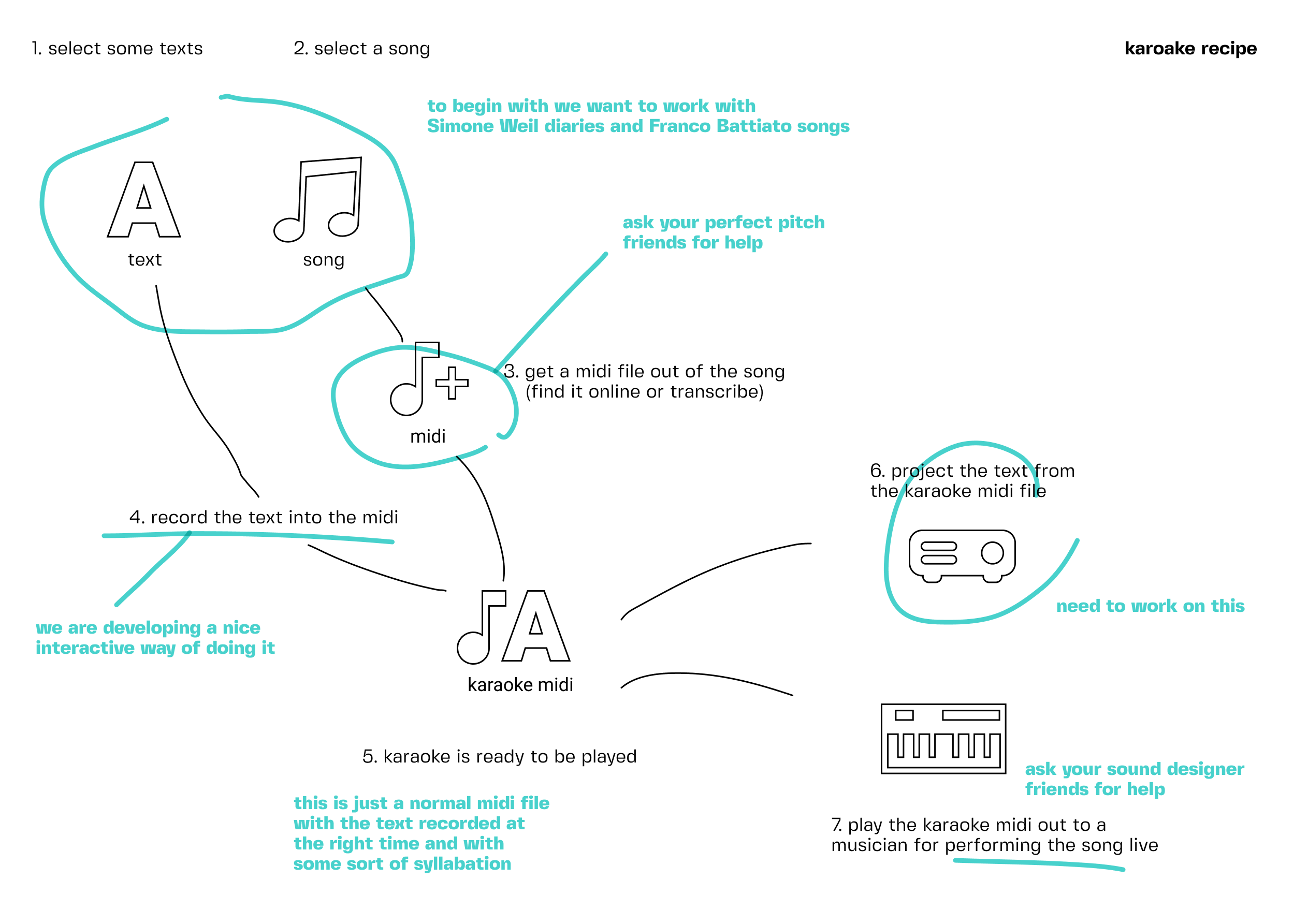 there is a text and writing aspect, a musical and sonic one, it's a collective and personal performative moment, and it's something already present in the collective imaginary. So it could be a good generative device.
|
||||
|
||||
### SI17
|
||||
|
||||
The process of SI17 was much more mindful than the previous one. As a group we put a lot of effort into the facilitation and organization of the work. The tired but cheering way we arrived at the launch is a sign of success.
|
||||
|
||||
The iterations of the work and the ideas were a lot, and the time passed on the readings was really effective to generate thoughts about the [relations within the public](https://hub.xpub.nl/soupboat/~kamo/projects/loot-box-multi-player/), the [jigsaw puzzle as a form of encryption ](https://hub.xpub.nl/soupboat/~kamo/projects/chaotic-evil-puzzles/) of our contents, the loot box as a [decorator or skin](https://hub.xpub.nl/soupboat/~kamo/projects/loot-box-decorator/) for other pubblications, [ways to seal](https://hub.xpub.nl/soupboat/~kamo/projects/loot-box-sealing-device/) 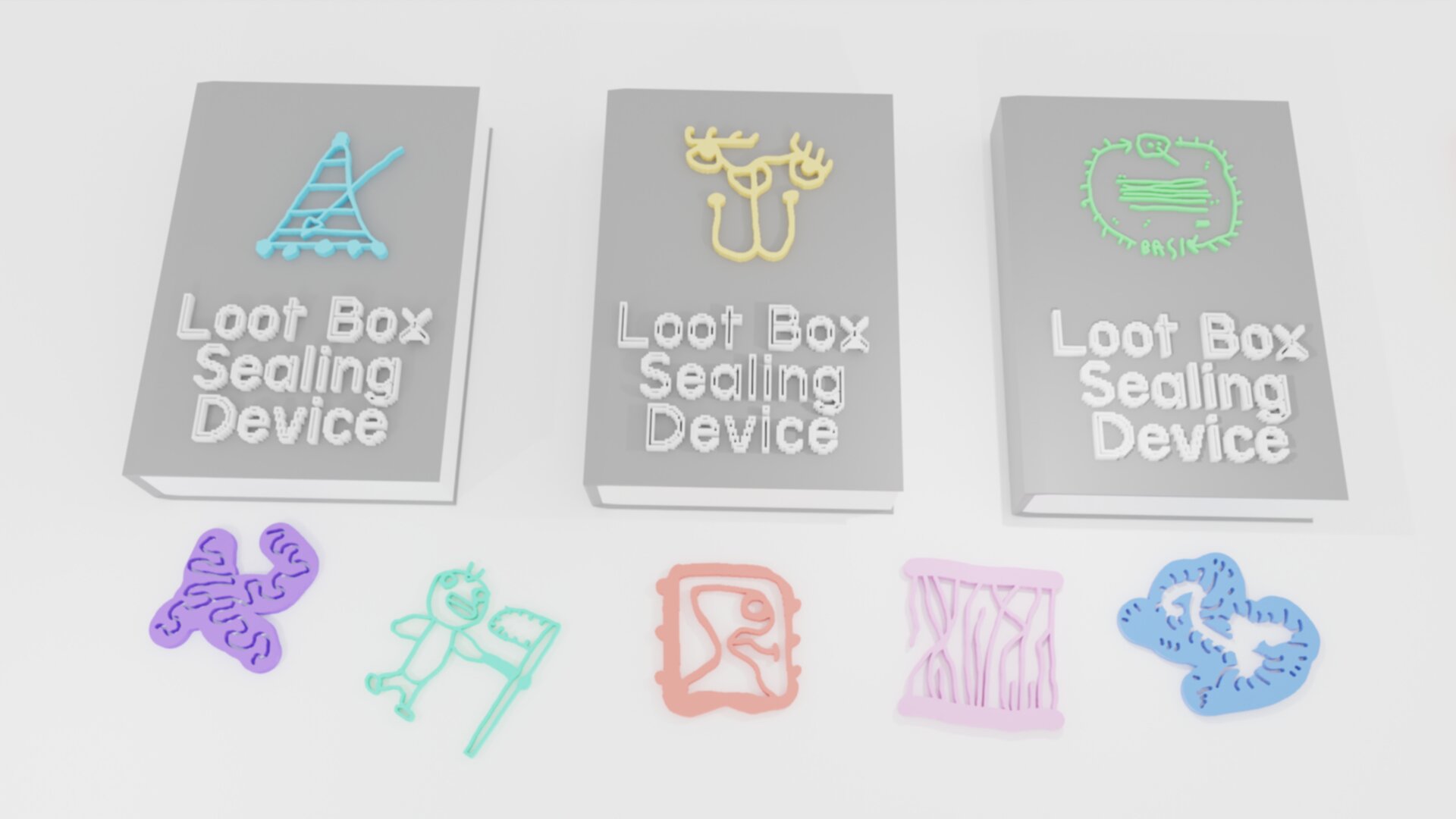 the boot lox instead of opening it, or ways to hack its inner [temporality](https://hub.xpub.nl/soupboat/~kamo/projects/loot-box-temporality/) .
|
||||
|
||||
When we entered the production phase I worked with Mitsa, Supi and Erica as part of the team 1, in charge of the contents of the boot lox. We approached the different contents with the idea of a common ground such as the [post-it](https://pad.xpub.nl/p/post-it).  We worked with a surface to [gather the contents](https://git.xpub.nl/kamo/post-it-contents)  and with another one to [generate the results](https://git.xpub.nl/kamo/postit)  . When Supi said that the way we worked made her rethink inDesign I was happy. Even if the perceived workload at some point was insane (tell me more about the blurred line between leisure and labor), the overall experience was super great, and we managed to work well together with a common pace.
|
||||
|
||||
Things went wrong only in the last days before sending the print, when we didn't manage to share the work in a fair way. To me this was a great loss since it was the main stake of the entire process. We had several reflections about this and then managed to recover the group morale working together on the website.
|
||||
|
||||
To keep things simple is difficult, but important. At the end I think the issue website  is great, since we did it altogether. It's not a problem if it's not technically interesting or flexible or modular or what else. I accepted that there are other parameters to create value and meaning. With the help of the group I also managed to give value to things I did such as the xquisite branch   and the mimic-loot box research , that ultimately ended as contents in the final pubblication.
|
||||
|
||||
Some moments were super hard: a certain Monday during spring break we should have decided on something to move on, but every proposal was rejected. It was really difficult, especially since there were valid ideas on the table. 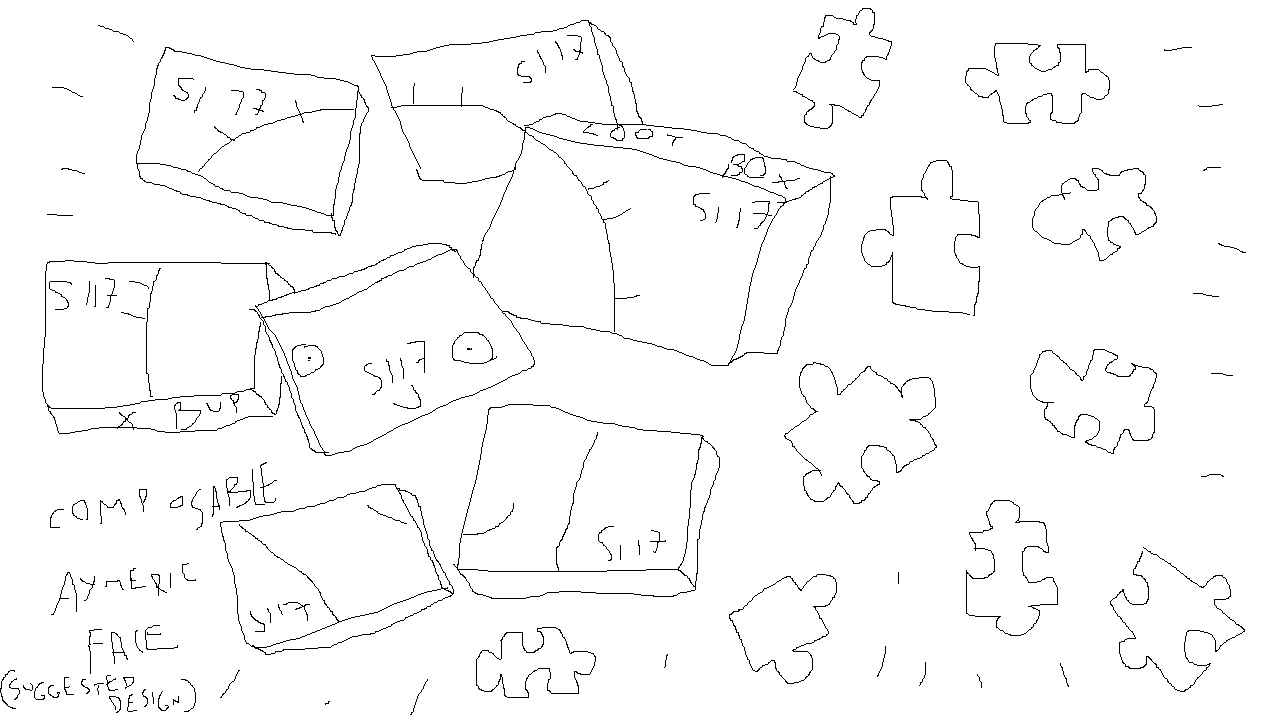  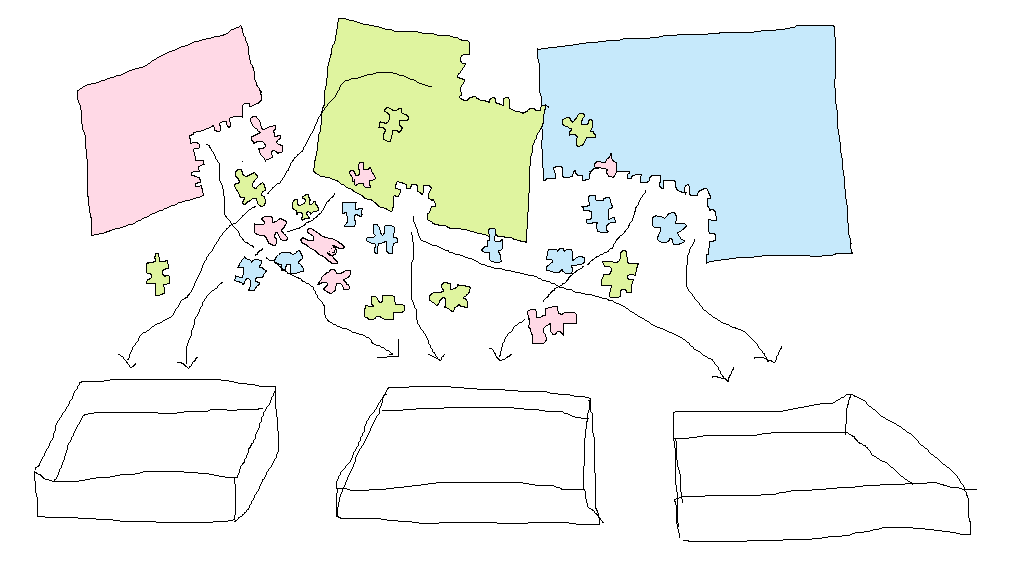 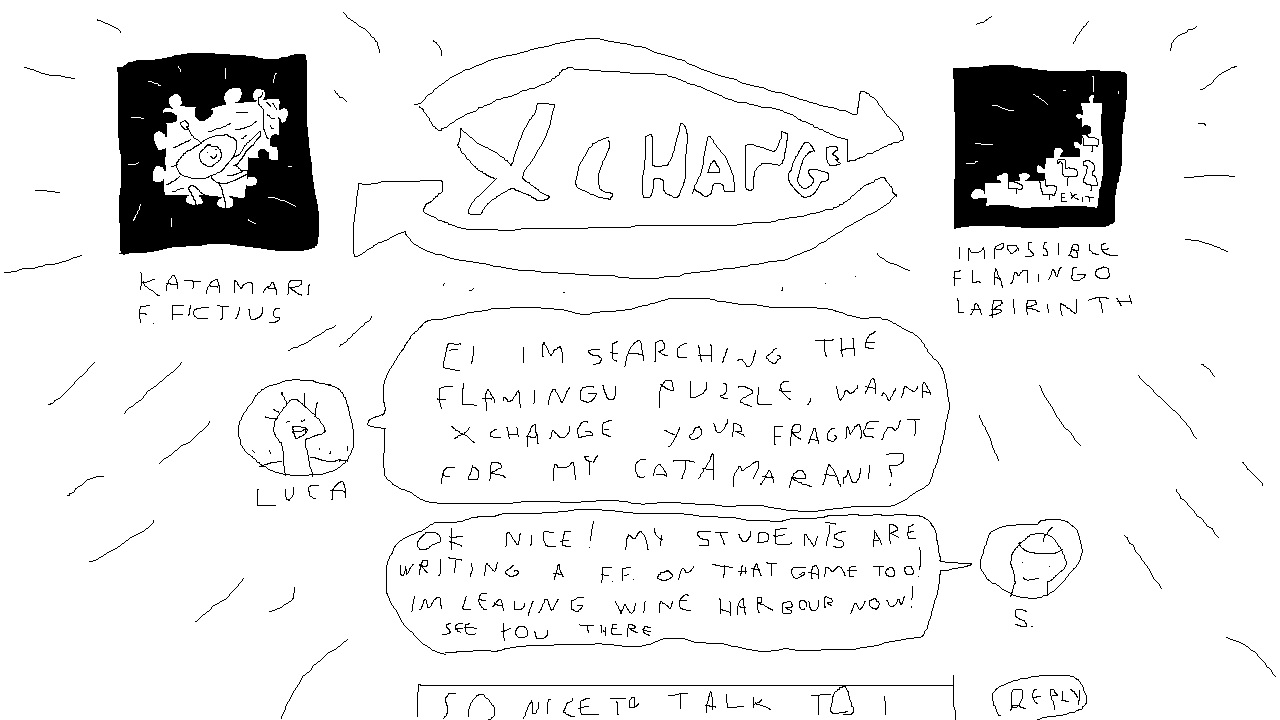 Usually I'm not really attached to my proposals and I'm always ready to give up to something in favor of something else. What I find really difficult it's to give up to something without a real alternative.
|
||||
|
||||
## Further developing interests
|
||||
|
||||
|
||||
<!-- I spent last year researching how different forms of intelligence (language, body, intuition, logic) can interact with complex (technological) systems. I would like to continue whit this using the tools and protocols I'm learning here at XPUB.
|
||||
|
||||
I would like to research more about emotional intelligence for example, especially when it comes to the forms of care that we are using during meetings or working sessions. How do they interact and influence our creative process and outcomes? Can we embed forms of care into software development? Can this help us approaching complexity with a wider emotional palette and more sensibility? -->
|
||||
|
||||
- How different forms of intelligence can interact with complexity (language, body, intuition, logic, emotion)
|
||||
- Coding as a form of care instead of control
|
||||
- Design pattern (OOP)
|
||||
- Learn how to play the accordion.
|
||||
|
||||
|
||||
<!-- usefull links
|
||||
|
||||
16
|
||||
|
||||
/soupboat/soup-gen/
|
||||
/soupboat/k-pub/
|
||||
/soupboat/padliography/
|
||||
|
||||
|
||||
/soupboat/~kamo/projects/concrete-label/
|
||||
/soupboat/si16/annotation-compass/
|
||||
|
||||
/soupboat/~kamo/projects/si16-backend/
|
||||
|
||||
17
|
||||
|
||||
/soupboat/xquisite/
|
||||
/soupboat/~kamo/projects/chaotic-evil-puzzles/
|
||||
|
||||
/soupboat/postit/
|
||||
|
||||
|
||||
-->
|
@ -0,0 +1,33 @@
|
||||
---
|
||||
title: test highlight
|
||||
---
|
||||
|
||||
```json
|
||||
{
|
||||
"image": "filename.jpg",
|
||||
"position": {"x": 12, "y": 97},
|
||||
"size": {"width": 43, "height": 18},
|
||||
"text": "Content of the annotation",
|
||||
"timestamp": "Wed, 01 Dec 2021 14:04:00 GMT",
|
||||
"userID": 123456789
|
||||
}
|
||||
```
|
||||
|
||||
```css
|
||||
.highlight .kn { color: #fb660a; font-weight: bold } /* Keyword.Namespace */
|
||||
.highlight .kp { color: #fb660a } /* Keyword.Pseudo */
|
||||
.highlight .kr { color: #fb660a; font-weight: bold } /* Keyword.Reserved */
|
||||
.highlight .kt { color: #cdcaa9; font-weight: bold } /* Keyword.Type */
|
||||
.highlight .ld { color: #ffffff } /* Literal.Date */
|
||||
.highlight .m { color: #0086f7; font-weight: bold } /* Literal.Number */
|
||||
.highlight .s { color: #0086d2 } /* Literal.String */
|
||||
.highlight .na { color: #ff0086; font-weight: bold } /* Name.Attribute */
|
||||
.highlight .nb { color: #ffffff } /* Name.Builtin */
|
||||
.highlight .nc { color: #ffffff } /* Name.Class */
|
||||
.highlight .no { color: #0086d2 } /* Name.Constant */
|
||||
```
|
||||
|
||||
```python
|
||||
def repeat(text, times):
|
||||
return (text * times)
|
||||
```
|
@ -0,0 +1,70 @@
|
||||
---
|
||||
title: appunti x brera
|
||||
description: proto slide
|
||||
css: brera.css
|
||||
---
|
||||
|
||||
## Ricerca
|
||||
|
||||
Ciao io mi chiamo FRANCESCO e la mia passione è sviluppare _software site specific_, cioè: che rispondono alla forma delle comunità in cui emergono. In questo modo è possibile generare relazioni e strumenti slegati dalle specifiche imposte dall'industria.
|
||||
|
||||
Il mio background è: vario (musica, video, animazione, graphic design, web design, programmazione, danza, ) e questo è il motivo per cui il mio portfolio è: altrettanto vario.
|
||||
|
||||
Ciò che accomuna i lavori è un approccio infrastrutturale: ogni progetto è anche il modo per avviare un discorso sul tipo di strumenti e interfacce che quella particolare situazione richiede o offre.
|
||||
|
||||
A un certo punto questo metodo diventa anche una riflessione sull'ecologia del software: come scegliamo i nostri strumenti? Cosa implica usare un'applicazione, un linguaggio, una tecnologia rispetto ad un'altra? Che relazioni si creano tra sviluppatore e utente, tra artista e pubblico? Ecc.
|
||||
|
||||
## Pratica
|
||||
|
||||
Strategie per affrontare la complessità contemporanea:
|
||||
|
||||
### rappresentare → intuizione e sintesi
|
||||
- interfacce per accedere alla complessità,
|
||||
- forme intuitive contro forme razionali,
|
||||
- sintesi contro semplificazione,
|
||||
|
||||
### documentare → narrazione situata
|
||||
- distanza critica tra il mondo e i mezzi usati per documentarlo.
|
||||
- un approccio che slega il reale dal fotorealistico (decolonizzare l'immagine?)
|
||||
|
||||
### abitare → esplorazione epistemologica
|
||||
- esplorare una condizione dall'interno per rinegoziarne i limiti e il possibile
|
||||
- accettare la complicità coi problemi e farne un punto di forza
|
||||
|
||||
## Lavori
|
||||
|
||||
|
||||
|
||||
### Object Oriented Choreography
|
||||
|
||||
|
||||
È una performance collaborativa: danzatrice nel VR interagisce con il pubblico.
|
||||
|
||||
Concept: Affrontare l'informazione digitale attraverso diversi tipi di intelligenza: corpo e parola. Costruire un modello in scala delle piattaforme digitali contemporanee e usarlo come dispositivo drammaturgico.
|
||||
|
||||

|
||||

|
||||
|
||||
Sviluppo: tesi, Triennale, RomaEuropa, futuro
|
||||
|
||||
Materiali: foto, video, UI, schemi, testo
|
||||
|
||||
### Un \* Salta
|
||||
|
||||
- Ricerca per un internet locale
|
||||
- Progetto e Viaggio in senegal
|
||||
- Pubblicazione e riflessioni
|
||||
|
||||
### Frana Futura
|
||||
|
||||
Ricerche per un documentario 3D che mette in relazione la condizione idrogeologica ligure e le realtà che la abitano.
|
||||
|
||||

|
||||
|
||||

|
||||
|
||||
### XPUB
|
||||
|
||||
- Relazione tra sistemi complessi e ambienti in cui vengono sviluppati
|
||||
- Temi
|
||||
- metodi
|
@ -0,0 +1,14 @@
|
||||
---
|
||||
title: Documentation workout
|
||||
description: hello this is a log of francesco
|
||||
---
|
||||
|
||||
Hello these are some logs from kamo. I like to develop software to inhabit complexity with visual and performative outcomes. You can find here a lot of works in progress and some finished projects. My resolution for these 2 years is to get good in documenting things I do.
|
||||
So this is basically workout.
|
||||
|
||||
Ciao
|
||||
|
||||
## TO DO##
|
||||
|
||||
- Dark mood
|
||||
- Fix chat reader
|
@ -0,0 +1,105 @@
|
||||
---
|
||||
title: Transcript of my assessment from the future
|
||||
css: recap.css
|
||||
cover: recap/cover.jpg
|
||||
cover_alt: dinosaurs
|
||||
---
|
||||
|
||||
## Hello
|
||||
|
||||
This months in XPUB were a lot of fun. At the beginning I was terrorized because of the moving, new people, new places, different language, so probably to cope with the stress I had an initial outburst of energies. Now I'm trying to stay calm.
|
||||
|
||||
## Purpose
|
||||
|
||||
Lately I've been interested in programming. Probably because it's a playful way to interact with reality starting from abstraction. Something like theory but with instant and tangible outputs. Something like incantation or psychokinesis.
|
||||
|
||||
I'm into the development of site-specific software. Codes that inhabit and interact with a community. Coding as a form of care. Programming as a way to facilitate _agency-on_ and _comprehension-of_ complex systems. I'm learning how to approach complexity as an environment. How a work can be complex without forcing the result being complicated.
|
||||
|
||||
## Methods
|
||||
|
||||
I like to propose ideas to the group. Sometimes the idea [does not deliver](/soupboat/~kamo/projects/loot-box-sealing-device/) , sometimes it lands but the results are [catastrophic](/soupboat/~kamo/projects/si16-structure-proposal/) , sometimes the outcomes are [unexpected](/soupboat/~kamo/projects/annotation-compass/) , and sometimes they [unexpectedly work](/soupboat/padliography/) .
|
||||
|
||||
My main interest is the ecology around these ideas. How it can be enriched and transformed by different voices, and the kind of play and space that it offers. I'm trying to shift from _developing crazy things_ to _developing meaningful things_. Meaningful especially in relation to the environment and the other people involved in the process. To be meaningful an idea should stands on its own, but functioning as a starting point, more than a finite result.
|
||||
|
||||
I get easily _bored-of_ or _carried-away-by_ my train of thoughts, so I need to rely on others. That's why I always collaborate with someone else and rarely do things alone.
|
||||
|
||||
|
||||
## Outputs
|
||||
|
||||
### Warm up
|
||||
|
||||
During the first month every day was a fest and we developed everything that came to our mind. To have the Soupboat felt empowering. A place to call home in the internet.   Not really a critical approach maybe, but an ecstatic condition.
|
||||
|
||||
Together with Erica we tried to develop a [shared cookbook](/soupboat/soup-gen/)  , then a [birthday cards collector](/soupboat/b-say/)  to spawn best wishes in the Soupboat homepage at each birthday, some prototypes for the [karaoke as a form of republishing](/soupboat/k-pub/) , a way to track all [our pads](/soupboat/padliography/)  through the media wiki API.
|
||||
|
||||
During the group exercise I collaborated to the [text lifeboats](/soupboat/~kamo/static/html/lifeboats/)  and different ways to [weave](/soupboat/~kamo/static/html/weaving/) texts together  to generate new meanings, transforming a complex writings into an [abstract chat](/soupboat/~kamo/static/html/chat-reader/chat.html) , and the [insecam livestream transcription](/soupboat/~kamo/static/html/cam-transcript/)   .
|
||||
|
||||
### SI16
|
||||
|
||||
I like what we did for the SI16. The concept of using an API as a form of pubblishing was [mindblowing](/soupboat/~kamo/projects/api-worldbuilding/). The approach proposed during the prototyping sessions was extremely stimulating, even if sometimes frightening to [rewind](/soupboat/~kamo/projects/si16-API-express-prototype/) and [rewind](/soupboat/~kamo/projects/si16-API-strapi-nuxt-prototype/) and [redo](/soupboat/~kamo/projects/si16-backend/) things differently . I feel sorry if at some point I pushed for something that someone perceived as a failure. I get that it's a matter of perspective: from my point of view what's important is the process and the ways we work together, while for someone else a concrete outcome is more important. I need to keep in mind that both sides are legit and valid.
|
||||
|
||||
I contributed on the overall [structure](/soupboat/~kamo/projects/si16-structure-proposal/), the [backend](/soupboat/~kamo/projects/si16-backend/) and the [frontend](/soupboat/~kamo/projects/si16-frontend-design/) , and the [visual identity](https://www.are.na/si16-visual-identity). The [Concrete Label](https://pad.xpub.nl/p/AGAINST_FILTERING) project started with Supi to annotate concrete poetry to create vernacular corpora  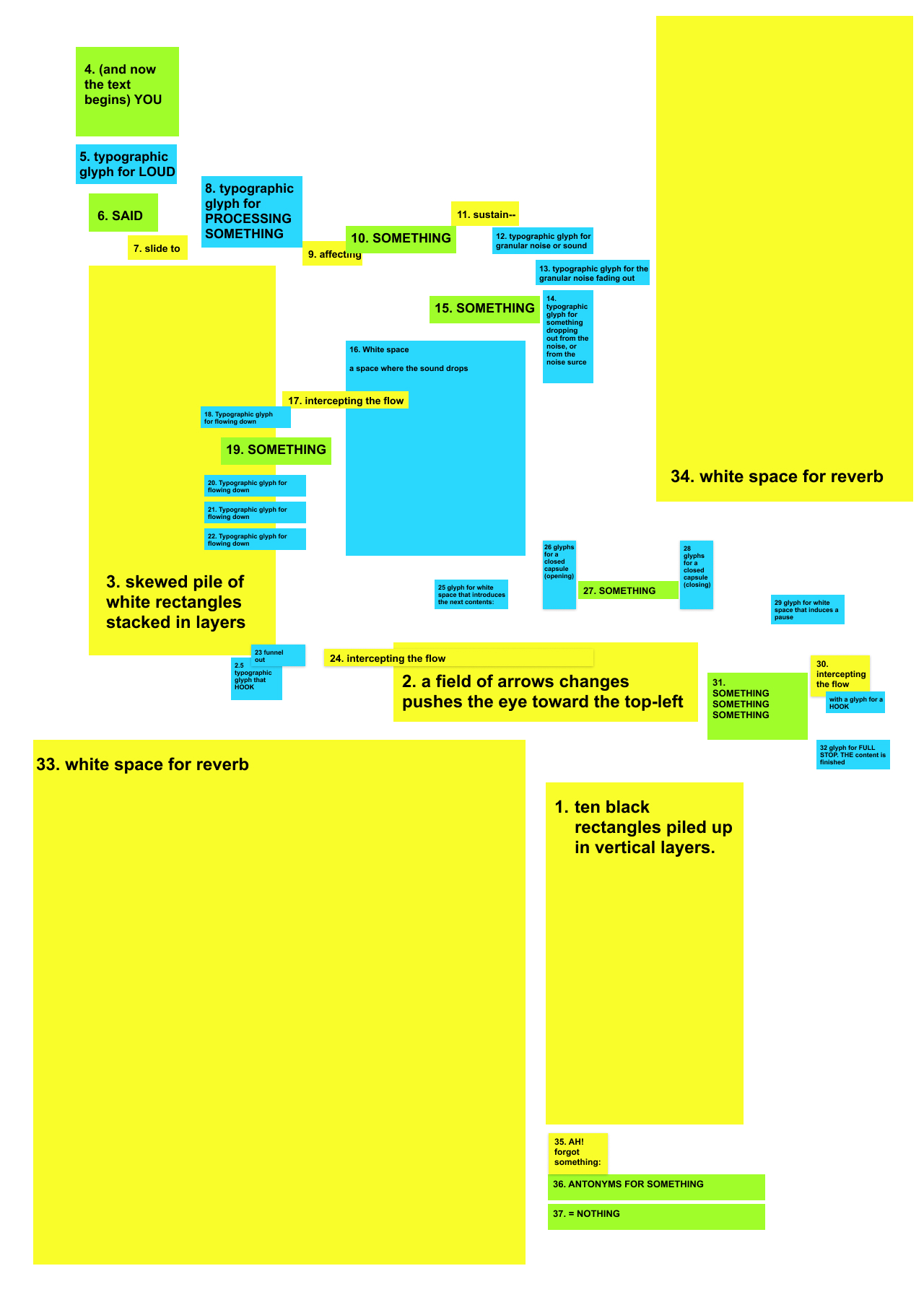 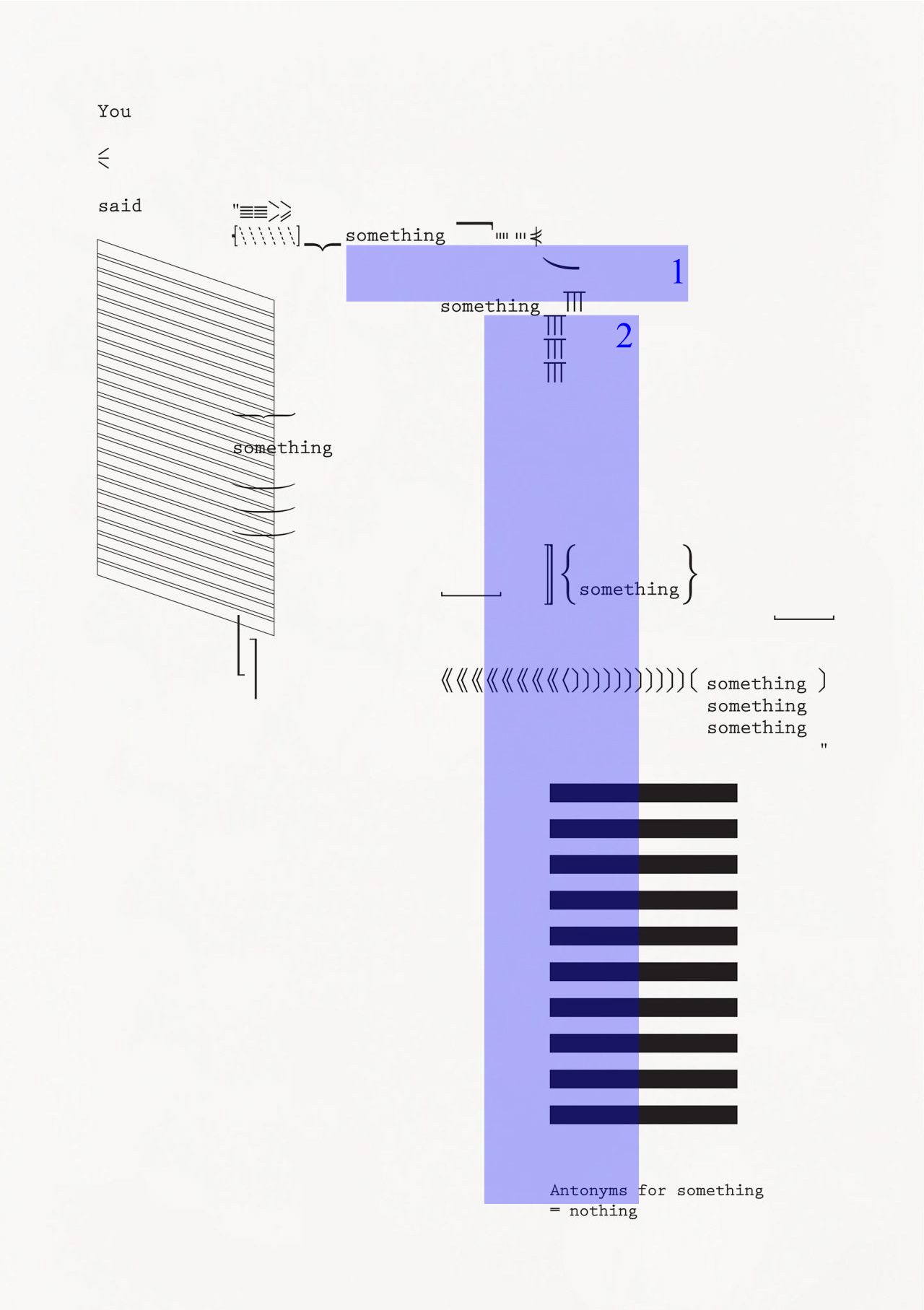 became the [Annotation Compass](/soupboat/~kamo/projects/annotation-compass/) , a tool to annotate images collectively.
|
||||
|
||||
We managed to achieve a lot: a distribuited API, a familiar CMS based on Jupiter Notebooks , a coherent environment with room for [customization](/soupboat/si16/projects/annotation-compass/rejection_map/), and a shared understanding of a complex topic . I tried to document these processes both in the soupboat and directly in the code . This required a lot of effort since I've always had a bad relation with documentation, probably because I'm an over-analitical-critical-shy person.
|
||||
|
||||
We failed in finishing things. The event-mode of the website as well as the API key circulation remain a draft. The workload was crazy, especially considered the technical difficulty of the overall project that led to unbalanced shares of work.
|
||||
|
||||
|
||||
### Slow down
|
||||
|
||||
After the winter break we slowed down, and spent more time studying the special issue's materials. Together with Erica, Mitsa, Chae, and Alex we read through all the text together. The ideology excercise with [noise canceling headphones](https://pad.xpub.nl/p/noice_cancelling_devices_turns_off_also_your_inner), the [critical karaoke](https://hub.xpub.nl/soupboat/postit/generate/karaoke) about gamification 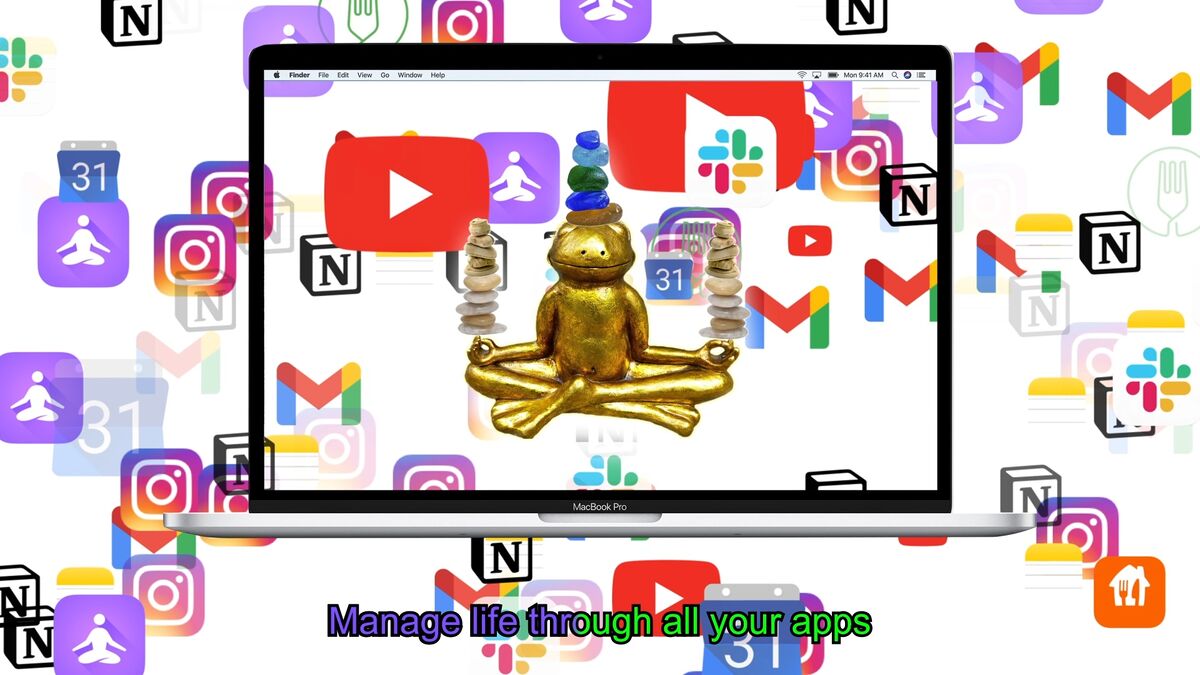, the katamari fanfiction , the [replace('mimic','loot box')](https://hub.xpub.nl/soupboat/postit/generate/mimic) research 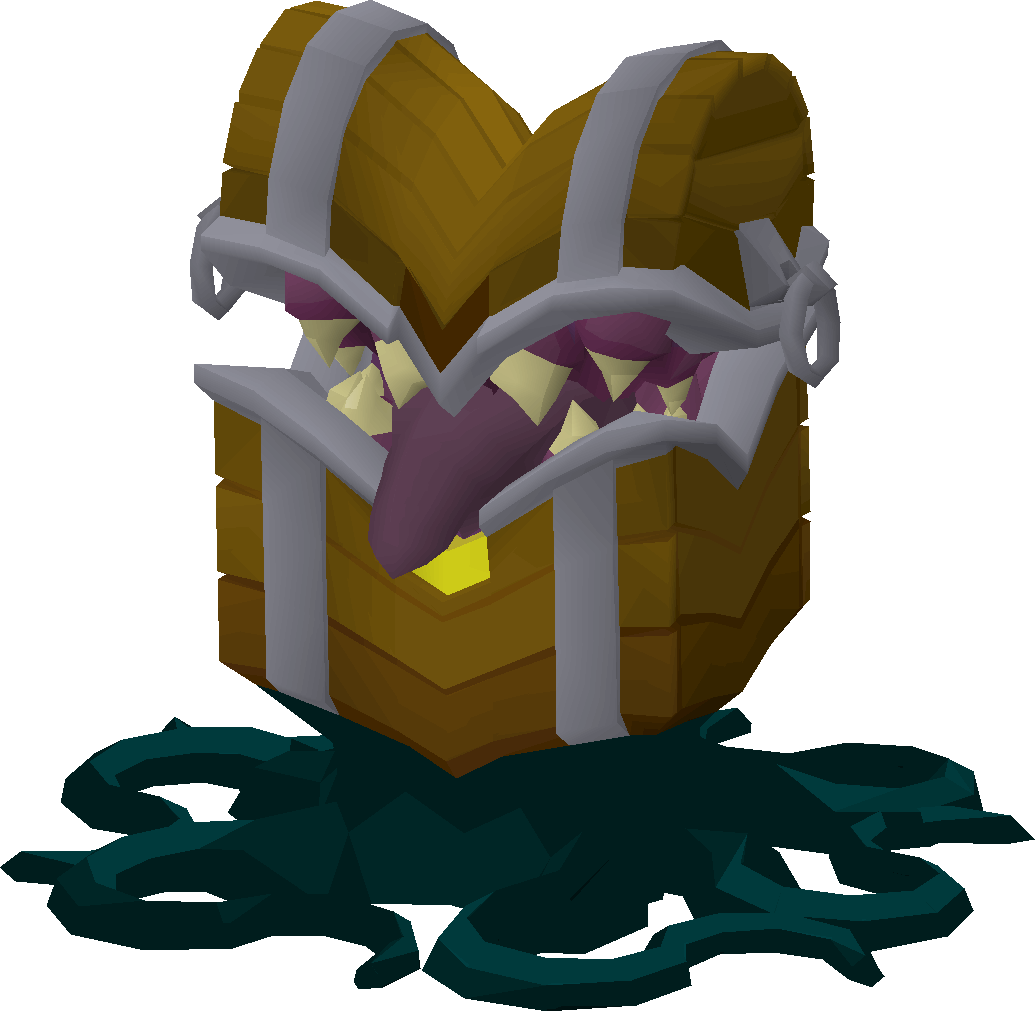, and the gravitational approach to mapping the theme of gamification 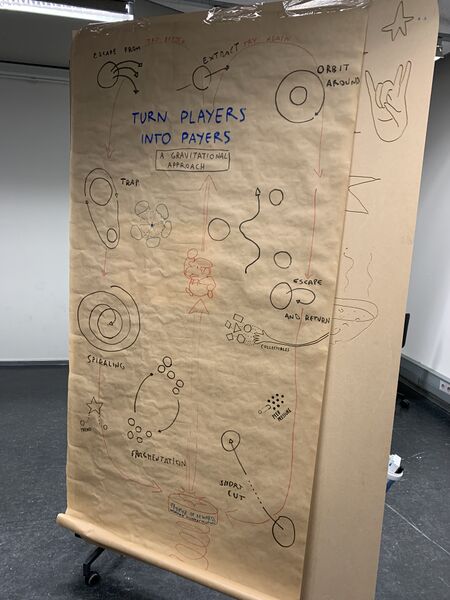 were all results of a moment with more critical focus on the contents and less on technical experiments.
|
||||
|
||||
At the beginning of the accademic year we applied to Room for Sound with the k-pub karaoke project, but timing and covid messed up with our plans, and in february after a couple of day of residency we decided to step back in order to focus more on less things.  Room for Sound understood our needs and proposed us to retry later on this year.
|
||||
|
||||
The plan now is to continue working on the karaoke format as a moment in which different forms of public meet each others: 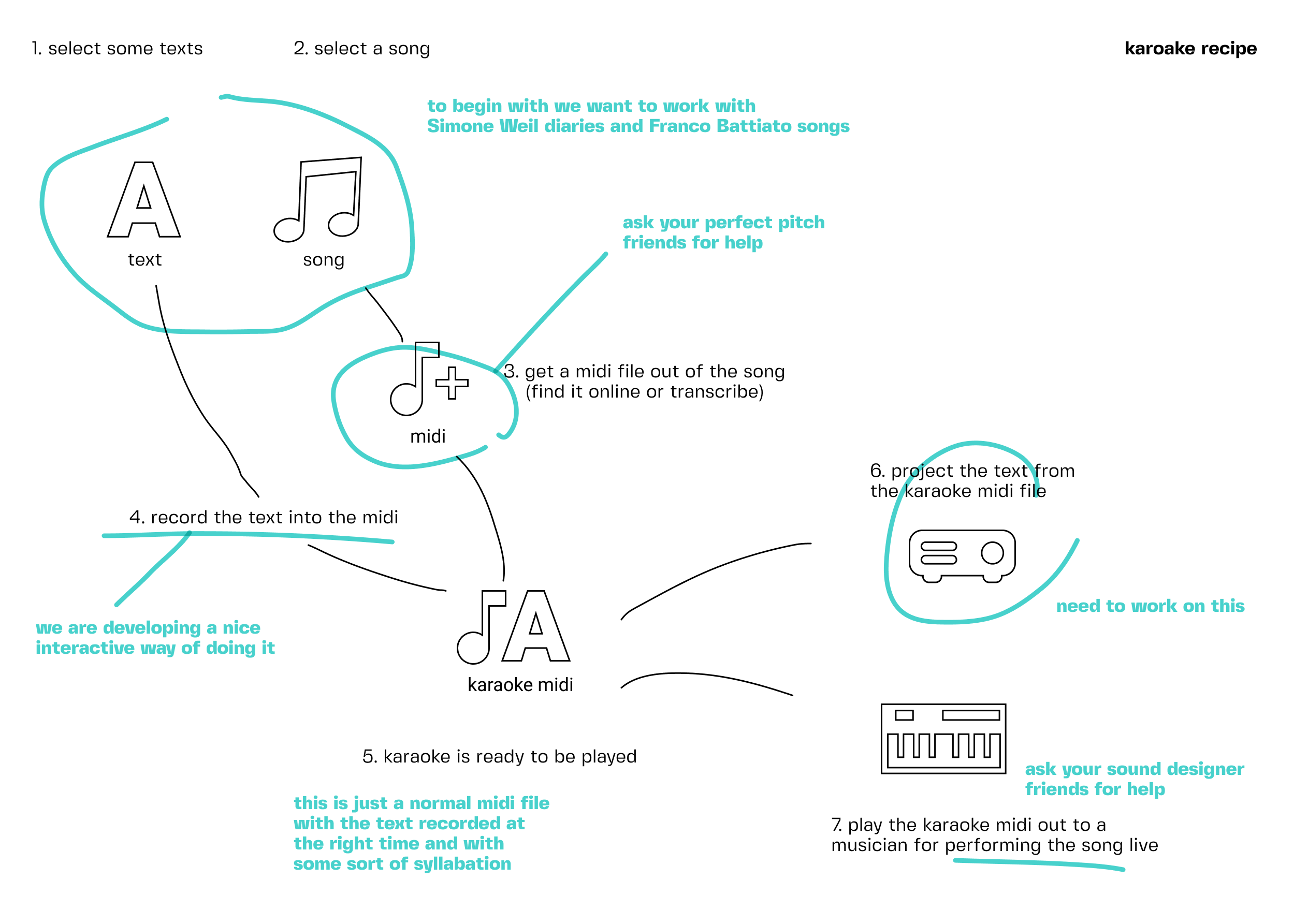 there is a text and writing aspect, a musical and sonic one, it's a collective and personal performative moment, and it's something already present in the collective imaginary. So it could be a good generative device.
|
||||
|
||||
### SI17
|
||||
|
||||
The process of SI17 was much more mindful than the previous one. As a group we put a lot of effort into the facilitation and organization of the work. The tired but cheering way we arrived at the launch is a sign of success.
|
||||
|
||||
The iterations of the work and the ideas were a lot, and the time passed on the readings was really effective to generate thoughts about the [relations within the public](https://hub.xpub.nl/soupboat/~kamo/projects/loot-box-multi-player/), the [jigsaw puzzle as a form of encryption ](https://hub.xpub.nl/soupboat/~kamo/projects/chaotic-evil-puzzles/) of our contents, the loot box as a [decorator or skin](https://hub.xpub.nl/soupboat/~kamo/projects/loot-box-decorator/) for other pubblications, [ways to seal](https://hub.xpub.nl/soupboat/~kamo/projects/loot-box-sealing-device/) 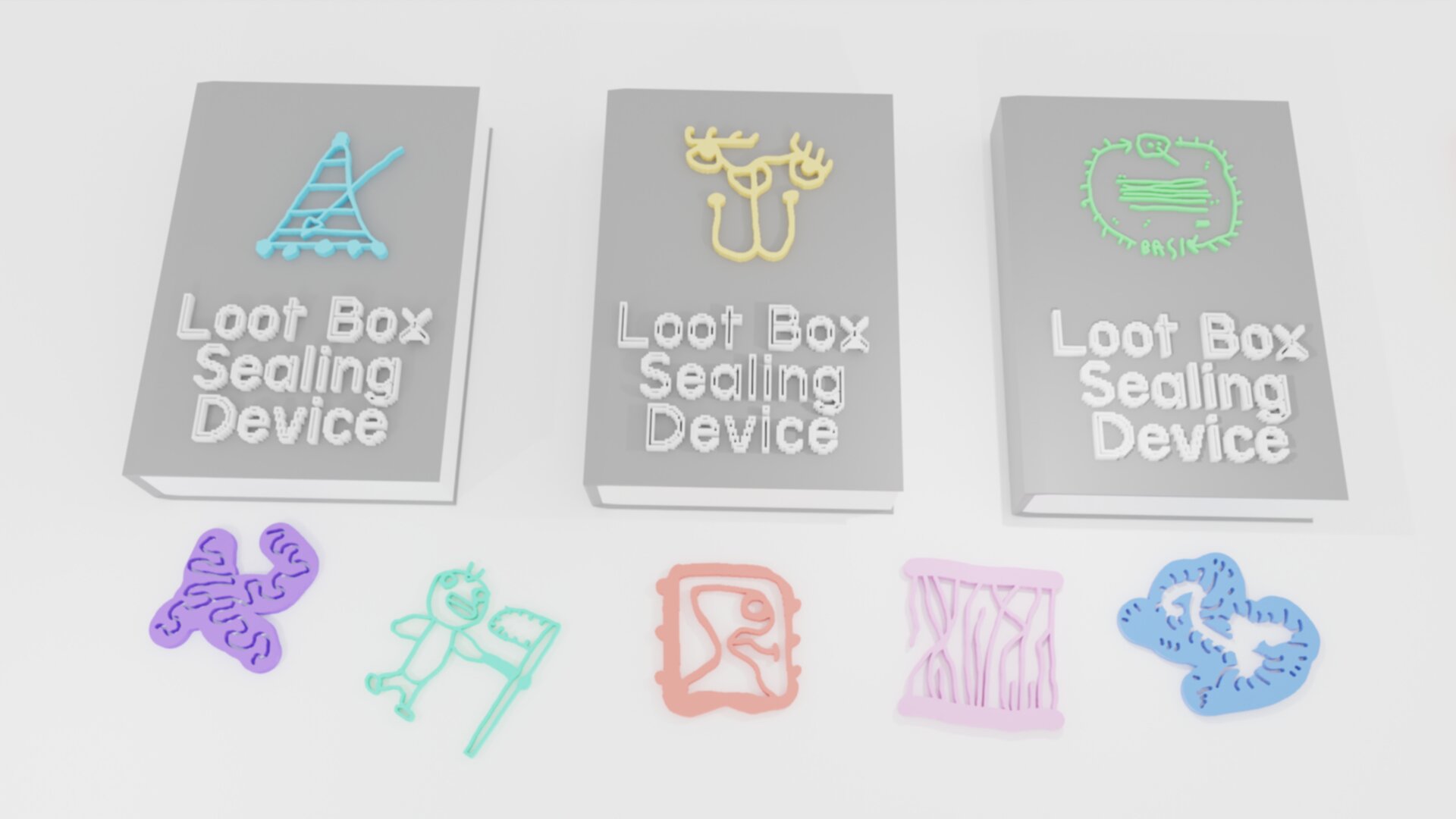 the boot lox instead of opening it, or ways to hack its inner [temporality](https://hub.xpub.nl/soupboat/~kamo/projects/loot-box-temporality/) .
|
||||
|
||||
When we entered the production phase I worked with Mitsa, Supi and Erica as part of the team 1, in charge of the contents of the boot lox. We approached the different contents with the idea of a common ground such as the [post-it](https://pad.xpub.nl/p/post-it).  We worked with a surface to [gather the contents](https://git.xpub.nl/kamo/post-it-contents)  and with another one to [generate the results](https://git.xpub.nl/kamo/postit)  . When Supi said that the way we worked made her rethink inDesign I was happy. Even if the perceived workload at some point was insane (tell me more about the blurred line between leisure and labor), the overall experience was super great, and we managed to work well together with a common pace.
|
||||
|
||||
Things went wrong only in the last days before sending the print, when we didn't manage to share the work in a fair way. To me this was a great loss since it was the main stake of the entire process. We had several reflections about this and then managed to recover the group morale working together on the website.
|
||||
|
||||
To keep things simple is difficult, but important. At the end I think the issue website  is great, since we did it altogether. It's not a problem if it's not technically interesting or flexible or modular or what else. I accepted that there are other parameters to create value and meaning. With the help of the group I also managed to give value to things I did such as the xquisite branch   and the mimic-loot box research , that ultimately ended as contents in the final pubblication.
|
||||
|
||||
Some moments were super hard: a certain Monday during spring break we should have decided on something to move on, but every proposal was rejected. It was really difficult, especially since there were valid ideas on the table. 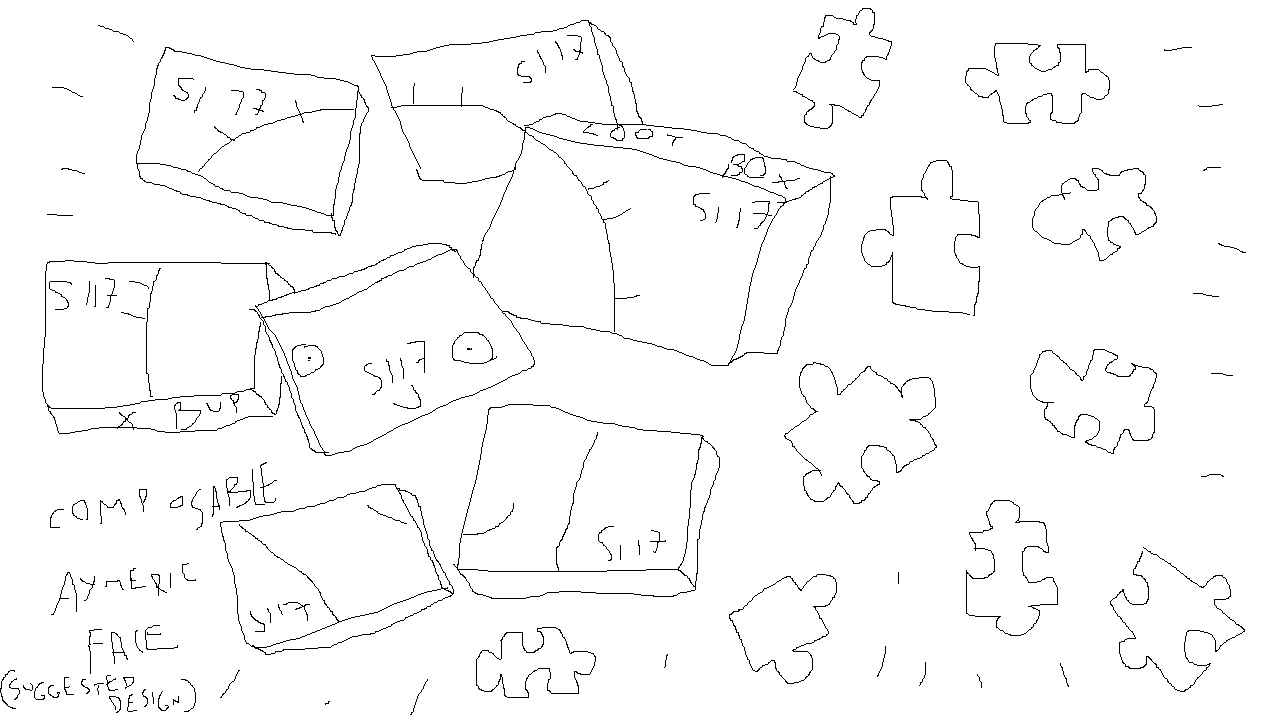  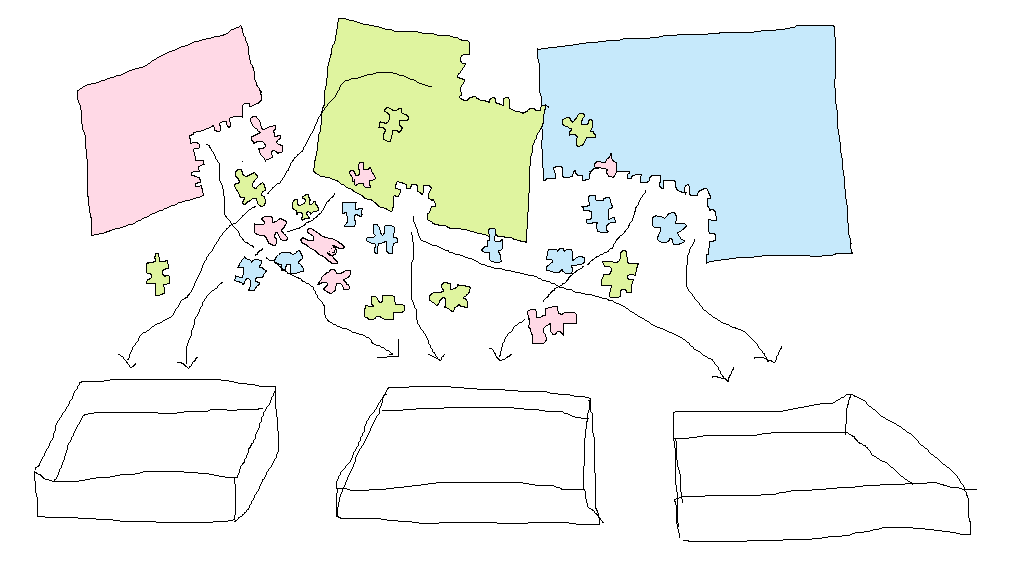 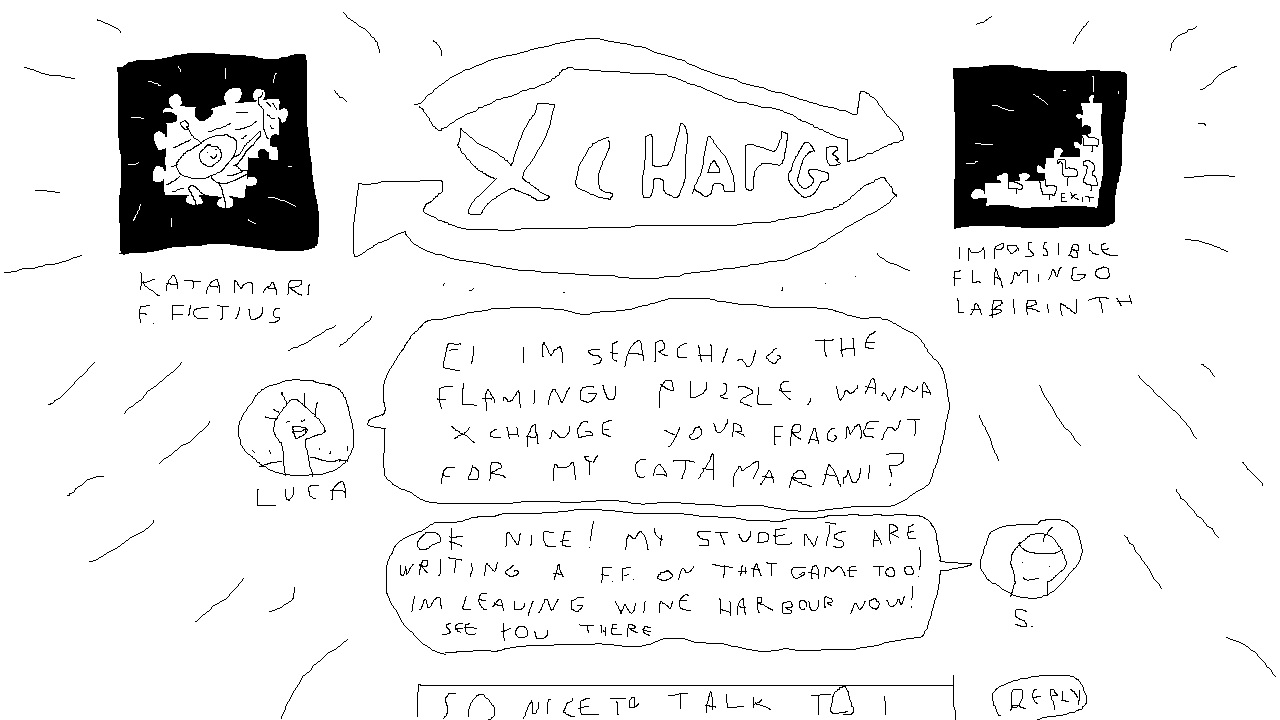 Usually I'm not really attached to my proposals and I'm always ready to give up to something in favor of something else. What I find really difficult it's to give up to something without a real alternative.
|
||||
|
||||
## Further developing interests
|
||||
|
||||
|
||||
<!-- I spent last year researching how different forms of intelligence (language, body, intuition, logic) can interact with complex (technological) systems. I would like to continue whit this using the tools and protocols I'm learning here at XPUB.
|
||||
|
||||
I would like to research more about emotional intelligence for example, especially when it comes to the forms of care that we are using during meetings or working sessions. How do they interact and influence our creative process and outcomes? Can we embed forms of care into software development? Can this help us approaching complexity with a wider emotional palette and more sensibility? -->
|
||||
|
||||
- How different forms of intelligence can interact with complexity (language, body, intuition, logic, emotion)
|
||||
- Coding as a form of care instead of control
|
||||
- Design pattern (OOP)
|
||||
- Learn how to play the accordion.
|
||||
|
||||
|
||||
<!-- usefull links
|
||||
|
||||
16
|
||||
|
||||
/soupboat/soup-gen/
|
||||
/soupboat/k-pub/
|
||||
/soupboat/padliography/
|
||||
|
||||
|
||||
/soupboat/~kamo/projects/concrete-label/
|
||||
/soupboat/si16/annotation-compass/
|
||||
|
||||
/soupboat/~kamo/projects/si16-backend/
|
||||
|
||||
17
|
||||
|
||||
/soupboat/xquisite/
|
||||
/soupboat/~kamo/projects/chaotic-evil-puzzles/
|
||||
|
||||
/soupboat/postit/
|
||||
|
||||
|
||||
-->
|
@ -0,0 +1,33 @@
|
||||
---
|
||||
title: test highlight
|
||||
---
|
||||
|
||||
```json
|
||||
{
|
||||
"image": "filename.jpg",
|
||||
"position": {"x": 12, "y": 97},
|
||||
"size": {"width": 43, "height": 18},
|
||||
"text": "Content of the annotation",
|
||||
"timestamp": "Wed, 01 Dec 2021 14:04:00 GMT",
|
||||
"userID": 123456789
|
||||
}
|
||||
```
|
||||
|
||||
```css
|
||||
.highlight .kn { color: #fb660a; font-weight: bold } /* Keyword.Namespace */
|
||||
.highlight .kp { color: #fb660a } /* Keyword.Pseudo */
|
||||
.highlight .kr { color: #fb660a; font-weight: bold } /* Keyword.Reserved */
|
||||
.highlight .kt { color: #cdcaa9; font-weight: bold } /* Keyword.Type */
|
||||
.highlight .ld { color: #ffffff } /* Literal.Date */
|
||||
.highlight .m { color: #0086f7; font-weight: bold } /* Literal.Number */
|
||||
.highlight .s { color: #0086d2 } /* Literal.String */
|
||||
.highlight .na { color: #ff0086; font-weight: bold } /* Name.Attribute */
|
||||
.highlight .nb { color: #ffffff } /* Name.Builtin */
|
||||
.highlight .nc { color: #ffffff } /* Name.Class */
|
||||
.highlight .no { color: #0086d2 } /* Name.Constant */
|
||||
```
|
||||
|
||||
```python
|
||||
def repeat(text, times):
|
||||
return (text * times)
|
||||
```
|
@ -0,0 +1,122 @@
|
||||
# IMPORT
|
||||
import os
|
||||
|
||||
from flask import Flask, render_template, request, url_for, redirect, jsonify, abort, send_from_directory
|
||||
import markdown
|
||||
import frontmatter
|
||||
from datetime import datetime
|
||||
|
||||
|
||||
# FUNCTIONS
|
||||
|
||||
|
||||
def list_files(folder, remove_ext=False):
|
||||
''' Read all the functions in a folder '''
|
||||
names = []
|
||||
for entry in os.scandir(folder):
|
||||
# add to the list only proper files
|
||||
if entry.is_file(follow_symlinks=False):
|
||||
# remove the extension from the filename
|
||||
n = os.path.splitext(entry.name)[0]
|
||||
if remove_ext:
|
||||
n = entry.name
|
||||
names.append(n)
|
||||
return names
|
||||
|
||||
|
||||
def list_folders(folder):
|
||||
''' Return all the folders in a folder '''
|
||||
names = []
|
||||
for entry in os.scandir(folder):
|
||||
# add to the list only proper files
|
||||
if not entry.name.startswith('.') and entry.is_dir():
|
||||
# remove the extension from the filename
|
||||
names.append(entry.name)
|
||||
return names
|
||||
|
||||
|
||||
def get_md_contents(filename, directory='./contents'):
|
||||
''' Return contents from a filename as frontmatter handler '''
|
||||
with open(f"{directory}/{filename}", "r") as f:
|
||||
metadata, content = frontmatter.parse(f.read())
|
||||
html_content = markdown.markdown(content, extensions=['markdown.extensions.attr_list','markdown.extensions.codehilite','markdown.extensions.fenced_code'])
|
||||
return metadata, html_content
|
||||
|
||||
|
||||
# FLASK APP
|
||||
base_url = "~kamo"
|
||||
projects = 'projects'
|
||||
|
||||
# create flask application
|
||||
app = Flask(__name__,
|
||||
static_url_path=f'/soupboat/{base_url}/static',
|
||||
static_folder=f'/soupboat/{base_url}/static')
|
||||
# Markdown(app, extensions=['extra'])
|
||||
# app.jinja_env.extend(jinja2_highlight_cssclass = 'codehilite')
|
||||
|
||||
|
||||
# add the base_url variable to all the flask templates
|
||||
@app.context_processor
|
||||
def set_base_url():
|
||||
return dict(base_url=base_url)
|
||||
|
||||
|
||||
# Homepage
|
||||
@app.route(f"/{base_url}/")
|
||||
def home_page():
|
||||
|
||||
# get the basic info of the website from the /contents/home.md file
|
||||
meta, content = get_md_contents("home.md")
|
||||
projects_list = []
|
||||
for project in list_folders("./projects"):
|
||||
project_info = get_md_contents("documentation.md",
|
||||
f"./{projects}/{project}")[0]
|
||||
project_date = datetime.strptime(project_info['date'], '%d/%m/%Y')
|
||||
project_info['date'] = datetime.strftime(project_date, '%d %b, %y')
|
||||
project_info['categories'].sort()
|
||||
|
||||
project_info['slug'] = project
|
||||
projects_list.append(project_info)
|
||||
|
||||
projects_list.sort(reverse=True, key=lambda project: datetime.strptime(
|
||||
project['date'], '%d %b, %y'))
|
||||
|
||||
# get the list of the projects, the functions, and the corpora
|
||||
home = {
|
||||
**meta,
|
||||
"content": content,
|
||||
"projects": projects_list
|
||||
}
|
||||
return render_template("home.html", **home)
|
||||
|
||||
|
||||
# For generic pages we can include a common template and change only the contents
|
||||
@app.route(f"/{base_url}/<slug>/")
|
||||
def dynamic_page(slug=None):
|
||||
# meta is a dictionary that contains all the attributes in the markdown file (ex: title, description, soup, etc)
|
||||
# content is the body of the md file aka the text content
|
||||
# in this way we can access those frontmatter attributes in jinja simply using the variables title, description, soup, etc
|
||||
meta, content = get_md_contents(f"{slug}.md")
|
||||
return render_template("page.html", **meta, content=content)
|
||||
|
||||
|
||||
# Single project
|
||||
@app.route(f"/{base_url}/projects/<project>/")
|
||||
def p_info(project=None):
|
||||
meta, content = get_md_contents("documentation.md",
|
||||
f"./{projects}/{project}")
|
||||
template = 'project.html'
|
||||
if 'template' in meta:
|
||||
template = meta['template']
|
||||
return render_template(template, **meta, content=content)
|
||||
|
||||
|
||||
|
||||
|
||||
@app.route(f'/{base_url}/projects/<project>/<path:filename>')
|
||||
def sendStaticFiles(project, filename):
|
||||
return send_from_directory(app.root_path + f'/projects/{project}/', filename, conditional=True)
|
||||
|
||||
|
||||
# RUN
|
||||
app.run(port="3132")
|
@ -1,11 +0,0 @@
|
||||
@font-face {
|
||||
font-family: "Ortica";
|
||||
font-weight: bold;
|
||||
src: url("./Ortica-Bold.woff2") format("woff2"), url("./Ortica-Bold.woff") format("woff");
|
||||
}
|
||||
|
||||
@font-face {
|
||||
font-family: "Ortica";
|
||||
font-weight: normal;
|
||||
src: url("./Ortica-Light.woff2") format("woff2"), url("./Ortica-Light.woff") format("woff");
|
||||
}
|
@ -1,36 +0,0 @@
|
||||
<!DOCTYPE html>
|
||||
<html lang="en">
|
||||
<head>
|
||||
<meta charset="UTF-8" />
|
||||
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
|
||||
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
|
||||
<title>Kamo</title>
|
||||
<link rel="stylesheet" href="global.css" />
|
||||
<link rel="stylesheet" href="style.css" />
|
||||
<script src="name.js" defer></script>
|
||||
<script src="cms.js" defer></script>
|
||||
</head>
|
||||
<body>
|
||||
<h1 class="title">probably hungry</h1>
|
||||
<div class="info">
|
||||
hello this is a log of francesco also and only known as
|
||||
<span id="name-space">kamo</span>
|
||||
<a href=".." class="back">back to the soupboat</a>
|
||||
</div>
|
||||
|
||||
<table id="table">
|
||||
<!-- <tr>
|
||||
<td class="title"><a href="05-10-2021-weaving/">Text Weaving</a></td>
|
||||
<td class="links">
|
||||
<a href="https://git.xpub.nl/kamo/text_weaving">git</a>
|
||||
</td>
|
||||
<td class="categories">
|
||||
<span class="tag">Python</span>
|
||||
<span class="tag">NLTK</span>
|
||||
<span class="tag">Text</span>
|
||||
</td>
|
||||
<td class="date">10-05-2021</td>
|
||||
</tr> -->
|
||||
</table>
|
||||
</body>
|
||||
</html>
|
@ -1,36 +0,0 @@
|
||||
const nameSpace = document.getElementById("name-space");
|
||||
|
||||
const list = [
|
||||
"kamo",
|
||||
"sumo",
|
||||
"camo",
|
||||
"gamo",
|
||||
"fra",
|
||||
"salamino",
|
||||
"zuppetta",
|
||||
"soup eater",
|
||||
"soul eater",
|
||||
"sound eater",
|
||||
"k",
|
||||
"eheheh",
|
||||
"🗿",
|
||||
"🐡",
|
||||
"fridge haunter",
|
||||
"fridge hunter",
|
||||
"fridge terror",
|
||||
"niiiice",
|
||||
"slice",
|
||||
"BUT",
|
||||
];
|
||||
|
||||
function randomName() {
|
||||
return list[Math.floor(Math.random() * list.length)];
|
||||
}
|
||||
|
||||
setInterval(() => {
|
||||
nameSpace.innerHTML = randomName();
|
||||
nameSpace.classList.add("rotate");
|
||||
setTimeout(() => {
|
||||
nameSpace.classList.remove("rotate");
|
||||
}, 5000);
|
||||
}, 5000);
|
@ -0,0 +1,25 @@
|
||||
---
|
||||
title: Cam Transcript
|
||||
date: 12/10/2021
|
||||
pad: https://pad.xpub.nl/p/cam-stranscript
|
||||
project: cam-transcript
|
||||
description: 10 minutes transcription from Insecam webcams
|
||||
categories:
|
||||
- Text
|
||||
- Video
|
||||
---
|
||||
|
||||
|
||||
|
||||
## Video Transcribing
|
||||
|
||||
[SI16 - with Cristina and Manetta](https://pad.xpub.nl/p/SP16_1210)
|
||||
|
||||
In groups of 2-3:
|
||||
|
||||
1. Decide a video to transcribe (max 10 min)
|
||||
2. If you can't decide on one, take 3-5 minutes to think about a subject of everyday knowledge that is particular to a location/group. Record yourself telling the story
|
||||
3. Transcribe individually either the video or your own recording
|
||||
4. Compare the transcriptions
|
||||
|
||||
fun with Kimberley + Carmen
|
@ -0,0 +1,25 @@
|
||||
---
|
||||
title: Cam Transcript
|
||||
date: 12/10/2021
|
||||
pad: https://pad.xpub.nl/p/cam-stranscript
|
||||
project: cam-transcript
|
||||
description: 10 minutes transcription from Insecam webcams
|
||||
categories:
|
||||
- Text
|
||||
- Video
|
||||
---
|
||||
|
||||
|
||||
|
||||
## Video Transcribing
|
||||
|
||||
[SI16 - with Cristina and Manetta](https://pad.xpub.nl/p/SP16_1210)
|
||||
|
||||
In groups of 2-3:
|
||||
|
||||
1. Decide a video to transcribe (max 10 min)
|
||||
2. If you can't decide on one, take 3-5 minutes to think about a subject of everyday knowledge that is particular to a location/group. Record yourself telling the story
|
||||
3. Transcribe individually either the video or your own recording
|
||||
4. Compare the transcriptions
|
||||
|
||||
fun with Kimberley + Carmen
|
@ -0,0 +1,31 @@
|
||||
---
|
||||
title: Chameleon RRPG 🦎
|
||||
description: A Random Role Play Game to inject micro scripted actions in daily life
|
||||
date: 01/03/2022
|
||||
categories:
|
||||
- Games
|
||||
cover: KecleonCelestialStorm122.jpg
|
||||
cover_alt: The chameleon pokemon named Keckleon
|
||||
template: cards.html
|
||||
cards:
|
||||
- KecleonCelestialStorm122.jpg
|
||||
- KecleonLostThunder161.jpg
|
||||
- KecleonLostThunder162.jpg
|
||||
css: cards.css
|
||||
---
|
||||
|
||||
The game requires a social context and can be played by any number of players.
|
||||
|
||||
Each player is a chameleon that mimetize from the others. the chameleon hidden awaits for its prey: usuallly a random insect.
|
||||
|
||||
At the beginning of the game each chameleon is assigned with a random insect: a secret simple action to be done with a certain degree of absurd (provide examples)
|
||||
|
||||
If the chameleon manages to catch its insect without being noticed from the others the game continue.
|
||||
|
||||
When all the chameleons have eaten they win together.
|
||||
|
||||
If a chameleon finds out that another one is eating, it moves faster its sticky tongue to steal the prey. he win, but everyone else loose and the game ends.
|
||||
|
||||
If the chameleon who tried to steal the instect was wrong and the other was not eating but just mimetizing as always, the latter is hit by the sticky tongue in one eye, and the game continues.
|
||||
|
||||
To facilitate the mimecy and the secrecy the chameleons have developed a simple website from which each participant could receive an insect, and notify when it eats it. when everyone have notified their lunch the website ring and give an award to everyone
|
@ -0,0 +1,32 @@
|
||||
---
|
||||
title: Chameleon RRPG 🦎
|
||||
description: A Random Role Play Game to inject micro scripted actions in daily life
|
||||
date: 01/03/2022
|
||||
categories:
|
||||
- Games
|
||||
cover: KecleonCelestialStorm122.jpg
|
||||
cover_alt: The chameleon pokemon named Keckleon
|
||||
template: cards.html
|
||||
cards:
|
||||
- KecleonCelestialStorm122.jpg
|
||||
- KecleonLostThunder161.jpg
|
||||
- KecleonLostThunder162.jpg
|
||||
css: cards.css
|
||||
---
|
||||
|
||||
The game requires a social context and can be played by any number of players.
|
||||
|
||||
Each player is a chameleon that mimetize from the others. the chameleon hidden awaits for its prey: usuallly a random insect.
|
||||
|
||||
At the beginning of the game each chameleon is assigned with a random insect: a secret simple action to be done with a certain degree of absurd (provide examples)
|
||||
|
||||
If the chameleon manages to catch its insect without being noticed from the others the game continue.
|
||||
|
||||
When all the chameleons have eaten they win together.
|
||||
|
||||
If a chameleon finds out that another one is eating, it moves faster its sticky tongue to steal the prey. he win, but everyone else loose and the game ends.
|
||||
|
||||
If the chameleon who tried to steal the instect was wrong and the other was not eating but just mimetizing as always, the latter is hit by the sticky tongue in one eye, and the game continues.
|
||||
|
||||
To facilitate the mimecy and the secrecy the chameleons have developed a simple website from which each participant could receive an insect, and notify when it eats it. when everyone have notified their lunch the website ring and give an award to everyone
|
||||
|
@ -0,0 +1,193 @@
|
||||
---
|
||||
title: Chaotic evil puzzles
|
||||
description: Jigsaw puzzle as a form of encryption of our SI17
|
||||
date: 18/02/2022
|
||||
cover: 91AC-jbMPsL._AC_SL1500_.jpg
|
||||
cover_alt: white japanes jigsaw puzzle
|
||||
git: https://git.xpub.nl/kamo/chaospuzzles
|
||||
categories:
|
||||
- Proposal
|
||||
- SI17
|
||||
- Games
|
||||
---
|
||||
|
||||
## There are 100 lot boxes with 100 different jigsaw puzzles of 100 pieces.*
|
||||
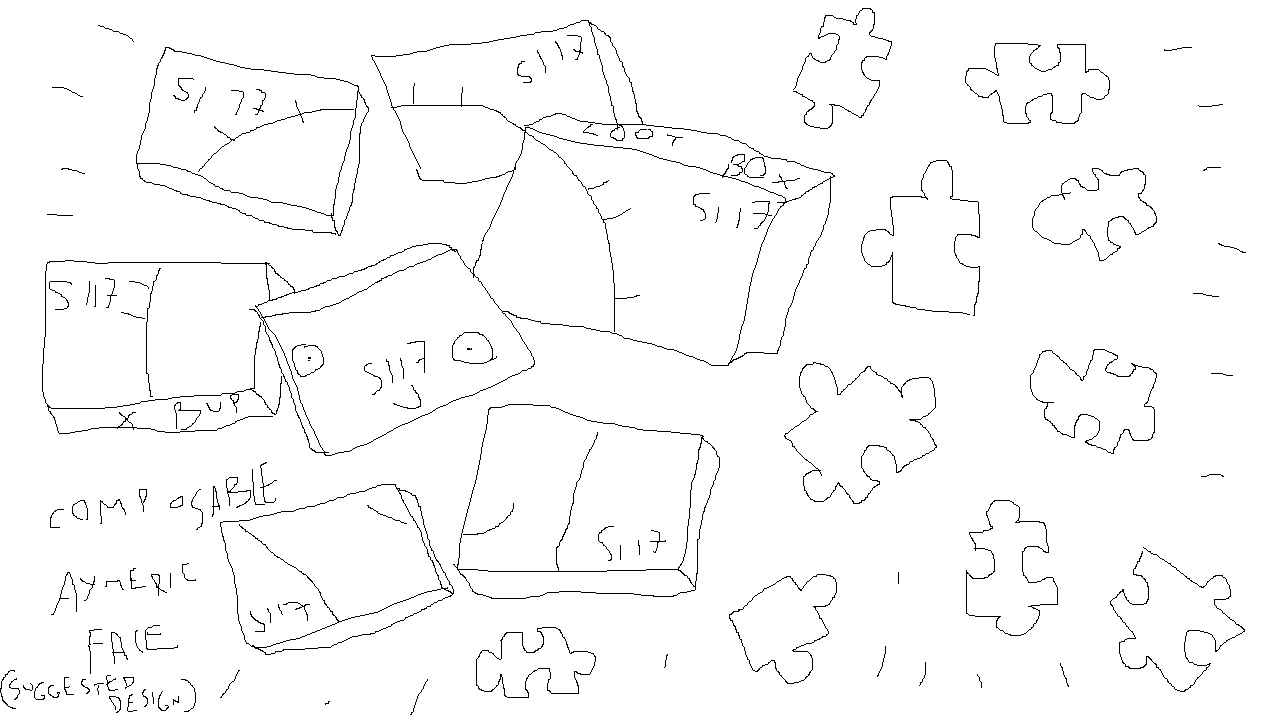
|
||||
_\* (exact quantities to be defined)_
|
||||
|
||||
## The picture on each puzzles is a content related to our experiments, games and researches for the SI17
|
||||

|
||||
|
||||
Each puzzle is an A2 sized image displaying the works we did during this trimester, designed in a way that can be interesting for the players to buy it, even for the sake of doing a jigsaw puzzle itself.
|
||||
|
||||
_f.e. A puzzle could be the rules for an RPG; or the map of a bitsy game with some critical texts about gamification; a generated maze, a fan fiction, the glossary, the list of one sentence games, etc._
|
||||
|
||||
In other words, the collection of puzzles will be a sort of inventory of our research framed in the form of jigsaw.
|
||||
|
||||
## The pieces are scattered through all the loot boxes, in a way that each one contains parts of multiple puzzles.
|
||||
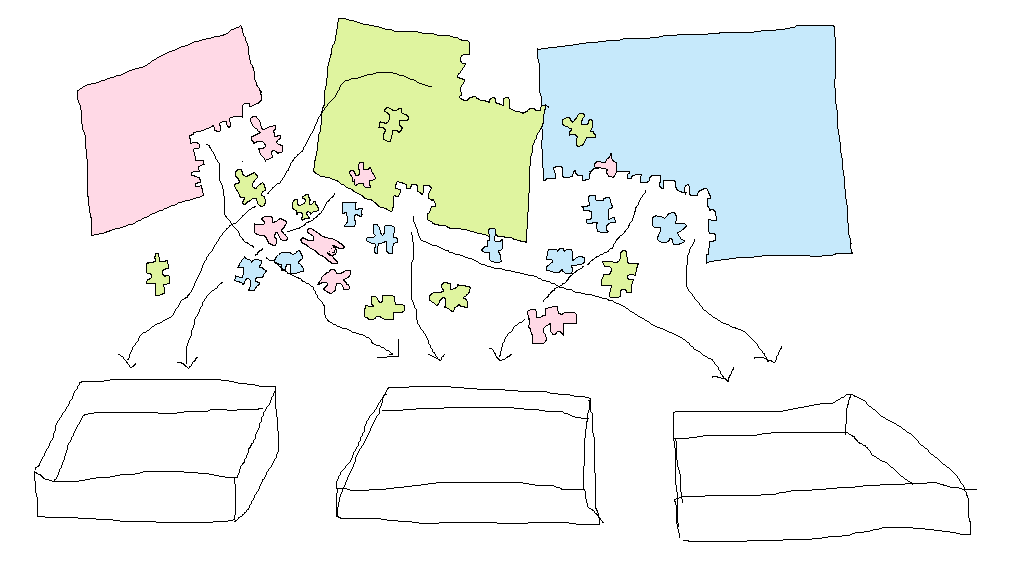
|
||||
|
||||
This could be done in a meaningful way: the idea is not to have total random pieces, but legible fragments from each content.
|
||||
|
||||
## When players buy the loot box they can compose the puzzle, but the result is a patchwork of different images.
|
||||
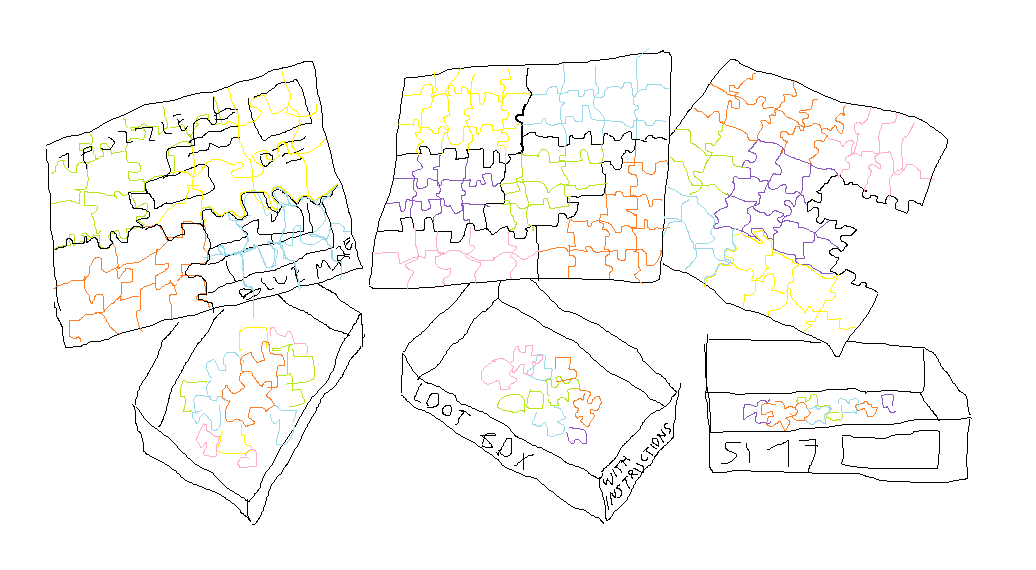
|
||||
|
||||
If the puzzles have different images but the same pieces pattern, each loot box can have a complete puzzle, but with mixed pieces. In this way we can avoid the frustration that having an incomplete jigsaw puzzle could cause.
|
||||
|
||||
## On the website of SI17 the players can upload their fragments, and compose together an online version to complete all the jigsaw puzzles.
|
||||

|
||||
|
||||
We can numerate or identify each piece of the puzzles with a code. This could be done when we generate the pattern of the puzzle with Python 👀. To upload a fragment of puzzle, the player is required to insert the code of the pieces, and maybe take a picture. In this way we can be sure that only who has the fragment can insert it online.
|
||||
|
||||
|
||||
## Optional feature: users can upload pictures of their fragments and we could have a collective documentation of the work.
|
||||
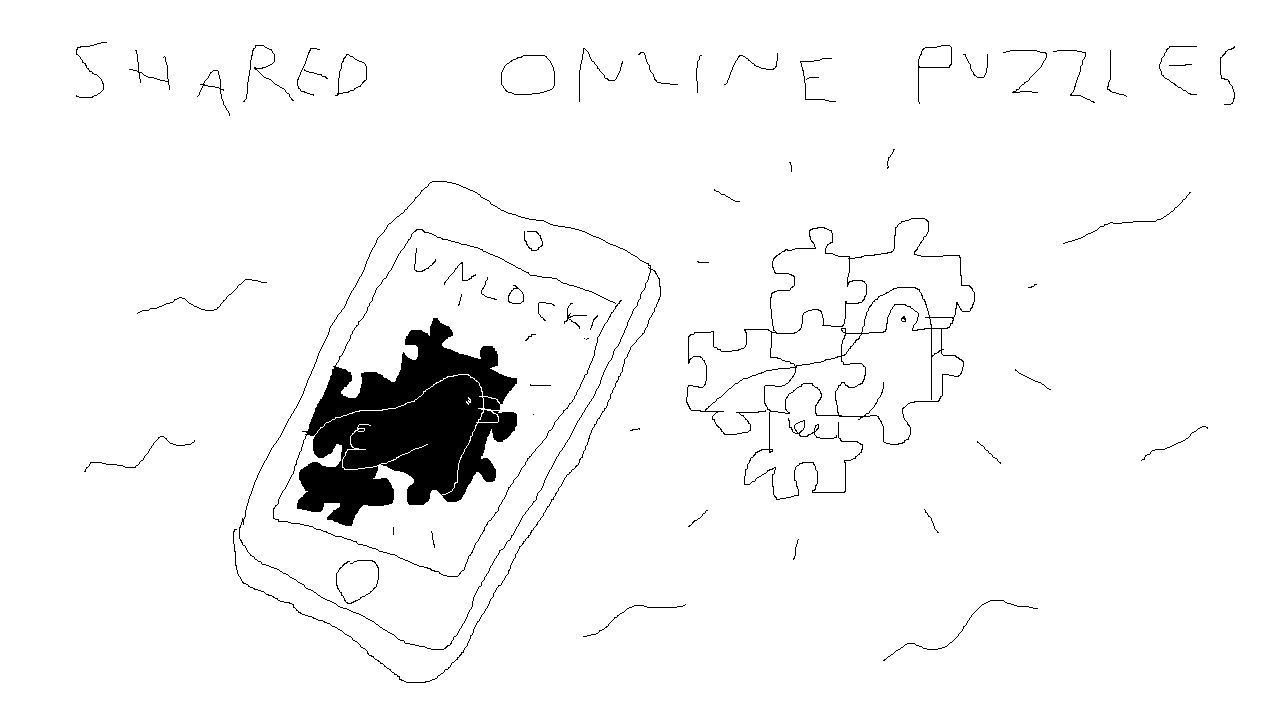
|
||||
_This is not unpaid work, it's participation_
|
||||
|
||||
Nice feature for the website could be that you can see the digital version of the puzzle, but on mouse :hover we could show the pictures from the public. A puzzle of photos of puzzles. This could be challenging but funny to develop.
|
||||
|
||||
## On the website of SI17 there is a community section for exchanging the fragments and complete the puzzle
|
||||
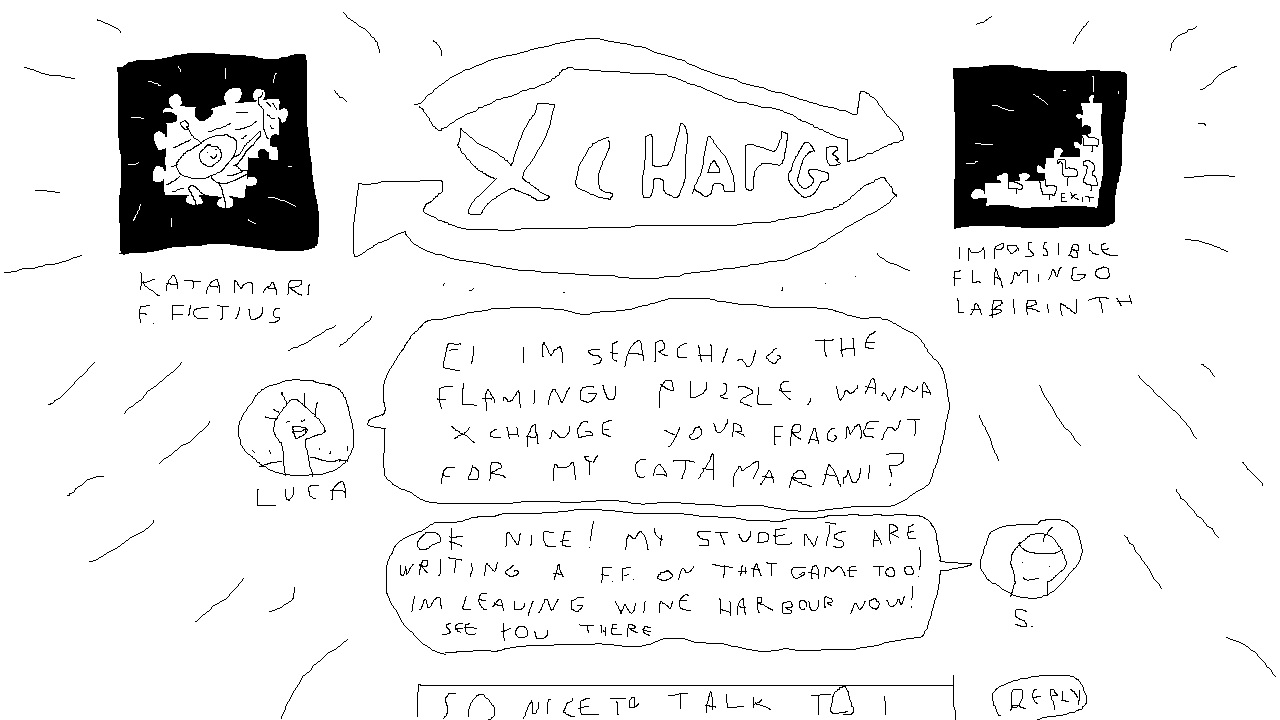
|
||||
|
||||
The community section with users and exchange etc could be tricky, but we can stay as simple as possible and do it with Flask. The exchange section should exclude by design the speculation on the pieces or money. A fragment for a fragment.
|
||||
|
||||
## On the website of SI17 the public can access to the experiments, games and researches as well
|
||||

|
||||
|
||||
In this way we can provide access to the contents such as the bitsy games, the karaoke video, the ruleset of our games, the reading list, etc.
|
||||
|
||||
### Risk / Benefit assessment
|
||||
|
||||
PROS
|
||||
+ simple to make
|
||||
+ accessible because it's a well known game
|
||||
+ a lot of design
|
||||
+ not to much code
|
||||
+ use what we already have
|
||||
+ interesting interaction with the public
|
||||
+ performative element ready for the launch
|
||||
+ multiple temporalities (individual puzzle, contents, shared puzzles)
|
||||
+ world building
|
||||
|
||||
CONS:
|
||||
- not an API 👀
|
||||
- i don't like puzzles
|
||||
- people mught not appreciate the fact of missing parts of their puzzle, but we're here to subvert it, and contents will be available online anyways
|
||||
|
||||
### Bonus: summary workflow
|
||||
|
||||

|
||||
The process to make the puzzles could be easy as design - print - cut - shuffle - package, nothing more (+ website)
|
||||
|
||||
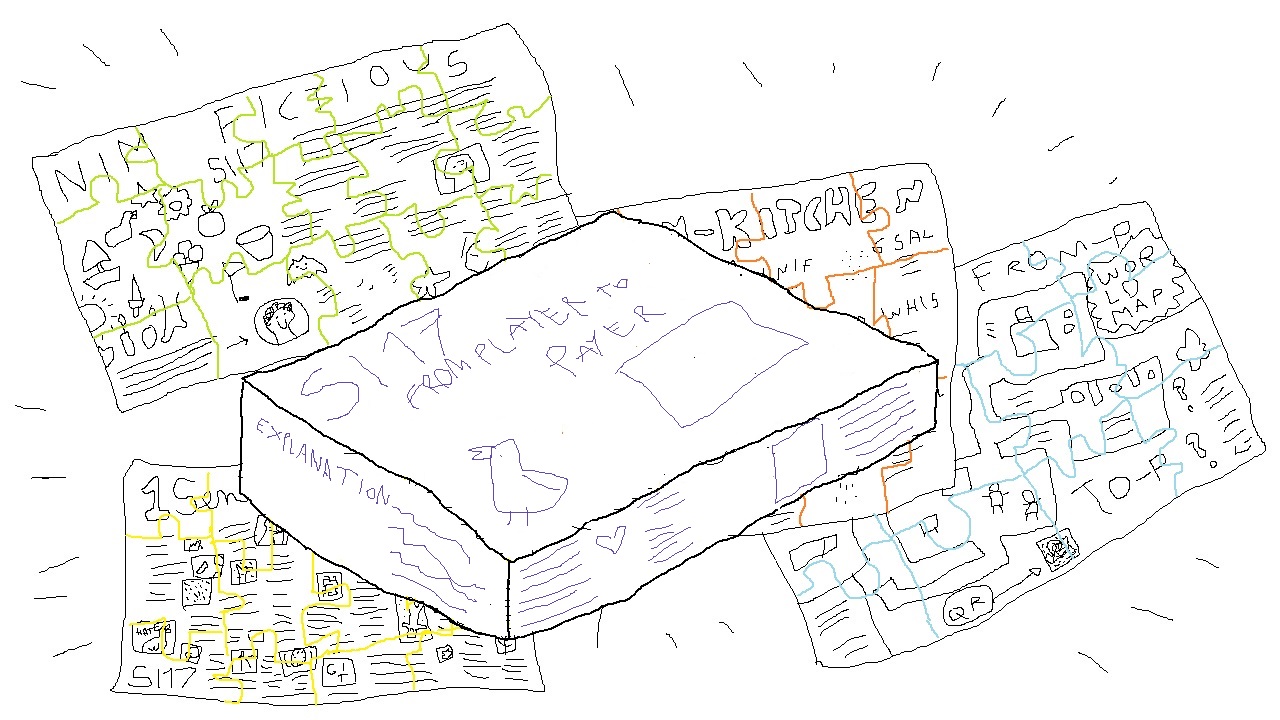
|
||||
The loot box could provide a context and the instruction of the game, as well as the link to the website.
|
||||
|
||||
|
||||
## Scenario
|
||||
|
||||
Mapping the chaotic evil puzzles in the through the different scenari
|
||||
|
||||
### of the form
|
||||
|
||||
_scenario 1: The lootbox is a physical box that contains something_
|
||||
|
||||
Fragments of several puzzles.
|
||||
|
||||
### of the feature
|
||||
|
||||
_scenario 7: The items in the loot-box are complementary and it is necessary to connect with other loot-box owners in order to assemble the pieces together._
|
||||
|
||||
There is a single player aspect and there is a collaborative aspect. These two components could be mediated by an online platform, such as the online shared puzzles, but could also work offline if people just combine the pieces of their loot boxes.
|
||||
|
||||
### of the contents
|
||||
|
||||
_scenario 2: The loot box is a collection of the prototyped games (and researches!) we did so far curated in some kind of form_
|
||||
|
||||
The jigsaw puzzle is just a form of encryption of our contents. A shared surface in which we can publish really different things such as a fan fiction, a board game, a link to a video karaoke or a videogame, an essay, the characters of a roleplaying game, etc.
|
||||
|
||||
_scenario 3: The loot box is a collection of mini-games + an ultimate game that has to be performed with other players that purcahsed the LB_
|
||||
|
||||
There are several layers of playability and access:
|
||||
|
||||
1. to solve the fragments (single player jigsaw)
|
||||
2. to access the contents (games, texts, etc. )
|
||||
3. to combine the fragments (ultimate multiplayer game, re-distribuition of the loot boxes contents)
|
||||
|
||||
|
||||
_scenario 6: The lootbox contains a series of jigsaw puzzles but their pieces are scattered through all the boxes and there is a platform online where you can see the missing tiles._
|
||||
|
||||
Nothing to declare.
|
||||
|
||||
|
||||
## Prototype (look at git!)
|
||||
|
||||
This is a rough prototype for generating ready-to-print jigsaw puzzles as well as a way to track their completion in a shared platform.
|
||||
The idea is to have several puzzles and mix their pieces, in a way that invites the players to collaborate in order to solve them.
|
||||
|
||||
This prototype covers two aspects of the process: the first is to split an image into pieces, tracking every piece with an ID and store the relation between adjacent tiles. The second concerns the online platform, and it is a Flask application that permits to upload cluster of pieces in order to share them with the other players and unlocking the full puzzle.
|
||||
|
||||
### To install the project:
|
||||
|
||||
1. Clone the repo
|
||||
2. Create a virtual environment
|
||||
```
|
||||
$ python3 -m venv venv
|
||||
```
|
||||
3. Activate the virtual environment
|
||||
```
|
||||
$ . venv/bin/activate
|
||||
```
|
||||
4. Install the dependencies
|
||||
```
|
||||
$ pip install -e .
|
||||
```
|
||||
5. Set the environmental variables for flask
|
||||
```
|
||||
$ export FLASK_APP=flaskr
|
||||
$ export FLASK_ENV=development
|
||||
$ flask run
|
||||
```
|
||||
6. The Flask application will be accessible on your browser at `localhost:5000`. If you try to navigate there you will see a blank page. This is because we need to generate some puzzles to display.
|
||||
|
||||
### Generating the contents
|
||||
|
||||
The first thing to do then is to run the `split.py` script:
|
||||
|
||||
```
|
||||
python3 chaospuzzles/split.py
|
||||
```
|
||||
|
||||
This will take the Katamari demo picture from `static/img` and will split it in tiles. The tiles will be used to compose the clusters when a player upload it online. In this way we can be super flexible in the randomization / distribuition of the pieces.
|
||||
|
||||
You can tweak the parameters at the end of the split.py file. This is temporary, later on it would be nice to have an interface to prepare the puzzle.
|
||||
|
||||
### Completing the puzzles
|
||||
|
||||
If you reload the website, you will see a link pointing to the Katamari page. Here we will find an empty frame, and a form to insert new pieces.
|
||||
|
||||
Try it! You can find the IDs for the pieces in the `chaospuzzles/puzzles/katamari/katamari_retro.png` image. This is the picture generated in order to be printed behind the puzzle and let every piece to have a unique ID.
|
||||
|
||||
By design an valid cluster is a group of adjacent pieces.
|
||||
|
||||
Keep in mind that this is a wip and rough prototype so everything need to be polished a lot. We count on your imagination to fill the lack of design, UI and UX here. Imagination is the best modern feature of 2022!
|
||||
|
||||
Thanks and see you sun ☀️
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
## memos
|
||||
|
||||
- play with quantities and distribuition of pieces (1 piece only, large groups, variations, etc)
|
||||
- play with puzzle pattern: alternative to the mainstream shape of the tiles
|
||||
- pieces naming system
|
||||
- And then the aim is to exchange pieces or something and rebuild the original puzzles? (can this be a critical approach?) (does this make sense only if there are as many puzzles as loot boxes?)
|
||||
- short term puzzles (link to multimedia contents, puzzle shards)
|
||||
- long term puzzles (hidden messages, 1 word in each puzzles and a secret sentence)
|
||||
- size and quantity
|
||||
- The jigsaw puzzles results should be secret? There would be much more mistery. Can we reveal only during the launch, but not on the loot boxes? Maybe is a compromise, but maybe is not necessary. Should we reveal them only in the website meanwhile they are completed? Could be.
|
||||
- [Generate Jigsaw Puzzle with python](https://github.com/jkenlooper/piecemaker)
|
||||
- [How To Laser Cut a Jigsaw Puzzle](https://www.youtube.com/watch?v=xqhOrY8unn4)
|
||||
- [Jigsaw puzzle generator](https://draradech.github.io/jigsaw/index.html)
|
||||
- [Fractlal Jigsaw](https://proceduraljigsaw.github.io/Fractalpuzzlejs/)
|
@ -0,0 +1,193 @@
|
||||
---
|
||||
title: Chaotic evil puzzles
|
||||
description: Jigsaw puzzle as a form of encryption of our SI17
|
||||
date: 18/02/2022
|
||||
cover: 91AC-jbMPsL._AC_SL1500_.jpg
|
||||
cover_alt: white japanes jigsaw puzzle
|
||||
git: https://git.xpub.nl/kamo/chaospuzzles
|
||||
categories:
|
||||
- Proposal
|
||||
- SI17
|
||||
- Games
|
||||
---
|
||||
|
||||
## There are 100 lot boxes with 100 different jigsaw puzzles of 100 pieces.*
|
||||
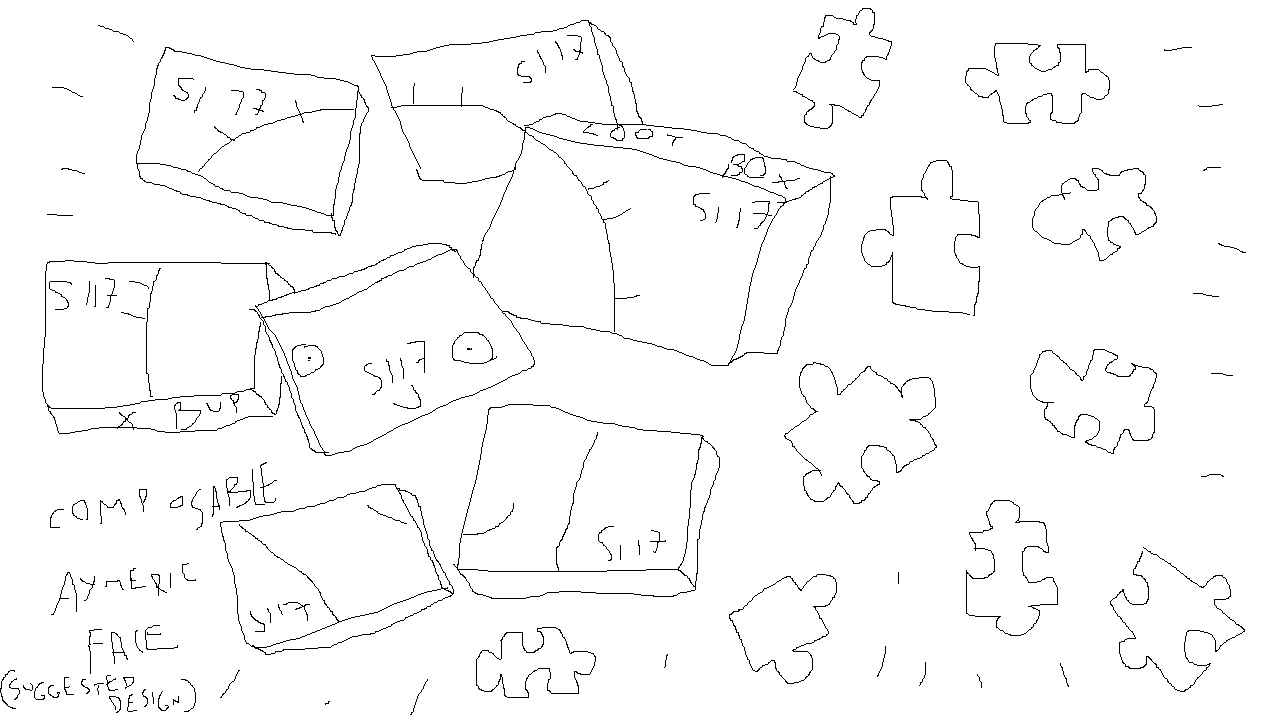
|
||||
_\* (exact quantities to be defined)_
|
||||
|
||||
## The picture on each puzzles is a content related to our experiments, games and researches for the SI17
|
||||

|
||||
|
||||
Each puzzle is an A2 sized image displaying the works we did during this trimester, designed in a way that can be interesting for the players to buy it, even for the sake of doing a jigsaw puzzle itself.
|
||||
|
||||
_f.e. A puzzle could be the rules for an RPG; or the map of a bitsy game with some critical texts about gamification; a generated maze, a fan fiction, the glossary, the list of one sentence games, etc._
|
||||
|
||||
In other words, the collection of puzzles will be a sort of inventory of our research framed in the form of jigsaw.
|
||||
|
||||
## The pieces are scattered through all the loot boxes, in a way that each one contains parts of multiple puzzles.
|
||||
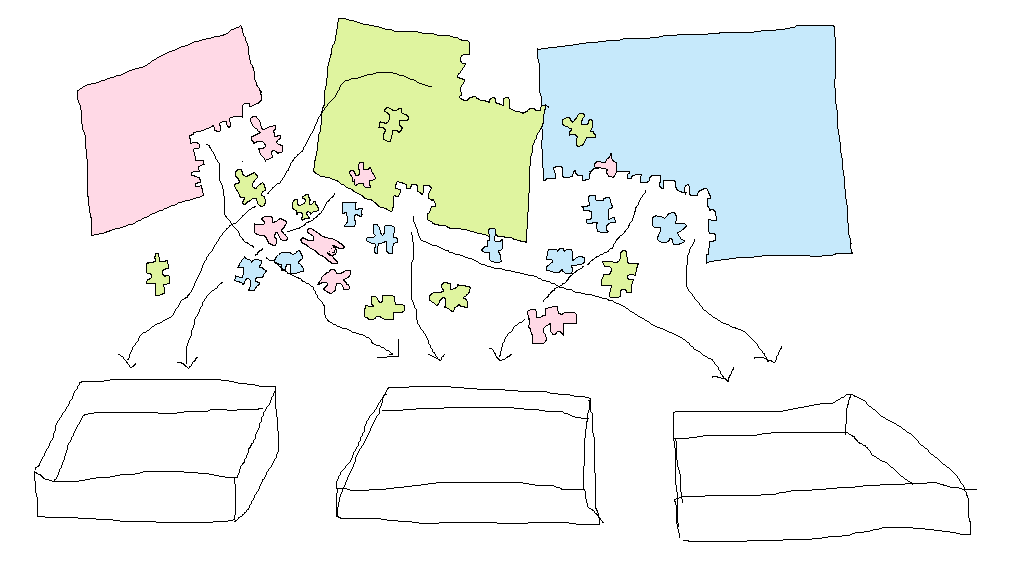
|
||||
|
||||
This could be done in a meaningful way: the idea is not to have total random pieces, but legible fragments from each content.
|
||||
|
||||
## When players buy the loot box they can compose the puzzle, but the result is a patchwork of different images.
|
||||
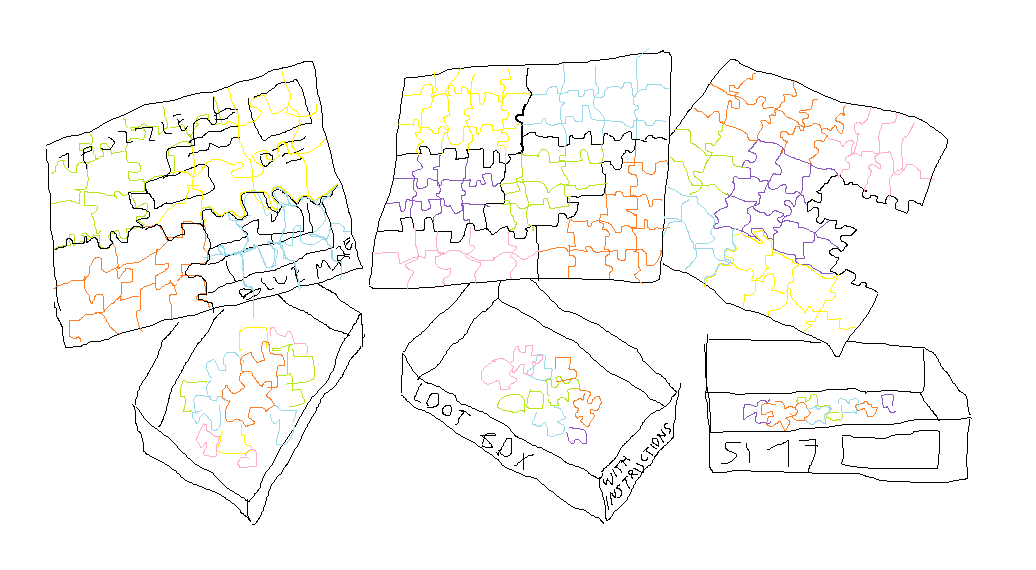
|
||||
|
||||
If the puzzles have different images but the same pieces pattern, each loot box can have a complete puzzle, but with mixed pieces. In this way we can avoid the frustration that having an incomplete jigsaw puzzle could cause.
|
||||
|
||||
## On the website of SI17 the players can upload their fragments, and compose together an online version to complete all the jigsaw puzzles.
|
||||

|
||||
|
||||
We can numerate or identify each piece of the puzzles with a code. This could be done when we generate the pattern of the puzzle with Python 👀. To upload a fragment of puzzle, the player is required to insert the code of the pieces, and maybe take a picture. In this way we can be sure that only who has the fragment can insert it online.
|
||||
|
||||
|
||||
## Optional feature: users can upload pictures of their fragments and we could have a collective documentation of the work.
|
||||
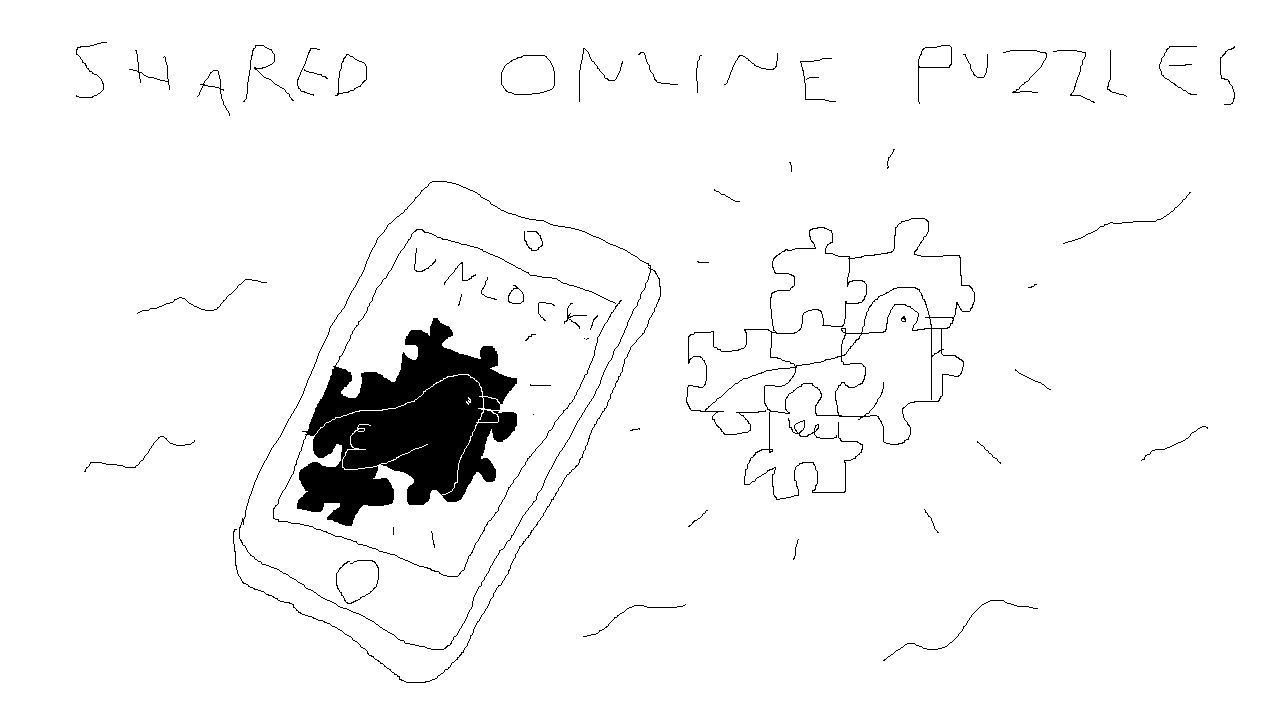
|
||||
_This is not unpaid work, it's participation_
|
||||
|
||||
Nice feature for the website could be that you can see the digital version of the puzzle, but on mouse :hover we could show the pictures from the public. A puzzle of photos of puzzles. This could be challenging but funny to develop.
|
||||
|
||||
## On the website of SI17 there is a community section for exchanging the fragments and complete the puzzle
|
||||
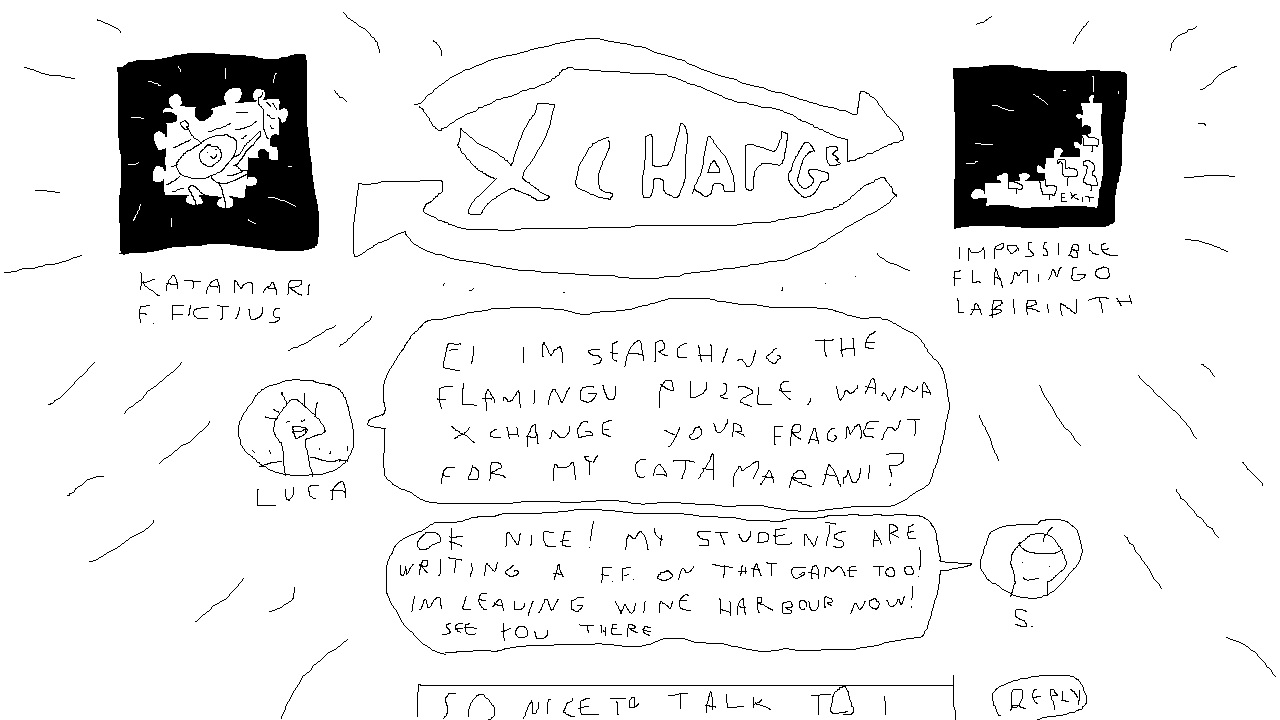
|
||||
|
||||
The community section with users and exchange etc could be tricky, but we can stay as simple as possible and do it with Flask. The exchange section should exclude by design the speculation on the pieces or money. A fragment for a fragment.
|
||||
|
||||
## On the website of SI17 the public can access to the experiments, games and researches as well
|
||||

|
||||
|
||||
In this way we can provide access to the contents such as the bitsy games, the karaoke video, the ruleset of our games, the reading list, etc.
|
||||
|
||||
### Risk / Benefit assessment
|
||||
|
||||
PROS
|
||||
+ simple to make
|
||||
+ accessible because it's a well known game
|
||||
+ a lot of design
|
||||
+ not to much code
|
||||
+ use what we already have
|
||||
+ interesting interaction with the public
|
||||
+ performative element ready for the launch
|
||||
+ multiple temporalities (individual puzzle, contents, shared puzzles)
|
||||
+ world building
|
||||
|
||||
CONS:
|
||||
- not an API 👀
|
||||
- i don't like puzzles
|
||||
- people mught not appreciate the fact of missing parts of their puzzle, but we're here to subvert it, and contents will be available online anyways
|
||||
|
||||
### Bonus: summary workflow
|
||||
|
||||

|
||||
The process to make the puzzles could be easy as design - print - cut - shuffle - package, nothing more (+ website)
|
||||
|
||||
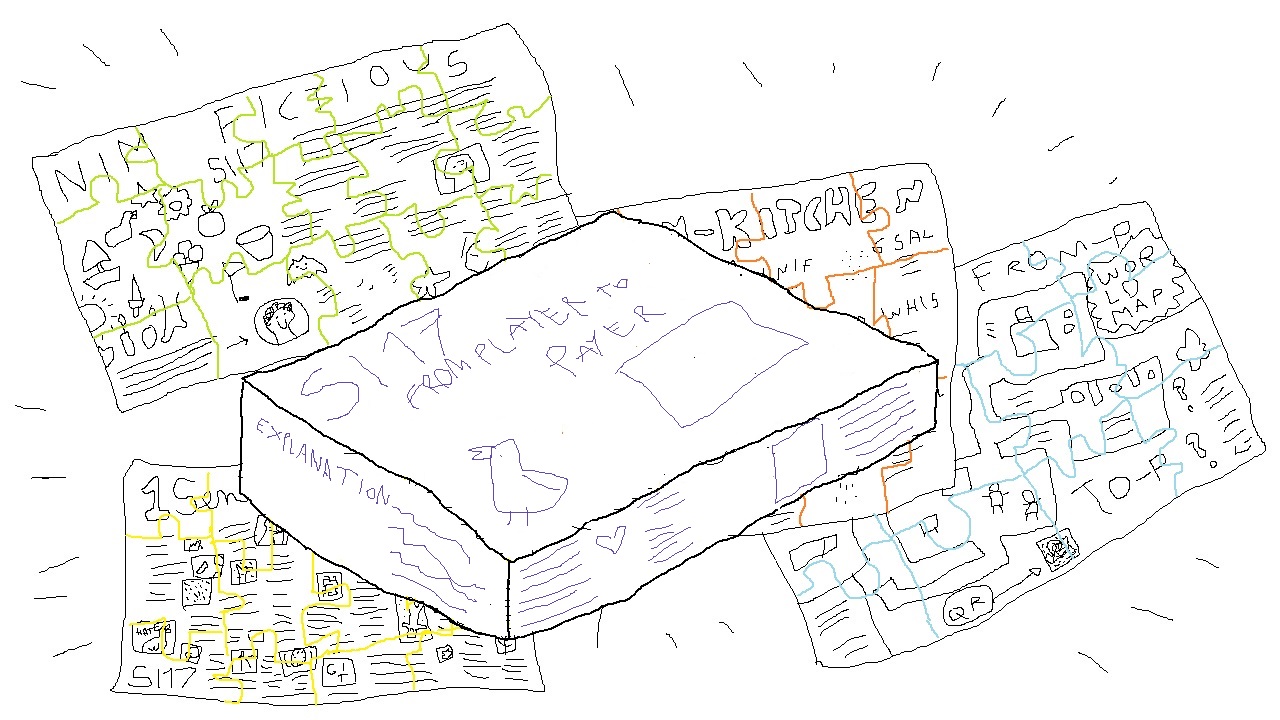
|
||||
The loot box could provide a context and the instruction of the game, as well as the link to the website.
|
||||
|
||||
|
||||
## Scenario
|
||||
|
||||
Mapping the chaotic evil puzzles in the through the different scenari
|
||||
|
||||
### of the form
|
||||
|
||||
_scenario 1: The lootbox is a physical box that contains something_
|
||||
|
||||
Fragments of several puzzles.
|
||||
|
||||
### of the feature
|
||||
|
||||
_scenario 7: The items in the loot-box are complementary and it is necessary to connect with other loot-box owners in order to assemble the pieces together._
|
||||
|
||||
There is a single player aspect and there is a collaborative aspect. These two components could be mediated by an online platform, such as the online shared puzzles, but could also work offline if people just combine the pieces of their loot boxes.
|
||||
|
||||
### of the contents
|
||||
|
||||
_scenario 2: The loot box is a collection of the prototyped games (and researches!) we did so far curated in some kind of form_
|
||||
|
||||
The jigsaw puzzle is just a form of encryption of our contents. A shared surface in which we can publish really different things such as a fan fiction, a board game, a link to a video karaoke or a videogame, an essay, the characters of a roleplaying game, etc.
|
||||
|
||||
_scenario 3: The loot box is a collection of mini-games + an ultimate game that has to be performed with other players that purcahsed the LB_
|
||||
|
||||
There are several layers of playability and access:
|
||||
|
||||
1. to solve the fragments (single player jigsaw)
|
||||
2. to access the contents (games, texts, etc. )
|
||||
3. to combine the fragments (ultimate multiplayer game, re-distribuition of the loot boxes contents)
|
||||
|
||||
|
||||
_scenario 6: The lootbox contains a series of jigsaw puzzles but their pieces are scattered through all the boxes and there is a platform online where you can see the missing tiles._
|
||||
|
||||
Nothing to declare.
|
||||
|
||||
|
||||
## Prototype (look at git!)
|
||||
|
||||
This is a rough prototype for generating ready-to-print jigsaw puzzles as well as a way to track their completion in a shared platform.
|
||||
The idea is to have several puzzles and mix their pieces, in a way that invites the players to collaborate in order to solve them.
|
||||
|
||||
This prototype covers two aspects of the process: the first is to split an image into pieces, tracking every piece with an ID and store the relation between adjacent tiles. The second concerns the online platform, and it is a Flask application that permits to upload cluster of pieces in order to share them with the other players and unlocking the full puzzle.
|
||||
|
||||
### To install the project:
|
||||
|
||||
1. Clone the repo
|
||||
2. Create a virtual environment
|
||||
```
|
||||
$ python3 -m venv venv
|
||||
```
|
||||
3. Activate the virtual environment
|
||||
```
|
||||
$ . venv/bin/activate
|
||||
```
|
||||
4. Install the dependencies
|
||||
```
|
||||
$ pip install -e .
|
||||
```
|
||||
5. Set the environmental variables for flask
|
||||
```
|
||||
$ export FLASK_APP=flaskr
|
||||
$ export FLASK_ENV=development
|
||||
$ flask run
|
||||
```
|
||||
6. The Flask application will be accessible on your browser at `localhost:5000`. If you try to navigate there you will see a blank page. This is because we need to generate some puzzles to display.
|
||||
|
||||
### Generating the contents
|
||||
|
||||
The first thing to do then is to run the `split.py` script:
|
||||
|
||||
```
|
||||
python3 chaospuzzles/split.py
|
||||
```
|
||||
|
||||
This will take the Katamari demo picture from `static/img` and will split it in tiles. The tiles will be used to compose the clusters when a player upload it online. In this way we can be super flexible in the randomization / distribuition of the pieces.
|
||||
|
||||
You can tweak the parameters at the end of the split.py file. This is temporary, later on it would be nice to have an interface to prepare the puzzle.
|
||||
|
||||
### Completing the puzzles
|
||||
|
||||
If you reload the website, you will see a link pointing to the Katamari page. Here we will find an empty frame, and a form to insert new pieces.
|
||||
|
||||
Try it! You can find the IDs for the pieces in the `chaospuzzles/puzzles/katamari/katamari_retro.png` image. This is the picture generated in order to be printed behind the puzzle and let every piece to have a unique ID.
|
||||
|
||||
By design an valid cluster is a group of adjacent pieces.
|
||||
|
||||
Keep in mind that this is a wip and rough prototype so everything need to be polished a lot. We count on your imagination to fill the lack of design, UI and UX here. Imagination is the best modern feature of 2022!
|
||||
|
||||
Thanks and see you sun ☀️
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
## memos
|
||||
|
||||
- play with quantities and distribuition of pieces (1 piece only, large groups, variations, etc)
|
||||
- play with puzzle pattern: alternative to the mainstream shape of the tiles
|
||||
- pieces naming system
|
||||
- And then the aim is to exchange pieces or something and rebuild the original puzzles? (can this be a critical approach?) (does this make sense only if there are as many puzzles as loot boxes?)
|
||||
- short term puzzles (link to multimedia contents, puzzle shards)
|
||||
- long term puzzles (hidden messages, 1 word in each puzzles and a secret sentence)
|
||||
- size and quantity
|
||||
- The jigsaw puzzles results should be secret? There would be much more mistery. Can we reveal only during the launch, but not on the loot boxes? Maybe is a compromise, but maybe is not necessary. Should we reveal them only in the website meanwhile they are completed? Could be.
|
||||
- [Generate Jigsaw Puzzle with python](https://github.com/jkenlooper/piecemaker)
|
||||
- [How To Laser Cut a Jigsaw Puzzle](https://www.youtube.com/watch?v=xqhOrY8unn4)
|
||||
- [Jigsaw puzzle generator](https://draradech.github.io/jigsaw/index.html)
|
||||
- [Fractlal Jigsaw](https://proceduraljigsaw.github.io/Fractalpuzzlejs/)
|
@ -0,0 +1 @@
|
||||
Subproject commit 1c992bfd6afd8a533927a8ba54e678123c175fd8
|
@ -0,0 +1,58 @@
|
||||
---
|
||||
title: Chat Reader
|
||||
description: Transform a text (ok no, actually a CSV file) into a chat
|
||||
date: 06/10/2021
|
||||
project: chat-reader
|
||||
git: https://git.xpub.nl/kamo/chat-reader
|
||||
pad: https://pad.xpub.nl/p/SP16_0510
|
||||
categories:
|
||||
- JS
|
||||
- Chat
|
||||
- Text
|
||||
---
|
||||
|
||||
## Reader Prototyping
|
||||
|
||||
- take suggested methods, use something we already used already - work on it, elaborate, don't exclude what we've been doing with Manetta, Michael and Cristina go in smaller groups/individually and make a prototype - network of texts,
|
||||
- something visual, reworking of something and what it can be a sensible way to explain to people
|
||||
- come together at 15:30? and we share what we've done - talk about how can we stitch it together to make a reader
|
||||
|
||||
## Aggregating different things ~ output: chat form
|
||||
|
||||
### Levels
|
||||
|
||||
- 🏸 1 touch the inputs
|
||||
- 🏸 2 overlap/merge them a bit
|
||||
- 🏸 3 mesh them completely
|
||||
|
||||
### Process
|
||||
|
||||
- 🏏 take an academic text and turn it into a chat - translating into vernacular;
|
||||
- 🏏 simplify the text
|
||||
- 🏏 break it into chats
|
||||
- 🏏 illustrate some bits
|
||||
|
||||
Starting from a difficult but relatable text: our [multi voiced pad](https://pad.xpub.nl/p/SP16_0510) of the day.
|
||||
Parsed here: [Spreadsheet ghost](https://cryptpad.fr/sheet/#/2/sheet/edit/N5uOS8x5Nu28ZiXPSk+kF-um/)
|
||||
|
||||
### Rules to manipulate text:
|
||||
|
||||
- 🏑 table of contents - shorts contents - tag them
|
||||
- 🏑 turn into chat bubbles
|
||||
- 🏑 illustrate a few
|
||||
|
||||
### Rules of text simplification (as ⛳️ objective ⛳️ as possible):
|
||||
|
||||
- 🏓 simple sentences
|
||||
- 🏓 on point
|
||||
- 🏓 short paragraphs and short chapter
|
||||
- 🏓 title on each paragraph
|
||||
- 🏓 text could become image caption/illustrate chapters/graphs?
|
||||
- 🏓 page number
|
||||
- 🏓 navigation (table of contents)
|
||||
|
||||
|
||||
### Demo online: [Chat_a_pad](/soupboat/~kamo/static/html/chat-reader/chat.html)
|
||||
|
||||
### Demo offline:
|
||||
<video src="/soupboat/~kamo/static/video/chat_reader.mp4" autoplay loop></video>
|
@ -0,0 +1,58 @@
|
||||
---
|
||||
title: Chat Reader
|
||||
description: Transform a text (ok no, actually a CSV file) into a chat
|
||||
date: 06/10/2021
|
||||
project: chat-reader
|
||||
git: https://git.xpub.nl/kamo/chat-reader
|
||||
pad: https://pad.xpub.nl/p/SP16_0510
|
||||
categories:
|
||||
- JS
|
||||
- Chat
|
||||
- Text
|
||||
---
|
||||
|
||||
## Reader Prototyping
|
||||
|
||||
- take suggested methods, use something we already used already - work on it, elaborate, don't exclude what we've been doing with Manetta, Michael and Cristina go in smaller groups/individually and make a prototype - network of texts,
|
||||
- something visual, reworking of something and what it can be a sensible way to explain to people
|
||||
- come together at 15:30? and we share what we've done - talk about how can we stitch it together to make a reader
|
||||
|
||||
## Aggregating different things ~ output: chat form
|
||||
|
||||
### Levels
|
||||
|
||||
- 🏸 1 touch the inputs
|
||||
- 🏸 2 overlap/merge them a bit
|
||||
- 🏸 3 mesh them completely
|
||||
|
||||
### Process
|
||||
|
||||
- 🏏 take an academic text and turn it into a chat - translating into vernacular;
|
||||
- 🏏 simplify the text
|
||||
- 🏏 break it into chats
|
||||
- 🏏 illustrate some bits
|
||||
|
||||
Starting from a difficult but relatable text: our [multi voiced pad](https://pad.xpub.nl/p/SP16_0510) of the day.
|
||||
Parsed here: [Spreadsheet ghost](https://cryptpad.fr/sheet/#/2/sheet/edit/N5uOS8x5Nu28ZiXPSk+kF-um/)
|
||||
|
||||
### Rules to manipulate text:
|
||||
|
||||
- 🏑 table of contents - shorts contents - tag them
|
||||
- 🏑 turn into chat bubbles
|
||||
- 🏑 illustrate a few
|
||||
|
||||
### Rules of text simplification (as ⛳️ objective ⛳️ as possible):
|
||||
|
||||
- 🏓 simple sentences
|
||||
- 🏓 on point
|
||||
- 🏓 short paragraphs and short chapter
|
||||
- 🏓 title on each paragraph
|
||||
- 🏓 text could become image caption/illustrate chapters/graphs?
|
||||
- 🏓 page number
|
||||
- 🏓 navigation (table of contents)
|
||||
|
||||
|
||||
### Demo online: [Chat_a_pad](/soupboat/~kamo/static/html/chat-reader/chat.html)
|
||||
|
||||
### Demo offline:
|
||||
<video src="/soupboat/~kamo/static/video/chat_reader.mp4" autoplay loop></video>
|
@ -0,0 +1,51 @@
|
||||
---
|
||||
title: Soupboat CMS 00
|
||||
description: Micro JSON→HTML CMS for the first trimester
|
||||
cover: cms00.jpg
|
||||
cover_alt: Notebook drawing of a dog saying dog and a Microsoft clipper saying pig with ancient hieroglyphs
|
||||
date: 24/12/2021
|
||||
git: https://git.xpub.nl/kamo/kamo-soupbato
|
||||
project: cms00
|
||||
categories:
|
||||
- CMS
|
||||
- JS
|
||||
---
|
||||
|
||||
## A micro CMS
|
||||
|
||||
During the first weeks at XPUB I spent some time trying to figure out how to archive and log the various projects going on. I felt to do it here in the Soupboat, because it's more flexible and playful than the wiki, that remains of course the source of truth and the future-proof archiving system etc. etc. 👹👺
|
||||
|
||||
After the second page though I was already ultra annoyed by the fact of rewriting or copy-pasting the HTML from a page to the other to keep at least a bit of style and structure and add contents manually. I wrote then a bit of code to have a default page and then used a JSON file filled with a list of projects. The script traversed this list and created a table with the basic informations about each one.
|
||||
|
||||
The model for a project was something like that:
|
||||
|
||||
```json
|
||||
{
|
||||
"title": "Text Weaving",
|
||||
"date": "Oct 5, 2021",
|
||||
"url": "10-05-2021-weaving/",
|
||||
"git": "https://git.xpub.nl/kamo/text_weaving",
|
||||
"pad": "https://pad.xpub.nl/p/replacing_cats",
|
||||
"links": [
|
||||
{
|
||||
"url": "",
|
||||
"title": "",
|
||||
}
|
||||
],
|
||||
"categories": [
|
||||
"Python",
|
||||
"NLTK",
|
||||
"Text"
|
||||
]
|
||||
},
|
||||
```
|
||||
|
||||
Each proj has a title, a date, an URL to a dedicated page. Then a list of links: the git repository for sharing the source code and the pad, that are the two most common types of link, and then a list of generic other links, each one composed by an URL and a title. There is also a list of categories, in order to give some hints about the project.
|
||||
|
||||
The dedicated page for a project could have been something somewhere in the Soupboat, or a subfolder in my personal folder.
|
||||
|
||||
The structure of the whole thing was: an `index.html` page with a `cms.js` script and a `cms.json` file. (Such imagination in these filenames). Then a `style.css` and a `global.css` for sharing the style with the various projects.
|
||||
|
||||
Not really a revolutionary CMS but a starting point. Ah ah
|
||||
|
||||
I'm writing this while im migrating everything into a flask based one, that will use more or less the same structure we developed for the SI16! Really happy with it. Good night
|
@ -0,0 +1,51 @@
|
||||
---
|
||||
title: Soupboat CMS 00
|
||||
description: Micro JSON→HTML CMS for the first trimester
|
||||
cover: cms00.jpg
|
||||
cover_alt: Notebook drawing of a dog saying dog and a Microsoft clipper saying pig with ancient hieroglyphs
|
||||
date: 24/12/2021
|
||||
git: https://git.xpub.nl/kamo/kamo-soupbato
|
||||
project: cms00
|
||||
categories:
|
||||
- CMS
|
||||
- JS
|
||||
---
|
||||
|
||||
## A micro CMS
|
||||
|
||||
During the first weeks at XPUB I spent some time trying to figure out how to archive and log the various projects going on. I felt to do it here in the Soupboat, because it's more flexible and playful than the wiki, that remains of course the source of truth and the future-proof archiving system etc. etc. 👹👺
|
||||
|
||||
After the second page though I was already ultra annoyed by the fact of rewriting or copy-pasting the HTML from a page to the other to keep at least a bit of style and structure and add contents manually. I wrote then a bit of code to have a default page and then used a JSON file filled with a list of projects. The script traversed this list and created a table with the basic informations about each one.
|
||||
|
||||
The model for a project was something like that:
|
||||
|
||||
```json
|
||||
{
|
||||
"title": "Text Weaving",
|
||||
"date": "Oct 5, 2021",
|
||||
"url": "10-05-2021-weaving/",
|
||||
"git": "https://git.xpub.nl/kamo/text_weaving",
|
||||
"pad": "https://pad.xpub.nl/p/replacing_cats",
|
||||
"links": [
|
||||
{
|
||||
"url": "",
|
||||
"title": "",
|
||||
}
|
||||
],
|
||||
"categories": [
|
||||
"Python",
|
||||
"NLTK",
|
||||
"Text"
|
||||
]
|
||||
},
|
||||
```
|
||||
|
||||
Each proj has a title, a date, an URL to a dedicated page. Then a list of links: the git repository for sharing the source code and the pad, that are the two most common types of link, and then a list of generic other links, each one composed by an URL and a title. There is also a list of categories, in order to give some hints about the project.
|
||||
|
||||
The dedicated page for a project could have been something somewhere in the Soupboat, or a subfolder in my personal folder.
|
||||
|
||||
The structure of the whole thing was: an `index.html` page with a `cms.js` script and a `cms.json` file. (Such imagination in these filenames). Then a `style.css` and a `global.css` for sharing the style with the various projects.
|
||||
|
||||
Not really a revolutionary CMS but a starting point. Ah ah
|
||||
|
||||
I'm writing this while im migrating everything into a flask based one, that will use more or less the same structure we developed for the SI16! Really happy with it. Good night
|
@ -0,0 +1,21 @@
|
||||
---
|
||||
title: Concrete 🎏 Label
|
||||
date: 01/11/2021
|
||||
description: A tool for annotating visual contents
|
||||
git: https://git.xpub.nl/kamo/concrete-label
|
||||
pad: https://pad.xpub.nl/p/AGAINST_FILTERING
|
||||
categories:
|
||||
- Web
|
||||
- Text
|
||||
- Label
|
||||
- Tool
|
||||
---
|
||||
|
||||
|
||||
How could computer read concrete & visual poetry? How does computer navigate through text objects in which layout and graphical elements play a fundamental role?
|
||||
|
||||
With this tool you can upload an image and then annotate it spatially. In doing so you generate a transcription of the image that keeps track of the order of your annotations (and so the visual path you take when reading the image), as well as their position and size.
|
||||
|
||||
Neither the image nor the labels nor the transcription will be uploaded online. Everything happen in your browser.
|
||||
|
||||
__a join research with Supi 👹👺__
|
@ -0,0 +1,21 @@
|
||||
---
|
||||
title: Concrete 🎏 Label
|
||||
date: 01/11/2021
|
||||
description: A tool for annotating visual contents
|
||||
git: https://git.xpub.nl/kamo/concrete-label
|
||||
pad: https://pad.xpub.nl/p/AGAINST_FILTERING
|
||||
categories:
|
||||
- Web
|
||||
- Text
|
||||
- Label
|
||||
- Tool
|
||||
---
|
||||
|
||||
|
||||
How could computer read concrete & visual poetry? How does computer navigate through text objects in which layout and graphical elements play a fundamental role?
|
||||
|
||||
With this tool you can upload an image and then annotate it spatially. In doing so you generate a transcription of the image that keeps track of the order of your annotations (and so the visual path you take when reading the image), as well as their position and size.
|
||||
|
||||
Neither the image nor the labels nor the transcription will be uploaded online. Everything happen in your browser.
|
||||
|
||||
__a join research with Supi 👹👺__
|
@ -0,0 +1,76 @@
|
||||
---
|
||||
title: 🎵 K-PUB
|
||||
date: 21/10/2021
|
||||
url: /soupboat/k-pub/
|
||||
description: Karaoke as a mean of republishing
|
||||
git: https://git.xpub.nl/grgr/k-pub
|
||||
categories:
|
||||
- Event
|
||||
- vvvv
|
||||
- Text
|
||||
- Long Term
|
||||
---
|
||||
|
||||
## Karaoke as a mean of republishing
|
||||
|
||||
The idea is easy: take some songs, take some texts, and merge them through the logic of karaoke.
|
||||
For our first issue we want to work with Simon Weil's diaries as text material and Franco Battiato's songs as musical starting point.
|
||||
|
||||
Using a popular and performative device such as karaoke we want to join different voices. Not only the ones from the people singing, but also from all the different authors that participate in this event: the writer of texts, the composer of musical bases and the musicians that will perform them.
|
||||
This project started as a joke and eventually growed because we saw a lot of potential in it.
|
||||
|
||||

|
||||
|
||||
## Christmas Update
|
||||
|
||||
Ok we got the room of the little Room for Sound at WdkA: nice.
|
||||
So here is a list of things we need and a list of things to do:
|
||||
|
||||
### TODO:
|
||||
|
||||
- text from simone weil
|
||||
- select excerpts
|
||||
- excerpts to lyrics
|
||||
- audio from franco battiato
|
||||
- select songs
|
||||
- find or write midi files
|
||||
- sound design
|
||||
- performance mode
|
||||
- visual
|
||||
- finish setup record mode (excerpts → lyrics)
|
||||
- setup playback mode
|
||||
- design
|
||||
- development
|
||||
- performance
|
||||
- call musicians
|
||||
- space setup
|
||||
- technical setup
|
||||
- comunication
|
||||
- documentation
|
||||
- pubblication
|
||||
- residency
|
||||
- daily contents to be published on their radio (readings, log, musical experiemnts...)
|
||||
|
||||
### workflow for 1 song:
|
||||
|
||||
1. select text excerpts
|
||||
2. select song
|
||||
3. song to midi
|
||||
1. if there is already a midi: cleanup: split and join tracks meaningfully
|
||||
2. if not: song transcription
|
||||
4. karaoke recording
|
||||
1. input: midi song, text excerpts
|
||||
2. process: performative conversion, excerpt to lyrics tool
|
||||
3. output: karaoke midi song with text track
|
||||
5. karaoke performance
|
||||
1. input: karaoke midi song
|
||||
2. output: karaoke text, karaoke midi
|
||||
1. midi → text, display the text for singin along
|
||||
2. midi → audio, for live playing and real time sound design of the song
|
||||
3. midi → visual, for live visual effects
|
||||
|
||||
### people we need:
|
||||
- musician (at least 1 to start with) (micalis? gambas? others?)
|
||||
- visual (open call? or we can do it on our own for this)
|
||||
- event logic & logistic (chae? gersande? etc? if anyone wants to take care of the setup it would be super cool)
|
||||
- documentation (pinto? carmen? etc?)
|
@ -0,0 +1,76 @@
|
||||
---
|
||||
title: 🎵 K-PUB
|
||||
date: 21/10/2021
|
||||
url: /soupboat/k-pub/
|
||||
description: Karaoke as a mean of republishing
|
||||
git: https://git.xpub.nl/grgr/k-pub
|
||||
categories:
|
||||
- Event
|
||||
- vvvv
|
||||
- Text
|
||||
- Long Term
|
||||
---
|
||||
|
||||
## Karaoke as a mean of republishing
|
||||
|
||||
The idea is easy: take some songs, take some texts, and merge them through the logic of karaoke.
|
||||
For our first issue we want to work with Simon Weil's diaries as text material and Franco Battiato's songs as musical starting point.
|
||||
|
||||
Using a popular and performative device such as karaoke we want to join different voices. Not only the ones from the people singing, but also from all the different authors that participate in this event: the writer of texts, the composer of musical bases and the musicians that will perform them.
|
||||
This project started as a joke and eventually growed because we saw a lot of potential in it.
|
||||
|
||||

|
||||
|
||||
## Christmas Update
|
||||
|
||||
Ok we got the room of the little Room for Sound at WdkA: nice.
|
||||
So here is a list of things we need and a list of things to do:
|
||||
|
||||
### TODO:
|
||||
|
||||
- text from simone weil
|
||||
- select excerpts
|
||||
- excerpts to lyrics
|
||||
- audio from franco battiato
|
||||
- select songs
|
||||
- find or write midi files
|
||||
- sound design
|
||||
- performance mode
|
||||
- visual
|
||||
- finish setup record mode (excerpts → lyrics)
|
||||
- setup playback mode
|
||||
- design
|
||||
- development
|
||||
- performance
|
||||
- call musicians
|
||||
- space setup
|
||||
- technical setup
|
||||
- comunication
|
||||
- documentation
|
||||
- pubblication
|
||||
- residency
|
||||
- daily contents to be published on their radio (readings, log, musical experiemnts...)
|
||||
|
||||
### workflow for 1 song:
|
||||
|
||||
1. select text excerpts
|
||||
2. select song
|
||||
3. song to midi
|
||||
1. if there is already a midi: cleanup: split and join tracks meaningfully
|
||||
2. if not: song transcription
|
||||
4. karaoke recording
|
||||
1. input: midi song, text excerpts
|
||||
2. process: performative conversion, excerpt to lyrics tool
|
||||
3. output: karaoke midi song with text track
|
||||
5. karaoke performance
|
||||
1. input: karaoke midi song
|
||||
2. output: karaoke text, karaoke midi
|
||||
1. midi → text, display the text for singin along
|
||||
2. midi → audio, for live playing and real time sound design of the song
|
||||
3. midi → visual, for live visual effects
|
||||
|
||||
### people we need:
|
||||
- musician (at least 1 to start with) (micalis? gambas? others?)
|
||||
- visual (open call? or we can do it on our own for this)
|
||||
- event logic & logistic (chae? gersande? etc? if anyone wants to take care of the setup it would be super cool)
|
||||
- documentation (pinto? carmen? etc?)
|
@ -0,0 +1,24 @@
|
||||
---
|
||||
title: A Katamari Fanfiction
|
||||
description: What's left when you roll on everything?
|
||||
date: 09/02/2022
|
||||
pad: https://pad.xpub.nl/p/katamari-fanfiction
|
||||
link:
|
||||
- title: Ideology
|
||||
url: https://pad.xpub.nl/p/katamari-damacy
|
||||
project: katamari
|
||||
cover: frog.png
|
||||
cover_alt: 3d frog from katamari
|
||||
categories:
|
||||
- SI17
|
||||
- Games
|
||||
- Text
|
||||
---
|
||||
|
||||
## Modding narrative
|
||||
|
||||
`After lunch we will be writing fanfic based on the games you played and analysed this week!` Lidia said this.
|
||||
|
||||
We played Katamari Damacy this week → We wrote a fan faction about it → We approached the fanfiction with empathyzing with the most inanimate things of the game → So we focused on the prince and the objects of the game → We left the King of all Cosmos in the backgroud, since he talks already a lot in the original game. → Suggested soundtrack for the reading: [katamari OST](https://www.youtube.com/watch?v=QAA6hq9RL-4)
|
||||
|
||||
Fun fictious with Mitsa and Erica
|
@ -0,0 +1,79 @@
|
||||
{
|
||||
"cells": [
|
||||
{
|
||||
"cell_type": "code",
|
||||
"execution_count": 7,
|
||||
"id": "ba7a2054-5b27-46e7-aad7-14d0fe1d7a00",
|
||||
"metadata": {},
|
||||
"outputs": [
|
||||
{
|
||||
"name": "stdout",
|
||||
"output_type": "stream",
|
||||
"text": [
|
||||
"hello book \n",
|
||||
"hello book hello shelves \n",
|
||||
"hello book hello shelves hello supermarket \n",
|
||||
"hello book hello shelves hello supermarket hello street \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine hello mineral \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine hello mineral hello anti men mine \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine hello mineral hello anti men mine hello gun \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine hello mineral hello anti men mine hello gun hello killer \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine hello mineral hello anti men mine hello gun hello killer hello kinder \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine hello mineral hello anti men mine hello gun hello killer hello kinder hello children \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine hello mineral hello anti men mine hello gun hello killer hello kinder hello children hello \n"
|
||||
]
|
||||
}
|
||||
],
|
||||
"source": [
|
||||
"text = \"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine hello mineral hello anti men mine hello gun hello killer hello kinder hello children hello\"\n",
|
||||
"things = text.split('hello')\n",
|
||||
"output = ''\n",
|
||||
"for thing in things[1:]:\n",
|
||||
" output = output + f'hello {thing.strip()} '\n",
|
||||
" print(output)"
|
||||
]
|
||||
},
|
||||
{
|
||||
"cell_type": "code",
|
||||
"execution_count": null,
|
||||
"id": "35b17264-b530-4a19-ad5d-e3027fcee1dd",
|
||||
"metadata": {},
|
||||
"outputs": [],
|
||||
"source": []
|
||||
}
|
||||
],
|
||||
"metadata": {
|
||||
"kernelspec": {
|
||||
"display_name": "Python 3 (ipykernel)",
|
||||
"language": "python",
|
||||
"name": "python3"
|
||||
},
|
||||
"language_info": {
|
||||
"codemirror_mode": {
|
||||
"name": "ipython",
|
||||
"version": 3
|
||||
},
|
||||
"file_extension": ".py",
|
||||
"mimetype": "text/x-python",
|
||||
"name": "python",
|
||||
"nbconvert_exporter": "python",
|
||||
"pygments_lexer": "ipython3",
|
||||
"version": "3.7.3"
|
||||
}
|
||||
},
|
||||
"nbformat": 4,
|
||||
"nbformat_minor": 5
|
||||
}
|
@ -0,0 +1,24 @@
|
||||
---
|
||||
title: A Katamari Fanfiction
|
||||
description: What's left when you roll on everything?
|
||||
date: 09/02/2022
|
||||
pad: https://pad.xpub.nl/p/katamari-fanfiction
|
||||
link:
|
||||
- title: Ideology
|
||||
url: https://pad.xpub.nl/p/katamari-damacy
|
||||
project: katamari
|
||||
cover: frog.png
|
||||
cover_alt: 3d frog from katamari
|
||||
categories:
|
||||
- SI17
|
||||
- Games
|
||||
- Text
|
||||
---
|
||||
|
||||
## Modding narrative
|
||||
|
||||
`After lunch we will be writing fanfic based on the games you played and analysed this week!` Lidia said this.
|
||||
|
||||
We played Katamari Damacy this week → We wrote a fan faction about it → We approached the fanfiction with empathyzing with the most inanimate things of the game → So we focused on the prince and the objects of the game → We left the King of all Cosmos in the backgroud, since he talks already a lot in the original game. → Suggested soundtrack for the reading: [katamari OST](https://www.youtube.com/watch?v=QAA6hq9RL-4)
|
||||
|
||||
Fun fictious with Mitsa and Erica
|
@ -0,0 +1,218 @@
|
||||
{
|
||||
"cells": [
|
||||
{
|
||||
"cell_type": "code",
|
||||
"execution_count": 8,
|
||||
"id": "ba7a2054-5b27-46e7-aad7-14d0fe1d7a00",
|
||||
"metadata": {},
|
||||
"outputs": [],
|
||||
"source": [
|
||||
"def katamari(text, separator='hello'):\n",
|
||||
" things = text.split(separator)\n",
|
||||
" output = ''\n",
|
||||
" for thing in things[1:]:\n",
|
||||
" output = output + f'hello {thing.strip()} '\n",
|
||||
" print(output)"
|
||||
]
|
||||
},
|
||||
{
|
||||
"cell_type": "code",
|
||||
"execution_count": 9,
|
||||
"id": "35b17264-b530-4a19-ad5d-e3027fcee1dd",
|
||||
"metadata": {},
|
||||
"outputs": [
|
||||
{
|
||||
"name": "stdout",
|
||||
"output_type": "stream",
|
||||
"text": [
|
||||
"hello moon \n",
|
||||
"hello moon hello earth \n",
|
||||
"hello moon hello earth hello rings of saturn \n",
|
||||
"hello moon hello earth hello rings of saturn hello orbital elements \n",
|
||||
"hello moon hello earth hello rings of saturn hello orbital elements hello fixed stars \n",
|
||||
"hello moon hello earth hello rings of saturn hello orbital elements hello fixed stars hello milky way \n",
|
||||
"hello moon hello earth hello rings of saturn hello orbital elements hello fixed stars hello milky way hello popping stars \n",
|
||||
"hello moon hello earth hello rings of saturn hello orbital elements hello fixed stars hello milky way hello popping stars hello shooting stars \n",
|
||||
"hello moon hello earth hello rings of saturn hello orbital elements hello fixed stars hello milky way hello popping stars hello shooting stars hello soundtrack \n",
|
||||
"hello moon hello earth hello rings of saturn hello orbital elements hello fixed stars hello milky way hello popping stars hello shooting stars hello soundtrack hello marimba \n",
|
||||
"hello moon hello earth hello rings of saturn hello orbital elements hello fixed stars hello milky way hello popping stars hello shooting stars hello soundtrack hello marimba hello calimba \n",
|
||||
"hello moon hello earth hello rings of saturn hello orbital elements hello fixed stars hello milky way hello popping stars hello shooting stars hello soundtrack hello marimba hello calimba hello mazinga \n"
|
||||
]
|
||||
}
|
||||
],
|
||||
"source": [
|
||||
"katamari('hello moon hello earth hello rings of saturn hello orbital elements hello fixed stars hello milky way hello popping stars hello shooting stars hello soundtrack hello marimba hello calimba hello mazinga')"
|
||||
]
|
||||
},
|
||||
{
|
||||
"cell_type": "code",
|
||||
"execution_count": 10,
|
||||
"id": "1752882a-1226-4408-9deb-fc2e31321412",
|
||||
"metadata": {},
|
||||
"outputs": [
|
||||
{
|
||||
"name": "stdout",
|
||||
"output_type": "stream",
|
||||
"text": [
|
||||
"hello book \n",
|
||||
"hello book hello shelves \n",
|
||||
"hello book hello shelves hello supermarket \n",
|
||||
"hello book hello shelves hello supermarket hello street \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine hello mineral \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine hello mineral hello anti men mine \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine hello mineral hello anti men mine hello gun \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine hello mineral hello anti men mine hello gun hello killer \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine hello mineral hello anti men mine hello gun hello killer hello kinder \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine hello mineral hello anti men mine hello gun hello killer hello kinder hello children \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine hello mineral hello anti men mine hello gun hello killer hello kinder hello children hello \n"
|
||||
]
|
||||
}
|
||||
],
|
||||
"source": [
|
||||
"katamari('hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine hello mineral hello anti men mine hello gun hello killer hello kinder hello children hello')"
|
||||
]
|
||||
},
|
||||
{
|
||||
"cell_type": "code",
|
||||
"execution_count": 11,
|
||||
"id": "97c2a743-263b-49da-9632-70e80918e72d",
|
||||
"metadata": {},
|
||||
"outputs": [
|
||||
{
|
||||
"name": "stdout",
|
||||
"output_type": "stream",
|
||||
"text": [
|
||||
"hello king \n",
|
||||
"hello king hello queen \n",
|
||||
"hello king hello queen hello prince \n",
|
||||
"hello king hello queen hello prince hello \n"
|
||||
]
|
||||
}
|
||||
],
|
||||
"source": [
|
||||
"katamari('hello king hello queen hello prince hello')"
|
||||
]
|
||||
},
|
||||
{
|
||||
"cell_type": "code",
|
||||
"execution_count": 12,
|
||||
"id": "d0b7860a-e65b-4f5a-b532-e61df1145742",
|
||||
"metadata": {},
|
||||
"outputs": [
|
||||
{
|
||||
"name": "stdout",
|
||||
"output_type": "stream",
|
||||
"text": [
|
||||
"hello frog \n",
|
||||
"hello frog hello giraffe \n",
|
||||
"hello frog hello giraffe hello lipstick \n",
|
||||
"hello frog hello giraffe hello lipstick hello rubber \n",
|
||||
"hello frog hello giraffe hello lipstick hello rubber hello beaver \n",
|
||||
"hello frog hello giraffe hello lipstick hello rubber hello beaver hello flower \n",
|
||||
"hello frog hello giraffe hello lipstick hello rubber hello beaver hello flower hello candy \n",
|
||||
"hello frog hello giraffe hello lipstick hello rubber hello beaver hello flower hello candy hello treasure \n",
|
||||
"hello frog hello giraffe hello lipstick hello rubber hello beaver hello flower hello candy hello treasure hello television \n",
|
||||
"hello frog hello giraffe hello lipstick hello rubber hello beaver hello flower hello candy hello treasure hello television hello window \n",
|
||||
"hello frog hello giraffe hello lipstick hello rubber hello beaver hello flower hello candy hello treasure hello television hello window hello chair \n",
|
||||
"hello frog hello giraffe hello lipstick hello rubber hello beaver hello flower hello candy hello treasure hello television hello window hello chair hello fire extinguisher \n",
|
||||
"hello frog hello giraffe hello lipstick hello rubber hello beaver hello flower hello candy hello treasure hello television hello window hello chair hello fire extinguisher hello carpet \n",
|
||||
"hello frog hello giraffe hello lipstick hello rubber hello beaver hello flower hello candy hello treasure hello television hello window hello chair hello fire extinguisher hello carpet hello grass \n",
|
||||
"hello frog hello giraffe hello lipstick hello rubber hello beaver hello flower hello candy hello treasure hello television hello window hello chair hello fire extinguisher hello carpet hello grass hello cigarette \n",
|
||||
"hello frog hello giraffe hello lipstick hello rubber hello beaver hello flower hello candy hello treasure hello television hello window hello chair hello fire extinguisher hello carpet hello grass hello cigarette hello mouse \n",
|
||||
"hello frog hello giraffe hello lipstick hello rubber hello beaver hello flower hello candy hello treasure hello television hello window hello chair hello fire extinguisher hello carpet hello grass hello cigarette hello mouse hello mice \n",
|
||||
"hello frog hello giraffe hello lipstick hello rubber hello beaver hello flower hello candy hello treasure hello television hello window hello chair hello fire extinguisher hello carpet hello grass hello cigarette hello mouse hello mice hello nietche \n",
|
||||
"hello frog hello giraffe hello lipstick hello rubber hello beaver hello flower hello candy hello treasure hello television hello window hello chair hello fire extinguisher hello carpet hello grass hello cigarette hello mouse hello mice hello nietche hello beach \n",
|
||||
"hello frog hello giraffe hello lipstick hello rubber hello beaver hello flower hello candy hello treasure hello television hello window hello chair hello fire extinguisher hello carpet hello grass hello cigarette hello mouse hello mice hello nietche hello beach hello whale \n",
|
||||
"hello frog hello giraffe hello lipstick hello rubber hello beaver hello flower hello candy hello treasure hello television hello window hello chair hello fire extinguisher hello carpet hello grass hello cigarette hello mouse hello mice hello nietche hello beach hello whale hello waves \n"
|
||||
]
|
||||
}
|
||||
],
|
||||
"source": [
|
||||
"katamari('hello frog hello giraffe hello lipstick hello rubber hello beaver hello flower hello candy hello treasure hello television hello window hello chair hello fire extinguisher hello carpet hello grass hello cigarette hello mouse hello mice hello nietche hello beach hello whale hello waves')"
|
||||
]
|
||||
},
|
||||
{
|
||||
"cell_type": "code",
|
||||
"execution_count": 13,
|
||||
"id": "5e0d5cfb-a9df-4337-ac4c-523d93250841",
|
||||
"metadata": {},
|
||||
"outputs": [
|
||||
{
|
||||
"name": "stdout",
|
||||
"output_type": "stream",
|
||||
"text": [
|
||||
"hello book \n",
|
||||
"hello book hello shelves \n",
|
||||
"hello book hello shelves hello supermarket \n",
|
||||
"hello book hello shelves hello supermarket hello street \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine hello mineral \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine hello mineral hello anti men mine \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine hello mineral hello anti men mine hello gun \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine hello mineral hello anti men mine hello gun hello killer \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine hello mineral hello anti men mine hello gun hello killer hello kinder \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine hello mineral hello anti men mine hello gun hello killer hello kinder hello children \n",
|
||||
"hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine hello mineral hello anti men mine hello gun hello killer hello kinder hello children hello \n"
|
||||
]
|
||||
}
|
||||
],
|
||||
"source": [
|
||||
"katamari('hello book hello shelves hello supermarket hello street hello car hello ceo of the city public transportation system hello playstation hello carpet hello giraffe hello frog hello ball hello dust hello microbs hello crown hello corona hello clown hello mine hello mineral hello anti men mine hello gun hello killer hello kinder hello children hello')"
|
||||
]
|
||||
},
|
||||
{
|
||||
"cell_type": "code",
|
||||
"execution_count": null,
|
||||
"id": "825a8878-cbcf-4cfa-b7e3-fa9ed0cd71fc",
|
||||
"metadata": {},
|
||||
"outputs": [],
|
||||
"source": []
|
||||
}
|
||||
],
|
||||
"metadata": {
|
||||
"kernelspec": {
|
||||
"display_name": "Python 3 (ipykernel)",
|
||||
"language": "python",
|
||||
"name": "python3"
|
||||
},
|
||||
"language_info": {
|
||||
"codemirror_mode": {
|
||||
"name": "ipython",
|
||||
"version": 3
|
||||
},
|
||||
"file_extension": ".py",
|
||||
"mimetype": "text/x-python",
|
||||
"name": "python",
|
||||
"nbconvert_exporter": "python",
|
||||
"pygments_lexer": "ipython3",
|
||||
"version": "3.7.3"
|
||||
}
|
||||
},
|
||||
"nbformat": 4,
|
||||
"nbformat_minor": 5
|
||||
}
|
@ -0,0 +1,27 @@
|
||||
---
|
||||
title: Text ⛵ Lifeboats
|
||||
description: What if we could use some excerpts from all of what we are reading now as lifeboats in a sea of text?
|
||||
date: 28/09/2021
|
||||
project: lifeboats
|
||||
pad: https://pad.xpub.nl/p/collab_week3
|
||||
categories:
|
||||
- JS
|
||||
- Text
|
||||
---
|
||||
|
||||
## Text Traversing
|
||||
|
||||
- Which texts will you traverse? will you make a "quote landscape" from
|
||||
the different texts brought today or stay with one single text?
|
||||
- Identify patterns / gather observations / draw the relations between the
|
||||
words/paragraphs/sounds
|
||||
- What are markers of orientation you would like to set for this text?
|
||||
- Where should the reader turn?
|
||||
- What are the rhythms in the text and how can they be amplified/interrupted/multiplied?
|
||||
- Make a score or a diagram or a script to be performed out loud
|
||||
|
||||
## Process
|
||||
|
||||
What if we could use some excerpts from all of what we are reading now as lifeboats
|
||||
in a sea of text? An attempt to play around with the continous permutations between
|
||||
contents and contexts.
|
@ -0,0 +1,27 @@
|
||||
---
|
||||
title: Text ⛵ Lifeboats
|
||||
description: What if we could use some excerpts from all of what we are reading now as lifeboats in a sea of text?
|
||||
date: 28/09/2021
|
||||
project: lifeboats
|
||||
pad: https://pad.xpub.nl/p/collab_week3
|
||||
categories:
|
||||
- JS
|
||||
- Text
|
||||
---
|
||||
|
||||
## Text Traversing
|
||||
|
||||
- Which texts will you traverse? will you make a "quote landscape" from
|
||||
the different texts brought today or stay with one single text?
|
||||
- Identify patterns / gather observations / draw the relations between the
|
||||
words/paragraphs/sounds
|
||||
- What are markers of orientation you would like to set for this text?
|
||||
- Where should the reader turn?
|
||||
- What are the rhythms in the text and how can they be amplified/interrupted/multiplied?
|
||||
- Make a score or a diagram or a script to be performed out loud
|
||||
|
||||
## Process
|
||||
|
||||
What if we could use some excerpts from all of what we are reading now as lifeboats
|
||||
in a sea of text? An attempt to play around with the continous permutations between
|
||||
contents and contexts.
|
@ -0,0 +1,24 @@
|
||||
---
|
||||
title: Deciduous Time Everlasting Internet
|
||||
description: Design the lifespan of a website
|
||||
date: 01/04/2022
|
||||
categories:
|
||||
- Workshop
|
||||
- Web
|
||||
url: https://hub.xpub.nl/soupboat/constr/lifespan/
|
||||
cover: clock.jpg
|
||||
cover_alt: reflected clock in the xpub studio
|
||||
---
|
||||
|
||||
## Design with constraints
|
||||
|
||||
Together with Carmen we approached the workshop of [Raphaël Bastide](https://raphaelbastide.com/) working on the lifespan of a website. The idea was to create a website that disappears, an online space that the user can visit only once. Scarcity against the refresh.
|
||||
|
||||
We used some pictures of clocks as main contents. I wrote a [simple tool](https://hub.xpub.nl/soupboat/constr/lifespan/transform.html) to help us centering the photos on the center of each clock in the web page. Then we stacked every image on top of each other. This pile of clocks disappears in front of the user, almost exploding at the end.
|
||||
|
||||
Then the website it's gone.
|
||||
Lifspan: 10s on each browser.
|
||||
It was nice until it lasted.
|
||||
|
||||
See the other super nice results from the workshop [here](https://hub.xpub.nl/soupboat/constr/)
|
||||
|
@ -0,0 +1,24 @@
|
||||
---
|
||||
title: Deciduous Time Everlasting Internet
|
||||
description: Design the lifespan of a website
|
||||
date: 01/04/2022
|
||||
categories:
|
||||
- Workshop
|
||||
- Web
|
||||
url: https://hub.xpub.nl/soupboat/constr/lifespan/
|
||||
cover: clock.jpg
|
||||
cover_alt: reflected clock in the xpub studio
|
||||
---
|
||||
|
||||
## Design with constraints
|
||||
|
||||
Together with Carmen we approached the workshop of [Raphaël Bastide](https://raphaelbastide.com/) working on the lifespan of a website. The idea was to create a website that disappears, an online space that the user can visit only once. Scarcity against the refresh.
|
||||
|
||||
We used some pictures of clocks as main contents. I wrote a [simple tool](https://hub.xpub.nl/soupboat/constr/lifespan/transform.html) to help us centering the photos on the center of each clock in the web page. Then we stacked every image on top of each other. This pile of clocks disappears in front of the user, almost exploding at the end.
|
||||
|
||||
Then the website it's gone.
|
||||
Lifspan: 10s on each browser.
|
||||
It was nice until it lasted.
|
||||
|
||||
See the other super nice results from the workshop [here](https://hub.xpub.nl/soupboat/constr/)
|
||||
|
@ -0,0 +1,107 @@
|
||||
---
|
||||
title: Loot Box as a Decorator
|
||||
date: 11/02/2022
|
||||
description: Hermit crab in the book store
|
||||
cover: 080.png
|
||||
cover_alt: A Slowbro, notoriously slow pokemon
|
||||
categories:
|
||||
- SI17
|
||||
- Games
|
||||
- Research
|
||||
---
|
||||
|
||||
## 3 intuitions come together
|
||||
|
||||
Right now:
|
||||
|
||||
1. A _loot box_ within a context as such: a book store
|
||||
2. A _loot box_ within a temporality
|
||||
3. A _loot box_ with different kinds of public
|
||||
|
||||
Over me 🦶🦶 🥁 🦵🦵 📀----
|
||||
|
||||
|
||||
|
||||
### Context
|
||||
|
||||
A _lolt box_ accellerates and forces the mechanics of an environment. In some games it can speed up some tedious process, in other it offers a specific special instant rewarding. Our _loot bbx_ inhabits a book store, or more in general a cultural space. In which ways can we hack through the normal functioning of such place? At a certain point today I thought: _ah, we could fill it with the last page of every books in Page Not Found_, just to say something about the presumed shortcuts that the _loat box_ promises to the player. The idea is kinda fun, but then what? So maybe no.
|
||||
|
||||
### Temporality
|
||||
|
||||
A couple of days ago I wrote some notes about the temporality of the _loto box_. In 1 sentence the idea is: if the _lot bx_ is a mechanism of instant rewarding, we could hijack and inflate its tempo and then fill it with our contents. Instead of opening in 30 seconds, the _loot bocs_ takes one hour. Meanwhile we can deliver our messages.
|
||||
|
||||
Today I read _Play like a feminist_ by _Shira Chess_ and guess what: there's an entire part about the temporality of leisure → 🤯
|
||||
|
||||
There is something really important we should keep in mind: we are aiming to a public that is etherogeneous. The intersectional approach that Chess advocates it's a reminder that we can inflate the temporality of the _loot biusch_, but not everyone will have access to it. So we need to think at both the limits of this spectrum, and put them in a meaningful relation.
|
||||
|
||||
### Public
|
||||
|
||||
As said: our public could be complex. For sure there will be some ultra publishing nerd that will sip all our soup and will be happy with it, but isn't 1 of our goals to reach also the world outside XPUB? _Chess_ in her book writes about micro temporality, little timespans carved between work shifts or commutes. She has a point when writes that with smartphones leisure time is more affordable and is detached from the rigid tempo of labour.
|
||||
|
||||
## Decorator
|
||||
|
||||
Combining these three aspects the question is: can we create a relation between who can spend an hour at PNF waiting for the _loot bosx_ and who cannot?
|
||||
|
||||
Enter the _boot lox_ as a decorator.
|
||||
|
||||
A decorator is something that adorn something else. In Python and object-oriented programming in general is also a name of a design pattern that adds some functionality to other functions. We used it already also with Flask! A _oobt olx_ as a decorator means that we could attach it to other pubblication at PNF. Something like an hermit crab inside other shells or that spiky things that bites the tail of a Slowpoke.
|
||||
|
||||
|
||||
### The setup
|
||||
|
||||
1. The physical decorator, that is a digital manufactured object produced on demand
|
||||
2. A catalogue of books that can be decorated
|
||||
3. A website with a digital loot box
|
||||
|
||||
### The process
|
||||
|
||||
1. As a part of the research we compose a bibliography that is also a statement i.e: _away from the cis white west guys gang_. This bibliography could be site specific for PNF or the other places we will distribute our SI17. We should choose to sell our pubblication in book stores or spaces that want to host this bibliography in their inventory. In this way we can use our SI17 as a device to reclaim space for marginal and subaltern voices.
|
||||
|
||||
2. The decorator inhabits this bibliography. It is presented as a special offer in which you can buy one of the book from the bibliography and receive a decorated version of it. Maybe we can sort out some kind of discount mechanism using part of the budget we have. The point is to favor access.
|
||||
|
||||
3. The deal is that the production of the decorator has a certain temporality: if we imagine it as something that is 3D printed or laser cutted or CNC carved on demand, it involves a little waiting time. During this waiting time we can transform the book shop in a library, and offer full access to the titles in our bibliography.
|
||||
|
||||
4. In exchange we ask to the reader for some insights, notes or excerpts from the books. Those will be inserted in the inventory of our loot box.
|
||||
|
||||
5. This loot box can be accessed online from the website of SI17. It works exaclty as a classic one, except that we offer it for free. The content is a collection of thoughts questioning the issue of our project, in the context around our bibliography and readers. It could be an effective way to offer our research to that kind of public that has no means to access it.
|
||||
|
||||
6. To open the online loot box and get one (more or less random?) excerpt, the user is asked to draw a decorator. This could be made with a super simple web interface. The drawing will be the next digital manufactured decorator.
|
||||
|
||||
7. In the website of SI17 we can keep track of the decorators as well as the exceprts, in a process of inventory and world building.
|
||||
|
||||
|
||||
## Skin care routine
|
||||
|
||||
This idea of decorator is somehow similar to the concept of skin (in videogame terms). Here our decorator acts as cosmetic in the same way a fancy hat decorates your sniper in Team Fortress 2.
|
||||
|
||||
In the game itself the skin is nothing more than a visual candy. But once you look at the turbulence it puts in motion in the game superstructure, you realize that the kind of power-up it offers is something that acts in the social sphere around the game. (See: peer pressure, emotional commitment, skins gambling, product placement, collectibles)
|
||||
|
||||
A loot of lot boxes promise rare skins, and by doing so it lures in players. We could subvert this process by taking the skin out of the box.
|
||||
|
||||
Instead of opening it to get a new skin, you design a new skin (the decorator!) to open the loot box.
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
<!-- ### OLD DRAFT
|
||||
|
||||
1. Someone wants to buy our _olot xbox_ at PNF: nice!
|
||||
2. They discover that our _tool oxb_ can be purchased only if it decorates another product. What's more is that the _bloo tox_ acts actually as a discount for the other product (!!!). For example: if you decorate [A choreographer's score](https://www.rosas.be/nl/publications/425-a-choreographer-s-score-fase-rosas-danst-rosas-elena-s-aria-bartok) with our _blur fox_ it costs 10% less, amazin!
|
||||
3. The only constrain is: when the decorator-_otol xobo_ will be ready it would be nice to collect some meaningful excerpt from the book that the player want to buy.
|
||||
4. By the way our _tlob xob_ requires [1 hour to be ready](https://hub.xpub.nl/soupboat/~kamo/projects/loot-box-sealing-device/), so A) there is [plenty of leisure time to reclaim](https://hub.xpub.nl/soupboat/~kamo/projects/loot-box-temporality/) and B) it can be used for delve a bit into the contents and write some annotations for who has not access to it, for economical or temporal reasons.
|
||||
5. The excerpts are putted in a real _lool xox_.
|
||||
6. This _llll lll_ is the website of _the bbb_ SI17
|
||||
7. This _oooo ooo_ can be opened and the contents delivered to someone who wants a fast insight of our SI17.
|
||||
8. In order to open it, a key need to be drawn through a simple interface on the website (obv also super mobile friendly)
|
||||
9. And guess where this key will end up? Nothing less than 3D printed as the next _toto tot_ as decorator at PNF
|
||||
10. That will provide new excerpts for the digital _bbbb bbb_ in exchange of a super nice 3d printed decurator
|
||||
11. wow
|
||||
|
||||
Variant or elaboration of point 4.:
|
||||
During that hour the person is free to look at everything in PNF. In this hour the book store is transformed in a library.
|
||||
|
||||
Ok it makes super sense in my head right now but are like 3 in the morning and for sure im skipping passages in the process, it will seem super obscure I'm so sorry. Will elaborate more but atm happy with it ciao good night
|
||||
-->
|
||||
|
||||
|
@ -0,0 +1,107 @@
|
||||
---
|
||||
title: Loot Box as a Decorator
|
||||
date: 11/02/2022
|
||||
description: Hermit crab in the book store
|
||||
cover: 080.png
|
||||
cover_alt: A Slowbro, notoriously slow pokemon
|
||||
categories:
|
||||
- SI17
|
||||
- Games
|
||||
- Research
|
||||
---
|
||||
|
||||
## 3 intuitions come together
|
||||
|
||||
Right now:
|
||||
|
||||
1. A _loot box_ within a context as such: a book store
|
||||
2. A _loot box_ within a temporality
|
||||
3. A _loot box_ with different kinds of public
|
||||
|
||||
Over me 🦶🦶 🥁 🦵🦵 📀----
|
||||
|
||||
|
||||
|
||||
### Context
|
||||
|
||||
A _lolt box_ accellerates and forces the mechanics of an environment. In some games it can speed up some tedious process, in other it offers a specific special instant rewarding. Our _loot bbx_ inhabits a book store, or more in general a cultural space. In which ways can we hack through the normal functioning of such place? At a certain point today I thought: _ah, we could fill it with the last page of every books in Page Not Found_, just to say something about the presumed shortcuts that the _loat box_ promises to the player. The idea is kinda fun, but then what? So maybe no.
|
||||
|
||||
### Temporality
|
||||
|
||||
A couple of days ago I wrote some notes about the temporality of the _loto box_. In 1 sentence the idea is: if the _lot bx_ is a mechanism of instant rewarding, we could hijack and inflate its tempo and then fill it with our contents. Instead of opening in 30 seconds, the _loot bocs_ takes one hour. Meanwhile we can deliver our messages.
|
||||
|
||||
Today I read _Play like a feminist_ by _Shira Chess_ and guess what: there's an entire part about the temporality of leisure → 🤯
|
||||
|
||||
There is something really important we should keep in mind: we are aiming to a public that is etherogeneous. The intersectional approach that Chess advocates it's a reminder that we can inflate the temporality of the _loot biusch_, but not everyone will have access to it. So we need to think at both the limits of this spectrum, and put them in a meaningful relation.
|
||||
|
||||
### Public
|
||||
|
||||
As said: our public could be complex. For sure there will be some ultra publishing nerd that will sip all our soup and will be happy with it, but isn't 1 of our goals to reach also the world outside XPUB? _Chess_ in her book writes about micro temporality, little timespans carved between work shifts or commutes. She has a point when writes that with smartphones leisure time is more affordable and is detached from the rigid tempo of labour.
|
||||
|
||||
## Decorator
|
||||
|
||||
Combining these three aspects the question is: can we create a relation between who can spend an hour at PNF waiting for the _loot bosx_ and who cannot?
|
||||
|
||||
Enter the _boot lox_ as a decorator.
|
||||
|
||||
A decorator is something that adorn something else. In Python and object-oriented programming in general is also a name of a design pattern that adds some functionality to other functions. We used it already also with Flask! A _oobt olx_ as a decorator means that we could attach it to other pubblication at PNF. Something like an hermit crab inside other shells or that spiky things that bites the tail of a Slowpoke.
|
||||
|
||||
|
||||
### The setup
|
||||
|
||||
1. The physical decorator, that is a digital manufactured object produced on demand
|
||||
2. A catalogue of books that can be decorated
|
||||
3. A website with a digital loot box
|
||||
|
||||
### The process
|
||||
|
||||
1. As a part of the research we compose a bibliography that is also a statement i.e: _away from the cis white west guys gang_. This bibliography could be site specific for PNF or the other places we will distribute our SI17. We should choose to sell our pubblication in book stores or spaces that want to host this bibliography in their inventory. In this way we can use our SI17 as a device to reclaim space for marginal and subaltern voices.
|
||||
|
||||
2. The decorator inhabits this bibliography. It is presented as a special offer in which you can buy one of the book from the bibliography and receive a decorated version of it. Maybe we can sort out some kind of discount mechanism using part of the budget we have. The point is to favor access.
|
||||
|
||||
3. The deal is that the production of the decorator has a certain temporality: if we imagine it as something that is 3D printed or laser cutted or CNC carved on demand, it involves a little waiting time. During this waiting time we can transform the book shop in a library, and offer full access to the titles in our bibliography.
|
||||
|
||||
4. In exchange we ask to the reader for some insights, notes or excerpts from the books. Those will be inserted in the inventory of our loot box.
|
||||
|
||||
5. This loot box can be accessed online from the website of SI17. It works exaclty as a classic one, except that we offer it for free. The content is a collection of thoughts questioning the issue of our project, in the context around our bibliography and readers. It could be an effective way to offer our research to that kind of public that has no means to access it.
|
||||
|
||||
6. To open the online loot box and get one (more or less random?) excerpt, the user is asked to draw a decorator. This could be made with a super simple web interface. The drawing will be the next digital manufactured decorator.
|
||||
|
||||
7. In the website of SI17 we can keep track of the decorators as well as the exceprts, in a process of inventory and world building.
|
||||
|
||||
|
||||
## Skin care routine
|
||||
|
||||
This idea of decorator is somehow similar to the concept of skin (in videogame terms). Here our decorator acts as cosmetic in the same way a fancy hat decorates your sniper in Team Fortress 2.
|
||||
|
||||
In the game itself the skin is nothing more than a visual candy. But once you look at the turbulence it puts in motion in the game superstructure, you realize that the kind of power-up it offers is something that acts in the social sphere around the game. (See: peer pressure, emotional commitment, skins gambling, product placement, collectibles)
|
||||
|
||||
A loot of lot boxes promise rare skins, and by doing so it lures in players. We could subvert this process by taking the skin out of the box.
|
||||
|
||||
Instead of opening it to get a new skin, you design a new skin (the decorator!) to open the loot box.
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
<!-- ### OLD DRAFT
|
||||
|
||||
1. Someone wants to buy our _olot xbox_ at PNF: nice!
|
||||
2. They discover that our _tool oxb_ can be purchased only if it decorates another product. What's more is that the _bloo tox_ acts actually as a discount for the other product (!!!). For example: if you decorate [A choreographer's score](https://www.rosas.be/nl/publications/425-a-choreographer-s-score-fase-rosas-danst-rosas-elena-s-aria-bartok) with our _blur fox_ it costs 10% less, amazin!
|
||||
3. The only constrain is: when the decorator-_otol xobo_ will be ready it would be nice to collect some meaningful excerpt from the book that the player want to buy.
|
||||
4. By the way our _tlob xob_ requires [1 hour to be ready](https://hub.xpub.nl/soupboat/~kamo/projects/loot-box-sealing-device/), so A) there is [plenty of leisure time to reclaim](https://hub.xpub.nl/soupboat/~kamo/projects/loot-box-temporality/) and B) it can be used for delve a bit into the contents and write some annotations for who has not access to it, for economical or temporal reasons.
|
||||
5. The excerpts are putted in a real _lool xox_.
|
||||
6. This _llll lll_ is the website of _the bbb_ SI17
|
||||
7. This _oooo ooo_ can be opened and the contents delivered to someone who wants a fast insight of our SI17.
|
||||
8. In order to open it, a key need to be drawn through a simple interface on the website (obv also super mobile friendly)
|
||||
9. And guess where this key will end up? Nothing less than 3D printed as the next _toto tot_ as decorator at PNF
|
||||
10. That will provide new excerpts for the digital _bbbb bbb_ in exchange of a super nice 3d printed decurator
|
||||
11. wow
|
||||
|
||||
Variant or elaboration of point 4.:
|
||||
During that hour the person is free to look at everything in PNF. In this hour the book store is transformed in a library.
|
||||
|
||||
Ok it makes super sense in my head right now but are like 3 in the morning and for sure im skipping passages in the process, it will seem super obscure I'm so sorry. Will elaborate more but atm happy with it ciao good night
|
||||
-->
|
||||
|
||||
|
@ -0,0 +1,30 @@
|
||||
---
|
||||
title: Multi Player Loot Box
|
||||
date: 04/02/2022
|
||||
description: Notes to generate relations within the public
|
||||
cover: lost-in-time-2.png
|
||||
cover_alt: Bugs bunny lost in time cut scene
|
||||
categories:
|
||||
- SI17
|
||||
- Games
|
||||
- Research
|
||||
---
|
||||
|
||||
If the public of the classical loot box is made of individuals that are easier to exploit, our SI17 could research on ways to generate relations within the public.
|
||||
|
||||
|
||||
|
||||
## Homogeneous public?
|
||||
|
||||
The classical loot box assumes two main things:
|
||||
|
||||
* That the public is an homogeneous group of individual users
|
||||
* That the relation between the loot box and its public should be always the same
|
||||
|
||||
The loot box offers a limited amount of agency to the player. There is no quality in the interaction with it. The only way to use and access its content is to open it (and this usually means to pay). From the point of view of the loot box every player is the same, an their abilities or features or uniqueness have no meaning at all. One could say that the loot box is a popular device since is an object with a common interface for everyone, but is this really the case?
|
||||
|
||||
The interaction with the loot box has no quality, but for sure it implies some kind of quantity. To access is it required to spend a certain amount of money or time. This quantifiable expense is presented as a flat access scheme with homogeneous outcomes, but it is not. The effects of spending hundred €€€ in Fortnite skins are different for a kid and a streamer, for example. [While the streamer on Twitch spends 800€ in a row](https://www.youtube.com/watch?v=EXy83qr9jrI) to gain a thousandfold through sponsorizations and views, the average kid just throws away half of his mother's monthly income.
|
||||
|
||||
The public of the loot box is not homogeneous.
|
||||
|
||||
Keeping that in mind, we could rethink the basic way of interaction with the loot box. What if we offer something different from the flat price scheme? What if someone can pay less to access to the contents and someone else must pay more? Could this be a way to inject different qualities in the interaction with the loot box?
|
@ -0,0 +1,30 @@
|
||||
---
|
||||
title: Multi Player Loot Box
|
||||
date: 04/02/2022
|
||||
description: Notes to generate relations within the public
|
||||
cover: lost-in-time-2.png
|
||||
cover_alt: Bugs bunny lost in time cut scene
|
||||
categories:
|
||||
- SI17
|
||||
- Games
|
||||
- Research
|
||||
---
|
||||
|
||||
If the public of the classical loot box is made of individuals that are easier to exploit, our SI17 could research on ways to generate relations within the public.
|
||||
|
||||
|
||||
|
||||
## Homogeneous public?
|
||||
|
||||
The classical loot box assumes two main things:
|
||||
|
||||
* That the public is an homogeneous group of individual users
|
||||
* That the relation between the loot box and its public should be always the same
|
||||
|
||||
The loot box offers a limited amount of agency to the player. There is no quality in the interaction with it. The only way to use and access its content is to open it (and this usually means to pay). From the point of view of the loot box every player is the same, an their abilities or features or uniqueness have no meaning at all. One could say that the loot box is a popular device since is an object with a common interface for everyone, but is this really the case?
|
||||
|
||||
The interaction with the loot box has no quality, but for sure it implies some kind of quantity. To access is it required to spend a certain amount of money or time. This quantifiable expense is presented as a flat access scheme with homogeneous outcomes, but it is not. The effects of spending hundred €€€ in Fortnite skins are different for a kid and a streamer, for example. [While the streamer on Twitch spends 800€ in a row](https://www.youtube.com/watch?v=EXy83qr9jrI) to gain a thousandfold through sponsorizations and views, the average kid just throws away half of his mother's monthly income.
|
||||
|
||||
The public of the loot box is not homogeneous.
|
||||
|
||||
Keeping that in mind, we could rethink the basic way of interaction with the loot box. What if we offer something different from the flat price scheme? What if someone can pay less to access to the contents and someone else must pay more? Could this be a way to inject different qualities in the interaction with the loot box?
|
@ -0,0 +1,104 @@
|
||||
---
|
||||
title: LOOT—BOX—SEALING—DEVICE
|
||||
date: 04/02/2022
|
||||
description: Closing Pandora's 3D large jar
|
||||
categories:
|
||||
- SI17
|
||||
- Games
|
||||
- Proposal
|
||||
cover: test-3d.jpg
|
||||
cover_alt: 3d sculpted loot boxes
|
||||
---
|
||||
|
||||
## 3D printed loot box?
|
||||
|
||||
This is an idea that follows some intuitions regarding the [temporality of the loot box](/soupboat/~kamo/projects/loot-box-temporality/).
|
||||
|
||||
Imagine the loot box being 3D printed, and especially 3D printed on demand when the player want to buy it at Page Not Found or Varia or any other place we are going to distribute our work. 3D printing is a slow process, and in order to create a small piece you need to wait let's say an hour. When someone want to buy our loot box goes to PNF and ask for it, the 3d printing process begins and during the waiting time the player can access and navigate through the contents of our special issue. These contents are contained inside the temporality of the l~b, but they are not consumed instantaneously.
|
||||
|
||||

|
||||
|
||||
How do we want to deliver these contents? It could be related to the way of production of the physical l~b, for instance each player could contribute and shape the 3d model for the next player during the waiting time, and we can aggregate and collect narrations within and around the tools used in order to do so.
|
||||
|
||||
In order to cover the expenses of a similar process part of the SI17 budget could cover the cost for some small 3D printers and printing material. The term of services of our special issue could allocate a certain amount of money from each purchase to self sustain the process (buying new printing material, etc)
|
||||
|
||||
|
||||

|
||||
|
||||
|
||||
|
||||
## The loot—box—sealing—device
|
||||
|
||||
In the movie _The NeverEnding Story_ based on the novel by Michael Ende, the two main characters are linked together by the Auryn. In the (fictional) real world the Auryn is a sigil on the cover of the (fictional) Neverending Story book that tells the tales of the land of Fantasia. In Fantasia, the Auryn is a magic medalion that the hero wears during his mission.
|
||||
|
||||
|
||||

|
||||

|
||||
|
||||
Here the Auryn acts as a seal: by removing it from the cover of the book the magical world of Fantasia begins to leak inside the (fictional) real world. Later on it will be the main character to fall inside the (fictional) book of the Neverending Story.
|
||||
|
||||
This plot from Michael Ende resembles what happens when we play a game. Thanks to a weird device like a table game, a console or just a set of shared principles, we are able to flow into the [magic circle](https://en.wikipedia.org/wiki/Magic_circle_(virtual_worlds)). In the novel this happens with the main character reading a book, and while it's true that every cultural object offers a certain degree of immersivity, the kind of agency, interaction and participation in the NeverEnding Story is something that reminds me more the act of playing.
|
||||
|
||||

|
||||
|
||||
To elaborate more on the 3D printed loot box: we could have a book and using the 3d printer to seal it with a new 3d sigil every time! In a way it is like __sealing the loot box instead of opening it__. As in the NeverEnding Story (but this is a recurrent magical trope) we would have a sigil connecting who is reading the book with another player. This connection will be not with a fictional character, but with a real person: the one that bought the previous book. There is something like the [reciprocity](https://pzwiki.wdka.nl/mediadesign/Glossary_of_productive_play#Reciprocity) descripted by Mausse here, but is a reciprocity swimming backstroke: the player receives a gift from a past uknown fellow player, and leaves one for a future unkown one. In this way the reciprocity chain (sounds weird) goes spiral.
|
||||
|
||||

|
||||
|
||||
|
||||
|
||||
|
||||
## Overview
|
||||
|
||||
Here a brief description of the different pieces that compose the loot—box—sealing—device
|
||||
|
||||
1. The pubblication is composed of 2 main parts: a base and a superstructure. omg sorry
|
||||
2. The base is the same for every copy, and it's where the main contents are. (imagine it like a book)
|
||||
3. The superstructure is dynamic, it is produced and added to the base when and where the pubblication is purchased by someone. (imagine it like a 3d printed something)
|
||||
4. The production of the superstructure inflates the temporality of the loot box: our narration can inhabit this timespan.
|
||||
5. While someone wait for the 3d sigil to be printed we can offer a _temporary safe zone_: a checkpoint at PNF (or other locations)
|
||||
6. In this _temporary safe zone_ the player can leave something for the next player by designing the next sigil and
|
||||
7. In this _temporary safe zone_ the player can be guided through the contents of the pubblication while waiting for the superstructure to be produced
|
||||
8. When a new copy of the pubblication is bought, a sigil is 3d printed and then uploaded on the website of the SI17 as documentation
|
||||
|
||||
|
||||
|
||||
|
||||
### 1. Physical pubblication (a book? a box? something)
|
||||
I don't really know which kind of contents, but since we are reading a lot could be intresting to prepare a critical reader about the loot box issues, collecting different perspectives and heterogeneous voices? Then production mode ok and then we print say 100 copies. Our magical technical book binding team figures out the best way in which we can add some dynamic components or 3D addition to the cover-object-book, but we don't do that yet. We just leave the pubblications without the 3d cherry on the cake.
|
||||
|
||||
### 2. A custom 3d sculpting software
|
||||
_Note that the process could be also implemented with totally different techniques based on digital manufacturing like wood working with cnc, laser cut, etc endless possibilities, but let's say we want these exoterical 3d printed sigils._
|
||||
|
||||
We can develop a simple 3D sculpting software that even people not used to 3D modeling could use. Something like this [SculptGL](https://stephaneginier.com/sculptgl/). This is not super easy to do, but not as hard as an API. We could start from some open source thing and then customize it in the way we need. [Blender](https://www.blender.org/) is written in Python and has a super nice API for programming custom plugins, for example.
|
||||
|
||||
Side note totally (not totally) unrelated: plugin republishing? 🤯 Ahah it would be great to publish some excerpts from Simone Weil inside the UI of Blender or Photoshop or whatever. Injecting culture in the cultural industry tools.
|
||||
|
||||
|
||||
### 3. Some templates for the 3d sigil
|
||||
|
||||
- material and practical needs
|
||||
- SI17 visual identity as a starting point
|
||||
- SI17 contents as orientation
|
||||
- SI17 world building as heading
|
||||
|
||||
(wip)
|
||||
|
||||
### 4. A 3D Printing device
|
||||
|
||||
- A 3D printer
|
||||
- Some interface to sculpt the next 3d seal (aka 1 pc)
|
||||
- A nice setup for display everything (checkpoint? treasure chest? loot box?)
|
||||
|
||||
(wip)
|
||||
|
||||
### 5. A website
|
||||
|
||||
- Info
|
||||
- Inventory that keep track of the sigils (world building)
|
||||
|
||||
(wip)
|
||||
|
||||
|
||||
|
||||
<video src="/soupboat/~kamo/static/video/lootboxes 3d meme.mp4" autoplay loop></video>
|
@ -0,0 +1,104 @@
|
||||
---
|
||||
title: LOOT—BOX—SEALING—DEVICE
|
||||
date: 04/02/2022
|
||||
description: Closing Pandora's 3D large jar
|
||||
categories:
|
||||
- SI17
|
||||
- Games
|
||||
- Proposal
|
||||
cover: test-3d.jpg
|
||||
cover_alt: 3d sculpted loot boxes
|
||||
---
|
||||
|
||||
## 3D printed loot box?
|
||||
|
||||
This is an idea that follows some intuitions regarding the [temporality of the loot box](/soupboat/~kamo/projects/loot-box-temporality/).
|
||||
|
||||
Imagine the loot box being 3D printed, and especially 3D printed on demand when the player want to buy it at Page Not Found or Varia or any other place we are going to distribute our work. 3D printing is a slow process, and in order to create a small piece you need to wait let's say an hour. When someone want to buy our loot box goes to PNF and ask for it, the 3d printing process begins and during the waiting time the player can access and navigate through the contents of our special issue. These contents are contained inside the temporality of the l~b, but they are not consumed instantaneously.
|
||||
|
||||

|
||||
|
||||
How do we want to deliver these contents? It could be related to the way of production of the physical l~b, for instance each player could contribute and shape the 3d model for the next player during the waiting time, and we can aggregate and collect narrations within and around the tools used in order to do so.
|
||||
|
||||
In order to cover the expenses of a similar process part of the SI17 budget could cover the cost for some small 3D printers and printing material. The term of services of our special issue could allocate a certain amount of money from each purchase to self sustain the process (buying new printing material, etc)
|
||||
|
||||
|
||||

|
||||
|
||||
|
||||
|
||||
## The loot—box—sealing—device
|
||||
|
||||
In the movie _The NeverEnding Story_ based on the novel by Michael Ende, the two main characters are linked together by the Auryn. In the (fictional) real world the Auryn is a sigil on the cover of the (fictional) Neverending Story book that tells the tales of the land of Fantasia. In Fantasia, the Auryn is a magic medalion that the hero wears during his mission.
|
||||
|
||||
|
||||

|
||||

|
||||
|
||||
Here the Auryn acts as a seal: by removing it from the cover of the book the magical world of Fantasia begins to leak inside the (fictional) real world. Later on it will be the main character to fall inside the (fictional) book of the Neverending Story.
|
||||
|
||||
This plot from Michael Ende resembles what happens when we play a game. Thanks to a weird device like a table game, a console or just a set of shared principles, we are able to flow into the [magic circle](https://en.wikipedia.org/wiki/Magic_circle_(virtual_worlds)). In the novel this happens with the main character reading a book, and while it's true that every cultural object offers a certain degree of immersivity, the kind of agency, interaction and participation in the NeverEnding Story is something that reminds me more the act of playing.
|
||||
|
||||

|
||||
|
||||
To elaborate more on the 3D printed loot box: we could have a book and using the 3d printer to seal it with a new 3d sigil every time! In a way it is like __sealing the loot box instead of opening it__. As in the NeverEnding Story (but this is a recurrent magical trope) we would have a sigil connecting who is reading the book with another player. This connection will be not with a fictional character, but with a real person: the one that bought the previous book. There is something like the [reciprocity](https://pzwiki.wdka.nl/mediadesign/Glossary_of_productive_play#Reciprocity) descripted by Mausse here, but is a reciprocity swimming backstroke: the player receives a gift from a past uknown fellow player, and leaves one for a future unkown one. In this way the reciprocity chain (sounds weird) goes spiral.
|
||||
|
||||

|
||||
|
||||
|
||||
|
||||
|
||||
## Overview
|
||||
|
||||
Here a brief description of the different pieces that compose the loot—box—sealing—device
|
||||
|
||||
1. The pubblication is composed of 2 main parts: a base and a superstructure. omg sorry
|
||||
2. The base is the same for every copy, and it's where the main contents are. (imagine it like a book)
|
||||
3. The superstructure is dynamic, it is produced and added to the base when and where the pubblication is purchased by someone. (imagine it like a 3d printed something)
|
||||
4. The production of the superstructure inflates the temporality of the loot box: our narration can inhabit this timespan.
|
||||
5. While someone wait for the 3d sigil to be printed we can offer a _temporary safe zone_: a checkpoint at PNF (or other locations)
|
||||
6. In this _temporary safe zone_ the player can leave something for the next player by designing the next sigil and
|
||||
7. In this _temporary safe zone_ the player can be guided through the contents of the pubblication while waiting for the superstructure to be produced
|
||||
8. When a new copy of the pubblication is bought, a sigil is 3d printed and then uploaded on the website of the SI17 as documentation
|
||||
|
||||
|
||||
|
||||
|
||||
### 1. Physical pubblication (a book? a box? something)
|
||||
I don't really know which kind of contents, but since we are reading a lot could be intresting to prepare a critical reader about the loot box issues, collecting different perspectives and heterogeneous voices? Then production mode ok and then we print say 100 copies. Our magical technical book binding team figures out the best way in which we can add some dynamic components or 3D addition to the cover-object-book, but we don't do that yet. We just leave the pubblications without the 3d cherry on the cake.
|
||||
|
||||
### 2. A custom 3d sculpting software
|
||||
_Note that the process could be also implemented with totally different techniques based on digital manufacturing like wood working with cnc, laser cut, etc endless possibilities, but let's say we want these exoterical 3d printed sigils._
|
||||
|
||||
We can develop a simple 3D sculpting software that even people not used to 3D modeling could use. Something like this [SculptGL](https://stephaneginier.com/sculptgl/). This is not super easy to do, but not as hard as an API. We could start from some open source thing and then customize it in the way we need. [Blender](https://www.blender.org/) is written in Python and has a super nice API for programming custom plugins, for example.
|
||||
|
||||
Side note totally (not totally) unrelated: plugin republishing? 🤯 Ahah it would be great to publish some excerpts from Simone Weil inside the UI of Blender or Photoshop or whatever. Injecting culture in the cultural industry tools.
|
||||
|
||||
|
||||
### 3. Some templates for the 3d sigil
|
||||
|
||||
- material and practical needs
|
||||
- SI17 visual identity as a starting point
|
||||
- SI17 contents as orientation
|
||||
- SI17 world building as heading
|
||||
|
||||
(wip)
|
||||
|
||||
### 4. A 3D Printing device
|
||||
|
||||
- A 3D printer
|
||||
- Some interface to sculpt the next 3d seal (aka 1 pc)
|
||||
- A nice setup for display everything (checkpoint? treasure chest? loot box?)
|
||||
|
||||
(wip)
|
||||
|
||||
### 5. A website
|
||||
|
||||
- Info
|
||||
- Inventory that keep track of the sigils (world building)
|
||||
|
||||
(wip)
|
||||
|
||||
|
||||
|
||||
<video src="/soupboat/~kamo/static/video/lootboxes 3d meme.mp4" autoplay loop></video>
|
@ -0,0 +1,32 @@
|
||||
---
|
||||
title: Temporality of the loot box
|
||||
date: 01/02/2022
|
||||
description: Against instant rewarding
|
||||
cover: sheep_raider.jpg
|
||||
cover_alt: Sheep raider nice game of when i was la monte young
|
||||
categories:
|
||||
- SI17
|
||||
- Games
|
||||
- Research
|
||||
---
|
||||
|
||||
## Bill Viola opens a loot box
|
||||
|
||||
The loot box implies a specific temporal dimension: the one with instant rewarding. When a player opens the loot box she receives immediate feedback. Sometimes it is dressed up with an aesthetic of suspense, but this is just cosmetics and the built-up climax often becomes just something undesired that the user wants (and even pay) to skip.
|
||||
|
||||
In order to work with the idea of the loot box without re-enacting its toxic behavior and mechanics it could be interesting to hijack its temporality. By inflating the time between the purchasing and the result, we could create space for dig deeper in this complex and delicate topic.
|
||||
|
||||
|
||||
Loot box
|
||||
`pay ●-->○ get`
|
||||
|
||||
SI17 Loot Box
|
||||
`pay ●--things could happen here-->○ get`
|
||||
|
||||
This approach could help us in filling the loot box (tempo) without falling for the same addictive schemes that the industry is implementing for exploiting the players.
|
||||
|
||||
__Inflating the loot box means that the player could reclaim her own leisure time.__ If we focus on the temporal fruition of the l~b we can imagine to produce not only an object, but a time slot that the person from the public can reserve for herself. If we define this time slot as leisure time then we could create a sacred and safe space to take a rest and to arrest the acceleration of capital. Something like a checkpoint, speaking from a gaming point of view.
|
||||
|
||||
An approach to deal with the temporal aspect in a way that doesn't feel forced could be to rely on _real-yet-slow-time_ processes for the material production of the special issue. A digital manufacturing production could make a lot of sense in this context. 👀
|
||||
|
||||
See the [loot—box—sealing—device](/soupboat/~kamo/projects/loot-box-sealing-device/) for a concrete and 3d example
|
@ -0,0 +1,32 @@
|
||||
---
|
||||
title: Temporality of the loot box
|
||||
date: 01/02/2022
|
||||
description: Against instant rewarding
|
||||
cover: sheep_raider.jpg
|
||||
cover_alt: Sheep raider nice game of when i was la monte young
|
||||
categories:
|
||||
- SI17
|
||||
- Games
|
||||
- Research
|
||||
---
|
||||
|
||||
## Bill Viola opens a loot box
|
||||
|
||||
The loot box implies a specific temporal dimension: the one with instant rewarding. When a player opens the loot box she receives immediate feedback. Sometimes it is dressed up with an aesthetic of suspense, but this is just cosmetics and the built-up climax often becomes just something undesired that the user wants (and even pay) to skip.
|
||||
|
||||
In order to work with the idea of the loot box without re-enacting its toxic behavior and mechanics it could be interesting to hijack its temporality. By inflating the time between the purchasing and the result, we could create space for dig deeper in this complex and delicate topic.
|
||||
|
||||
|
||||
Loot box
|
||||
`pay ●-->○ get`
|
||||
|
||||
SI17 Loot Box
|
||||
`pay ●--things could happen here-->○ get`
|
||||
|
||||
This approach could help us in filling the loot box (tempo) without falling for the same addictive schemes that the industry is implementing for exploiting the players.
|
||||
|
||||
__Inflating the loot box means that the player could reclaim her own leisure time.__ If we focus on the temporal fruition of the l~b we can imagine to produce not only an object, but a time slot that the person from the public can reserve for herself. If we define this time slot as leisure time then we could create a sacred and safe space to take a rest and to arrest the acceleration of capital. Something like a checkpoint, speaking from a gaming point of view.
|
||||
|
||||
An approach to deal with the temporal aspect in a way that doesn't feel forced could be to rely on _real-yet-slow-time_ processes for the material production of the special issue. A digital manufacturing production could make a lot of sense in this context. 👀
|
||||
|
||||
See the [loot—box—sealing—device](/soupboat/~kamo/projects/loot-box-sealing-device/) for a concrete and 3d example
|
@ -0,0 +1,34 @@
|
||||
---
|
||||
title: Mimic research 📦
|
||||
description: Exploring a tricky treasure trope
|
||||
date: 04/02/2022
|
||||
categories:
|
||||
- SI17
|
||||
- Research
|
||||
- Games
|
||||
cover: The_Mimic.png
|
||||
cover_alt: A mimic from Runescape
|
||||
---
|
||||
|
||||
## 2 different types of treasure chest
|
||||
|
||||
In RPG games the Mimic is a monster that appears as a treasure chest. When a player tries to interact with it in order to get the contents of the chest, it reveals its true nature and attacks her. The name of the Mimic come from its act of mimesis: this creature is like a predator that disguises itself in order to sneak up on its prey.
|
||||
|
||||
A treasure chest in a game can be seen as a _temporary safe zone_ because it interrupts the flow of incoming threats by offering a reward to the player. The Mimic endangers this _temporary safe zone_, and breaks a kind of contract between the player and the game. The treasure chest is transformed in a risky russian roulette, that inoculates danger in the safe zones of a narration.
|
||||
|
||||
I'm tempted to write here that the loot box is something like a _meta mimic_: an object that promises an in-game reward, but produces a damage to the player. What's more is that this damage is inflicted in the real world, not to the player but to the person. What's then the difference between a loot box and a Mimic?
|
||||
|
||||
Starting from the [Dungeons and Dragons' Mimic](https://en.wikipedia.org/wiki/Mimic_(Dungeons_%26_Dragons)) I'd like to explore the evolution and the ecology of the mimic through different games. How do the game designers choose where Mimics spawn? What are the relations between those creatures, the level design, the stress of the player, as well as her expectations and trust in the game world? Are there similarities in the way the Mimics and the loot boxes are presented to the player?
|
||||
|
||||
__TODO: amazon package but has fangs__
|
||||
|
||||
<!-- ## Mimics gallery
|
||||
|
||||

|
||||
Mimics from D&D
|
||||
|
||||

|
||||
Baldur's Gate Killer Mimic
|
||||
|
||||

|
||||
[Dragon Quest Mimic](https://dragon-quest.org/wiki/Mimic) -->
|
@ -0,0 +1,34 @@
|
||||
---
|
||||
title: Mimic research 📦
|
||||
description: Exploring a tricky treasure trope
|
||||
date: 04/02/2022
|
||||
categories:
|
||||
- SI17
|
||||
- Research
|
||||
- Games
|
||||
cover: The_Mimic.png
|
||||
cover_alt: A mimic from Runescape
|
||||
---
|
||||
|
||||
## 2 different types of treasure chest
|
||||
|
||||
In RPG games the Mimic is a monster that appears as a treasure chest. When a player tries to interact with it in order to get the contents of the chest, it reveals its true nature and attacks her. The name of the Mimic come from its act of mimesis: this creature is like a predator that disguises itself in order to sneak up on its prey.
|
||||
|
||||
A treasure chest in a game can be seen as a _temporary safe zone_ because it interrupts the flow of incoming threats by offering a reward to the player. The Mimic endangers this _temporary safe zone_, and breaks a kind of contract between the player and the game. The treasure chest is transformed in a risky russian roulette, that inoculates danger in the safe zones of a narration.
|
||||
|
||||
I'm tempted to write here that the loot box is something like a _meta mimic_: an object that promises an in-game reward, but produces a damage to the player. What's more is that this damage is inflicted in the real world, not to the player but to the person. What's then the difference between a loot box and a Mimic?
|
||||
|
||||
Starting from the [Dungeons and Dragons' Mimic](https://en.wikipedia.org/wiki/Mimic_(Dungeons_%26_Dragons)) I'd like to explore the evolution and the ecology of the mimic through different games. How do the game designers choose where Mimics spawn? What are the relations between those creatures, the level design, the stress of the player, as well as her expectations and trust in the game world? Are there similarities in the way the Mimics and the loot boxes are presented to the player?
|
||||
|
||||
__TODO: amazon package but has fangs__
|
||||
|
||||
<!-- ## Mimics gallery
|
||||
|
||||

|
||||
Mimics from D&D
|
||||
|
||||

|
||||
Baldur's Gate Killer Mimic
|
||||
|
||||

|
||||
[Dragon Quest Mimic](https://dragon-quest.org/wiki/Mimic) -->
|
@ -0,0 +1,44 @@
|
||||
---
|
||||
title: Notation system for 2 or + fingers
|
||||
description: Drawing and performing scores with Chae
|
||||
date: 20/04/2022
|
||||
categories:
|
||||
- Notation
|
||||
- Drawings
|
||||
- Sound
|
||||
links:
|
||||
- url: https://hub.xpub.nl/soupboat/SI18/notations/chamo/initial-vanila.mp3
|
||||
title: 🎵
|
||||
- url: https://hub.xpub.nl/soupboat/SI18/notations/chamo/linzen-tefalexpress.mp3
|
||||
title: 🎶
|
||||
- url: https://hub.xpub.nl/soupboat/SI18/notations/chamo/
|
||||
title: Cover
|
||||
cover: monstro.jpg
|
||||
cover_alt: a nice statue at venice biennale 2022
|
||||
pad: https://pad.xpub.nl/p/notation_experiment_0420
|
||||
css: rotating.css
|
||||
|
||||
---
|
||||
|
||||
A notation system made with Chae during Steve's class.
|
||||
|
||||
Here are the instructions and some examples.
|
||||
|
||||
```
|
||||
1. You need at least two participants
|
||||
2. Each participant chooses one word. It can be a word that exist, or gibberish.
|
||||
3. The score is a collective drawing in which each line should cross at least another one.
|
||||
4. The score has no orientation, we suggest to draw it on a circular sheet.
|
||||
5. Follow the score with your finger and interpret it with your chosen word.
|
||||
6. When fingers meet, swap the word.
|
||||
```
|
||||
|
||||
<img src='notation_big.png' class="rotating" />
|
||||
|
||||
<img src='notation_medium.png' class="rotating" />
|
||||
|
||||
<img src='notation_small.png' class="rotating" />
|
||||
|
||||
|
||||
|
||||
|
@ -0,0 +1,44 @@
|
||||
---
|
||||
title: Notation system for 2 or + fingers
|
||||
description: Drawing and performing scores with Chae
|
||||
date: 20/04/2022
|
||||
categories:
|
||||
- Notation
|
||||
- Drawings
|
||||
- Sound
|
||||
links:
|
||||
- url: https://hub.xpub.nl/soupboat/SI18/notations/chamo/initial-vanila.mp3
|
||||
title: 🎵
|
||||
- url: https://hub.xpub.nl/soupboat/SI18/notations/chamo/linzen-tefalexpress.mp3
|
||||
title: 🎶
|
||||
- url: https://hub.xpub.nl/soupboat/SI18/notations/chamo/
|
||||
title: Cover
|
||||
cover: monstro.jpg
|
||||
cover_alt: a nice statue at venice biennale 2022
|
||||
pad: https://pad.xpub.nl/p/notation_experiment_0420
|
||||
css: rotating.css
|
||||
|
||||
---
|
||||
|
||||
A notation system made with Chae during Steve's class.
|
||||
|
||||
Here are the instructions and some examples.
|
||||
|
||||
```
|
||||
1. You need at least two participants
|
||||
2. Each participant chooses one word. It can be a word that exist, or gibberish.
|
||||
3. The score is a collective drawing in which each line should cross at least another one.
|
||||
4. The score has no orientation, we suggest to draw it on a circular sheet.
|
||||
5. Follow the score with your finger and interpret it with your chosen word.
|
||||
6. When fingers meet, swap the word.
|
||||
```
|
||||
|
||||
<img src='notation_big.png' class="rotating" />
|
||||
|
||||
<img src='notation_medium.png' class="rotating" />
|
||||
|
||||
<img src='notation_small.png' class="rotating" />
|
||||
|
||||
|
||||
|
||||
|
After Width: | Height: | Size: 237 KiB |
After Width: | Height: | Size: 197 KiB |
After Width: | Height: | Size: 106 KiB |