You cannot select more than 25 topics
Topics must start with a letter or number, can include dashes ('-') and can be up to 35 characters long.
1123 lines
57 KiB
Plaintext
1123 lines
57 KiB
Plaintext
4 years ago
|
{
|
||
|
"cells": [
|
||
|
{
|
||
|
"cell_type": "markdown",
|
||
|
"metadata": {},
|
||
|
"source": [
|
||
|
"# Patterns (part 1)"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "markdown",
|
||
|
"metadata": {},
|
||
|
"source": [
|
||
|
"## Generating patterns"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "markdown",
|
||
|
"metadata": {},
|
||
|
"source": [
|
||
|
"Generating patterns, weaving & textile design.\n",
|
||
|
"\n",
|
||
|
"> The structure of a fabric or its weave — that is, the fastening of its elements of threads to each other — is as much a determining factor in its function as is the choice of the raw material. In fact, the interrelation of the two, the subtle play between them in supporting, impeding, or modiying each other's characteristics, is the essence of weaving. (p. 38)\n",
|
||
|
"\n",
|
||
|
"<small>Anni Albers - On Weaving (1965), https://monoskop.org/images/7/71/Albers_Anni_On_Weaving_1974.pdf</small>\n",
|
||
|
"\n",
|
||
|
"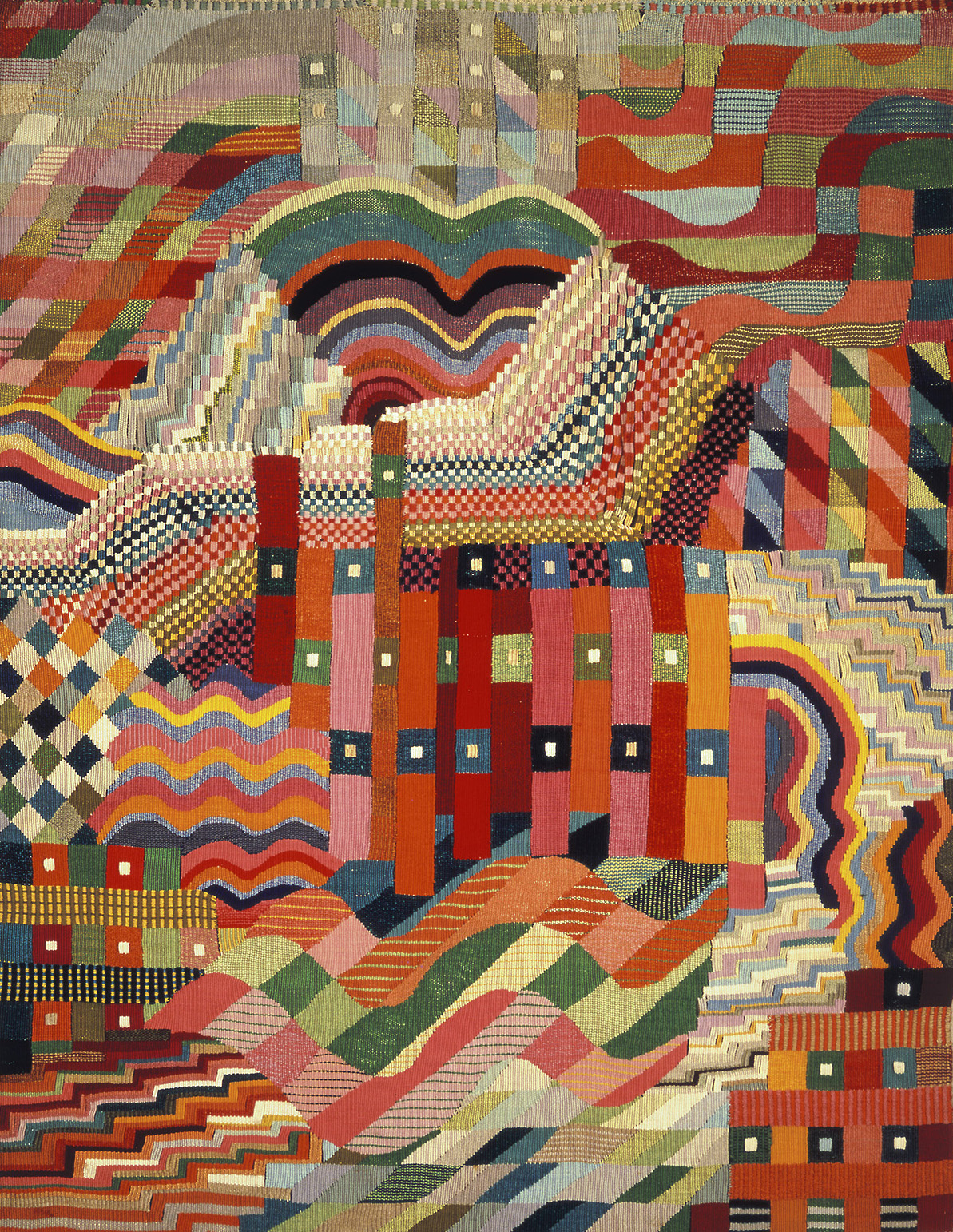\n",
|
||
|
"\n",
|
||
|
"<small>Red-Geen Slit Tapestry, Gunta Stölzl (1927/28)</small>"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "markdown",
|
||
|
"metadata": {},
|
||
|
"source": [
|
||
|
"-------------------"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "markdown",
|
||
|
"metadata": {},
|
||
|
"source": [
|
||
|
"### Re-turning (to): Variables, Lists & Loops"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": 5,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": [
|
||
|
"words = ['weaving', 'with', 'words', 'and', 'code']"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": 11,
|
||
|
"metadata": {},
|
||
|
"outputs": [
|
||
|
{
|
||
|
"name": "stdout",
|
||
|
"output_type": "stream",
|
||
|
"text": [
|
||
|
"weaving\n",
|
||
|
"with\n",
|
||
|
"words\n",
|
||
|
"and\n",
|
||
|
"code\n"
|
||
|
]
|
||
|
}
|
||
|
],
|
||
|
"source": [
|
||
|
"# First a simple loop through the list\n",
|
||
|
"for word in words:\n",
|
||
|
" print(word)"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": 21,
|
||
|
"metadata": {},
|
||
|
"outputs": [
|
||
|
{
|
||
|
"name": "stdout",
|
||
|
"output_type": "stream",
|
||
|
"text": [
|
||
|
"code weaving weaving code words\n",
|
||
|
"with with and code and\n",
|
||
|
"code code weaving and with\n",
|
||
|
"and weaving words code and\n",
|
||
|
"and with words words weaving\n"
|
||
|
]
|
||
|
}
|
||
|
],
|
||
|
"source": [
|
||
|
"# Then, a loop in which we start to play with random again\n",
|
||
|
"import random\n",
|
||
|
"for word in words:\n",
|
||
|
" print(random.choice(words), random.choice(words), random.choice(words), random.choice(words), random.choice(words))"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": 24,
|
||
|
"metadata": {},
|
||
|
"outputs": [
|
||
|
{
|
||
|
"data": {
|
||
|
"text/plain": [
|
||
|
"\u001b[0;31mInit signature:\u001b[0m \u001b[0mrange\u001b[0m\u001b[0;34m(\u001b[0m\u001b[0mself\u001b[0m\u001b[0;34m,\u001b[0m \u001b[0;34m/\u001b[0m\u001b[0;34m,\u001b[0m \u001b[0;34m*\u001b[0m\u001b[0margs\u001b[0m\u001b[0;34m,\u001b[0m \u001b[0;34m**\u001b[0m\u001b[0mkwargs\u001b[0m\u001b[0;34m)\u001b[0m\u001b[0;34m\u001b[0m\u001b[0;34m\u001b[0m\u001b[0m\n",
|
||
|
"\u001b[0;31mDocstring:\u001b[0m \n",
|
||
|
"range(stop) -> range object\n",
|
||
|
"range(start, stop[, step]) -> range object\n",
|
||
|
"\n",
|
||
|
"Return an object that produces a sequence of integers from start (inclusive)\n",
|
||
|
"to stop (exclusive) by step. range(i, j) produces i, i+1, i+2, ..., j-1.\n",
|
||
|
"start defaults to 0, and stop is omitted! range(4) produces 0, 1, 2, 3.\n",
|
||
|
"These are exactly the valid indices for a list of 4 elements.\n",
|
||
|
"When step is given, it specifies the increment (or decrement).\n",
|
||
|
"\u001b[0;31mType:\u001b[0m type\n",
|
||
|
"\u001b[0;31mSubclasses:\u001b[0m \n"
|
||
|
]
|
||
|
},
|
||
|
"metadata": {},
|
||
|
"output_type": "display_data"
|
||
|
}
|
||
|
],
|
||
|
"source": [
|
||
|
"# How to work with more iterations of the loop? \n",
|
||
|
"# For example 100?\n",
|
||
|
"# You can use \"range\"\n",
|
||
|
"range?"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": 26,
|
||
|
"metadata": {
|
||
|
"scrolled": true
|
||
|
},
|
||
|
"outputs": [
|
||
|
{
|
||
|
"name": "stdout",
|
||
|
"output_type": "stream",
|
||
|
"text": [
|
||
|
"words with and code words\n",
|
||
|
"words words and weaving with\n",
|
||
|
"and and and and code\n",
|
||
|
"words code weaving with weaving\n",
|
||
|
"code code code with weaving\n",
|
||
|
"and weaving weaving and with\n",
|
||
|
"weaving with words with and\n",
|
||
|
"and words and weaving and\n",
|
||
|
"words weaving code code weaving\n",
|
||
|
"code words words with code\n",
|
||
|
"weaving with words and code\n",
|
||
|
"weaving and and words weaving\n",
|
||
|
"and weaving weaving and words\n",
|
||
|
"with code weaving weaving and\n",
|
||
|
"with words words code with\n",
|
||
|
"code with weaving and weaving\n",
|
||
|
"weaving weaving weaving with with\n",
|
||
|
"weaving and code code with\n",
|
||
|
"words weaving and and weaving\n",
|
||
|
"weaving words weaving weaving weaving\n",
|
||
|
"with and with weaving and\n",
|
||
|
"and weaving with code code\n",
|
||
|
"code words words and code\n",
|
||
|
"weaving weaving weaving words and\n",
|
||
|
"and words words with and\n",
|
||
|
"and code code code weaving\n",
|
||
|
"weaving weaving words weaving with\n",
|
||
|
"words and code with code\n",
|
||
|
"weaving words and code code\n",
|
||
|
"and words words words words\n",
|
||
|
"weaving and code code weaving\n",
|
||
|
"with with words weaving and\n",
|
||
|
"words code weaving weaving code\n",
|
||
|
"code words words weaving weaving\n",
|
||
|
"words words code code words\n",
|
||
|
"words weaving with and and\n",
|
||
|
"with and and with and\n",
|
||
|
"with code code with words\n",
|
||
|
"with code code with words\n",
|
||
|
"words words and words code\n",
|
||
|
"code weaving words code code\n",
|
||
|
"weaving words and with with\n",
|
||
|
"with with weaving words and\n",
|
||
|
"weaving weaving weaving words code\n",
|
||
|
"and and weaving weaving weaving\n",
|
||
|
"code words code weaving weaving\n",
|
||
|
"with code and and code\n",
|
||
|
"with weaving weaving code words\n",
|
||
|
"and code weaving words with\n",
|
||
|
"and words words weaving weaving\n",
|
||
|
"with code with with code\n",
|
||
|
"weaving weaving code words words\n",
|
||
|
"and and and and words\n",
|
||
|
"words with weaving code weaving\n",
|
||
|
"words code with with code\n",
|
||
|
"code with with code code\n",
|
||
|
"and weaving and words code\n",
|
||
|
"code code with words and\n",
|
||
|
"code code and and words\n",
|
||
|
"and with words weaving weaving\n",
|
||
|
"with weaving with with and\n",
|
||
|
"words and words and with\n",
|
||
|
"weaving code words code words\n",
|
||
|
"with weaving with words words\n",
|
||
|
"weaving words and with and\n",
|
||
|
"code words and words and\n",
|
||
|
"and weaving weaving code code\n",
|
||
|
"with words and code words\n",
|
||
|
"with code weaving code code\n",
|
||
|
"code words code weaving and\n",
|
||
|
"words words code code weaving\n",
|
||
|
"weaving code and with and\n",
|
||
|
"with weaving with weaving words\n",
|
||
|
"with weaving and words and\n",
|
||
|
"and weaving words code and\n",
|
||
|
"with weaving with and code\n",
|
||
|
"weaving words with with words\n",
|
||
|
"words with weaving words and\n",
|
||
|
"weaving and words words words\n",
|
||
|
"with words weaving and and\n",
|
||
|
"weaving code weaving and words\n",
|
||
|
"weaving words weaving with code\n",
|
||
|
"with words and weaving weaving\n",
|
||
|
"code words with with code\n",
|
||
|
"weaving words words and weaving\n",
|
||
|
"and words words with and\n",
|
||
|
"and with code weaving code\n",
|
||
|
"code weaving words and weaving\n",
|
||
|
"with weaving words and and\n",
|
||
|
"code weaving and weaving and\n",
|
||
|
"with weaving code with weaving\n",
|
||
|
"and weaving code code with\n",
|
||
|
"and code words weaving words\n",
|
||
|
"and words weaving with code\n",
|
||
|
"words words weaving words with\n",
|
||
|
"code with with code and\n",
|
||
|
"words with code and code\n",
|
||
|
"code and and words with\n",
|
||
|
"with with words weaving and\n",
|
||
|
"words with weaving words and\n"
|
||
|
]
|
||
|
}
|
||
|
],
|
||
|
"source": [
|
||
|
"# Make a loop that starts at 0 and ends at 99 (100 iterations)\n",
|
||
|
"import random\n",
|
||
|
"for number in range(100):\n",
|
||
|
" print(random.choice(words), random.choice(words), random.choice(words), random.choice(words), random.choice(words))"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": 44,
|
||
|
"metadata": {},
|
||
|
"outputs": [
|
||
|
{
|
||
|
"name": "stdout",
|
||
|
"output_type": "stream",
|
||
|
"text": [
|
||
|
"3\n",
|
||
|
"4\n",
|
||
|
"5\n",
|
||
|
"6\n",
|
||
|
"7\n",
|
||
|
"8\n",
|
||
|
"9\n"
|
||
|
]
|
||
|
}
|
||
|
],
|
||
|
"source": [
|
||
|
"# You can use range also differently, for example to loop in between two numbers ...\n",
|
||
|
"for number in range(3,10):\n",
|
||
|
" print(number)"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": 43,
|
||
|
"metadata": {},
|
||
|
"outputs": [
|
||
|
{
|
||
|
"name": "stdout",
|
||
|
"output_type": "stream",
|
||
|
"text": [
|
||
|
"0\n",
|
||
|
"10\n",
|
||
|
"20\n",
|
||
|
"30\n",
|
||
|
"40\n",
|
||
|
"50\n",
|
||
|
"60\n",
|
||
|
"70\n",
|
||
|
"80\n",
|
||
|
"90\n"
|
||
|
]
|
||
|
}
|
||
|
],
|
||
|
"source": [
|
||
|
"# ... or with bigger steps:\n",
|
||
|
"for number in range(0,100,10):\n",
|
||
|
" print(number)"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": 4,
|
||
|
"metadata": {},
|
||
|
"outputs": [
|
||
|
{
|
||
|
"name": "stdout",
|
||
|
"output_type": "stream",
|
||
|
"text": [
|
||
|
"x y\n",
|
||
|
"x yy\n",
|
||
|
"x yyy\n",
|
||
|
"x yyyy\n",
|
||
|
"x yyyyy\n",
|
||
|
"xx y\n",
|
||
|
"xx yy\n",
|
||
|
"xx yyy\n",
|
||
|
"xx yyyy\n",
|
||
|
"xx yyyyy\n",
|
||
|
"xxx y\n",
|
||
|
"xxx yy\n",
|
||
|
"xxx yyy\n",
|
||
|
"xxx yyyy\n",
|
||
|
"xxx yyyyy\n",
|
||
|
"xxxx y\n",
|
||
|
"xxxx yy\n",
|
||
|
"xxxx yyy\n",
|
||
|
"xxxx yyyy\n",
|
||
|
"xxxx yyyyy\n",
|
||
|
"xxxxx y\n",
|
||
|
"xxxxx yy\n",
|
||
|
"xxxxx yyy\n",
|
||
|
"xxxxx yyyy\n",
|
||
|
"xxxxx yyyyy\n"
|
||
|
]
|
||
|
}
|
||
|
],
|
||
|
"source": [
|
||
|
"# Now... write a loop in a loop\n",
|
||
|
"for x in range(1,6):\n",
|
||
|
" for y in range(1,6):\n",
|
||
|
" print('x'*x, 'y'*y)"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "markdown",
|
||
|
"metadata": {},
|
||
|
"source": [
|
||
|
"---------------------------------------"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "markdown",
|
||
|
"metadata": {},
|
||
|
"source": [
|
||
|
"### ASCII canvas"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "markdown",
|
||
|
"metadata": {},
|
||
|
"source": [
|
||
|
"A not-too-big next step is to start generating patterns."
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "markdown",
|
||
|
"metadata": {},
|
||
|
"source": [
|
||
|
"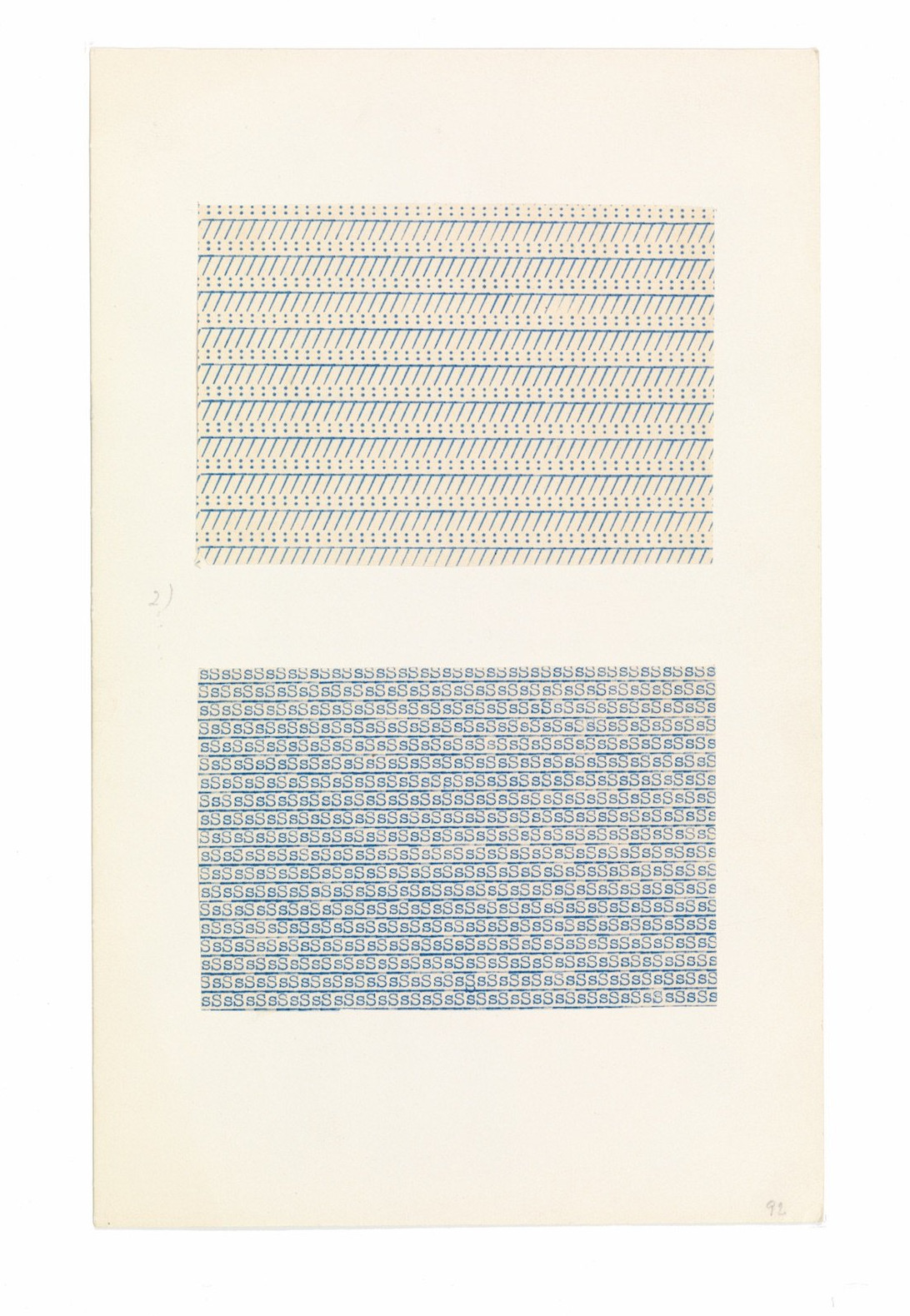\n",
|
||
|
"\n",
|
||
|
"> These varied experiments in articulation are to be understood not as an end in themselves but merely as a help to us in gaining new terms in the vocabulary of tactile language. (On Weaving, Anni Albers (1965))\n",
|
||
|
"\n",
|
||
|
"<small>Anni Albers - Typewriter Studies</small>"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": 5,
|
||
|
"metadata": {},
|
||
|
"outputs": [
|
||
|
{
|
||
|
"name": "stdout",
|
||
|
"output_type": "stream",
|
||
|
"text": [
|
||
|
"sSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsS\n",
|
||
|
"____________________________________________________________________________________________________\n",
|
||
|
"sSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsS\n",
|
||
|
"____________________________________________________________________________________________________\n",
|
||
|
"sSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsS\n",
|
||
|
"____________________________________________________________________________________________________\n",
|
||
|
"sSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsS\n",
|
||
|
"____________________________________________________________________________________________________\n",
|
||
|
"sSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsS\n",
|
||
|
"____________________________________________________________________________________________________\n",
|
||
|
"sSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsS\n",
|
||
|
"____________________________________________________________________________________________________\n",
|
||
|
"sSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsS\n",
|
||
|
"____________________________________________________________________________________________________\n",
|
||
|
"sSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsS\n",
|
||
|
"____________________________________________________________________________________________________\n",
|
||
|
"sSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsS\n",
|
||
|
"____________________________________________________________________________________________________\n",
|
||
|
"sSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsS\n",
|
||
|
"____________________________________________________________________________________________________\n",
|
||
|
"sSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsS\n",
|
||
|
"____________________________________________________________________________________________________\n",
|
||
|
"sSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsS\n",
|
||
|
"____________________________________________________________________________________________________\n",
|
||
|
"sSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsS\n",
|
||
|
"____________________________________________________________________________________________________\n",
|
||
|
"sSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsS\n",
|
||
|
"____________________________________________________________________________________________________\n",
|
||
|
"sSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsS\n",
|
||
|
"____________________________________________________________________________________________________\n",
|
||
|
"sSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsS\n",
|
||
|
"____________________________________________________________________________________________________\n",
|
||
|
"sSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsS\n",
|
||
|
"____________________________________________________________________________________________________\n",
|
||
|
"sSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsS\n",
|
||
|
"____________________________________________________________________________________________________\n",
|
||
|
"sSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsS\n",
|
||
|
"____________________________________________________________________________________________________\n",
|
||
|
"sSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsSsS\n",
|
||
|
"____________________________________________________________________________________________________\n"
|
||
|
]
|
||
|
}
|
||
|
],
|
||
|
"source": [
|
||
|
"# We could replicate Anni's typewriter study now:\n",
|
||
|
"width = 100\n",
|
||
|
"height = 20\n",
|
||
|
"for y in range(height):\n",
|
||
|
" print('sS' * 50)\n",
|
||
|
" print('_' * width)"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "markdown",
|
||
|
"metadata": {},
|
||
|
"source": [
|
||
|
"**mini-exercise**: try to replicate the other typewriter study yourself now!"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": null,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": []
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": null,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": []
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": null,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": []
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": null,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": []
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": 9,
|
||
|
"metadata": {},
|
||
|
"outputs": [
|
||
|
{
|
||
|
"name": "stdout",
|
||
|
"output_type": "stream",
|
||
|
"text": [
|
||
|
"▉▓▞▉▉░░▞▚░▞░▉▚▚▒▓▉▉░░▉▉▞░▓▒▓▞▉▚▒▉▒▓▓▉░▉▞▚░▉▒▞▓▒▞▚▚▉▒▓▓▉▒░▓▉▒▞▓░▉░▒▚░▞▞▒▉░▉▚░▓░▞▚▞▓▞░▓▒▓▞░░▉▉▉▞▚▚▉▞▉░\n",
|
||
|
"░▉▓▞▚▞░▉▚▓▞▉▞▉▚▉▚▚▉▓▒▚▓▞░░▞▚░▒▓▞░▓░▚░░▚░▓▓▒▒▓░▚▞▉▉░░▒▚▞▓░▚▉▚▞▚░░▒▓░░▒▉▉▒▉░▓▉░▉▓░▉▞▞▉▉▞░▞▉▚▉░░░▉▓▚░▉▚\n",
|
||
|
"░▓▒▉▓▒░▒▓▚▓▚▞▓▚▚▉▚▓▞▉▞▓▒▉▓▉▓░▉▓▓▓▞░▓▚░▚▉▒▞▚▉▓▒▒░▒▚▉░▉▞▓░▓▞▞▚▉▉▉▞░▒░▉░▓▒░░▚▒▓░▚░▒▒░░▓▉▉▓▒▉▉▚░▉▒▒▞▓░▞▓\n",
|
||
|
"▚▒▒▓░░▉▞▞▓▉▓▉▓░▚▉░▒▚▓▒▚▒▚▚▚▞▉▉▉▚░▉▚▞▒▚▉▓▉░░▞▚▓▒░▉▉▉▞░▒▉▒▞▚▞▓░▒▉▒▒░▉▉▒▓▓░▒▚░▒▞▉▞▞▚▉░░▚▒▉▒▉▓░░▒▒▉▓▉▚▞▚\n",
|
||
|
"▞▞▚▒▓▒▒▞▓▒▚▉▉▓▓▚▚▞▞▚▚▉▓▞▞▒░▓▓▚▓▒▓▓▚▚▚▒▉▉▚▓▉░▓▒▒▓▞▓▒▚▒▚▉░▓▚▉░▉▉░▞▚▞▚▞▒▓░▓░▓▓▚▉▞▞▒▚▞▒▚▞▉▓▞▚▞▓▞▒▉░░▓▓▓▒\n",
|
||
|
"░▞▓▒▉▒▒▉▞▚▉▒▓▚▞▒▚▚▓▞▉▒▚▞▓▒▉▞▓▒▓▚▞▞▒░▓░░▞▓▓▚▞▓░▞░▚▚░▒▓▚▒▒▚▒░▉▞▞░░▉▞▒▞▚▓▓░▉▉▞▚▚▞▞▓▒░▞▞▒▚░▓▓▓▉▞▚░░▚░▉▚▞\n",
|
||
|
"▒▉▒▒▓░▚▚▉▒▚▒▞▞▓▉▓▉▞▚▉▉▓▒░▒▒░▓▒▚▒▓▓▞▒▓▒▓▚▒░▞▉▉▒░▚░▓░▓▒▓▞▓▓▒▉▉▚▒▞▚▉▉░▚▞▚░▚▓░▉▞▉▚▚▉▞▞▓▒▓▉▓▞▓▓▉▚▉▉▉▒▒▒▒░\n",
|
||
|
"▒▒░▓▞▒░▓▉▚▚▓░▓▒▞▉▞▓▉▉▉▒▓▞▞░▓▚▒▓▓▉▉▞░░░▒▚▉▚░░▉▞▒▓▒▚▓▓▚▚▓▉▉▚▚▓░▒▓▒▞▉░▉▓░▞▒▒▞▚▚▓░▚▞▓▒▚░░▒▒▉▞░▒▒▉▞▞▓▉▒▚▉\n",
|
||
|
"▞▒▉▓▞▒░▚▞▒░▓▉▚▒▒▉▒▉▒▉░▞▚▒▒▒▉▚▓▚▒░▓▉▞▞░░▒▉▉▒▓▉▞▓▞▞░▒▞▒▚░░▓░▞▓▚▉▓▒▚▞░▓░▚▉▚▚▓▓▓▚▉▉▚▞▒▚▉▞▉▓▒▚▒▉▒░▒▉░▓░░▒\n",
|
||
|
"▞▚▞▉▉▚▉▓▞▒▚▒▚▉▞▉▞▒▓▞▒▚▉░▚▓▒▓▚▞▓▒▞▚▞░▞░▒▉▓▞▞▚▒░▓▉▚░▚▚▉▒▓▉▞▓▒▒▒▓▉░░▉▉▚░▓▉▉▞▒▓▚░▒▉▓▞▓▞▉▓▉▒▒▉▒▓▓▒▞░▞▞▚▓░\n",
|
||
|
"░▒▓▉▚▉▓▓▓▓░▞▞▚▚▚▞▞▒▓▚░░▉░▞▓▒▓▒▞▒░▓▞▞▒▓▒▒▚▚▓▉▒▉░▚▞▞▚▞▚▓░▉▓░▞▞▞▞▒░░▚▒▒░░▚░▚▚░▒▓▒▓▉░░░▓▚▒▞▚▓▒▒▚▉▒░▓▓▒▉░\n",
|
||
|
"▞░▚▞▞▚▚▚▒▒▞▒▒▒▉▞▓▚▉▒▞▚▒▉▒▓░▓░▓▞▚▓▒▚▉▞▞▉░▉▒▓░▞▞▉▉▉░▚░▉▉▉▒▓▉▒▚▒▓▉▞▒▞▓▉▒▓▉▞▚▞▒▚▓▚▚▓▓▞▉▉▒▒▓▒▚▞▚▚▒▞▉▞░▒▒▚\n",
|
||
|
"▚▓▒▚▒▚▒▒░▉▒▓▓▒▚▓▓▒░▚▚▚▚▓░░▚░░▞▒▒▉▞▞▒▉░▚▉░░▞▓░▒▞▉▞▉▚▉▓▉░▚▉▉░▒▞▓▒▞▒▚▒▓░▚░▉▚▓▓░▉▉▓▞░░▉▓▓▉░░▞▚▚▒▚▓▒▞▓▉▉▚\n",
|
||
|
"▚▒▞░░▓▓░▚▉▒▓▒▞▚▓▉░▞▚▒▚▞▒░▓▉░▞░▞░▒▒▒▞▚▚░▒░▒░▓▞▉▚▚▓▚▚▒▚▒▞░▒░▞▒▒▉▉▉▒▓▉▓░░▓▚▉▞▚▉▞▚▚▒░▚▒▞▞▒▒▒▞░░░░▞▞▚▉▓▓░\n",
|
||
|
"▒▓▚░▓░▚░▒░▓▞▒▚▓▓▞░▉▚▞▒▉▓▒▓▒░▚▞▚░▞▚▚░▚░▉░▞▓▞▒▞▓▚▚▒▚▉▞░░▞▓░░▒▓▒▒░▞▞▉▒░▓▒▉▉▚▚░░▉▉▚▞░▓▓▒▉░▚▞▞▓▞▉▓▚▚▚░▉░▒\n",
|
||
|
"▓▞▞░░▉▚░░▉▓▒▓▒▞▞▉▒▉▉▒▒░▞▒▒░▚▞▞░░░▉▉▞░▓▉▞▞░▞▞▉▞▉▒▓░▞▉▒▚▉▞░▞▒▉▒▒▞▓▉▞░▓▉▒▚▞▚▒░▓░▚▉▚▞▞▓▚▉▒▚░▚▒▉▓▉░▓▉░░▞▒\n",
|
||
|
"░▞▞▉▓▞▒▒▓▒▉▉▚▚▓▉▚▚▉▚▒▚░▓▉▚▓▓▓▞▚▚▚▞▒▚▓▒▉▚░░▓▉▓▓▉▉▒░▞░▞▚▉▓▞▉▓▒▚▉▞▞▒▞▉░▓░▚▓▒░▚░░░▒▒▞▚▞▓░▚▒░░░▉▞▉▒▉▉▉▓▞▚\n",
|
||
|
"░▞░▞▓▚▚▚▚▞▚▓▞░▒▓▉▞▚▓▚▚▞▞▞▚▓▉░░▓▞░▞▓▞▉▉▒▞▓▓░░▒▞▚▓▉▚▒▒░▓░▉▒░▓▓▒▚▉▓▞▓▞░░▓▚▉▓▓▞▉▓░░▚▚▉▒▓▓░░▞░▉▒▒▞░▒░▞▞░▚\n",
|
||
|
"▓▉▉▉▒▉▞▉░▉░░▒▓▞▉░▓▚▒▞▞▚▚▓▞▒▒░░▒▞▉▒░▉▞▓▉▉▚▓▓░▞▒▉▒▒▉▉▚▞▓▚░▒▉▞░▒▓▒▒▓▚▓░▚▒▞░▒░░▒▓▞▓▉▚▞▞▚▉▞▚▓▞▒▚▞▉▒▉░▓▞░▒\n",
|
||
|
"▒░▉▞▚▉▞▚░░▉▉▒▉▚▓▒▒░▒▒▞░▚▒▓░▉░▉▉▚▚▞▉▒▞▚▉▞▓▞▚░▉▒▞▉▒▞▚▞░▚▉▒▒▓░░░▚▉▓▉▞▉░▉▞▞▓▓▞▒▞▉▉▚▒▒▞▞▚▚▚▒▉░▒▞▓▒▚▒▓▉▒▚▓\n",
|
||
|
"▉░░▓▚▒▞▒▒▉░▚▉▉░▉▒▒▒░░▞▚▞▓▉▚▓▞▉▉▒▞▉▒▞▒▉▓▞░▞▒▚▒▚▓▓▚▚▚▒▒▓▓▓▞░▓▓▚░▉▉▒▞▓▞▓░▉▞▞▚▓▚░▉▓▚▚▓▓▞▒▉▚▓░░▓▞▒▉▉▞▞░▓▞\n",
|
||
|
"▉▓▚▉░▉▓▞▞░▚▒▓▞▉▞▚▉▉░▒▉▉▉▒░▒▒▚░▒▚▒▓▉▚░▚░▉▞▚▉▓▓▞▒▚▉▚▉▚▞▒▉▚▚▚▒░░▞▉▒▓▉▉▚▓▚▉▞▓▞▒▓▓▞▒▚▓▚░░▚▒▒▚░▉▞▒░▓░▉▓▉▒▓\n",
|
||
|
"▓▚▒▓▚▉▚▉░▓▚▒▓▚░░▚▒▞░░▒▚▓▓▞░▒▒▚▓░▒▞▉▚░░▚▉▚▒░▒▉▚▉▞▞▞▓▞▉▞▒▚░▚▉▒▚▞▓▚▉░▓▚▚░▒▞░▉▞▒▉▉▞▚▞▓▓▓▒▚▉▚▚▉▒▉░▞▉▚▚░▉▒\n",
|
||
|
"▚░▉▓▞░▓▉░▉▞▓▉▓▓▉▉░▚▓▉▞░▓░▚▞▞▚░▓▒▚░▞░▓▚░▞░▓▚▓▉░▞▓▓░▉▒▓▓░▚▓▉▓▞▉▚▒▞▉▚▓▓░░▞▓▚▞▉▓▉▒▓░▉░▉▞░░▞░░▞▒▒▞▚▓▓▉▓▞▞\n",
|
||
|
"▒▓▞▉▒▚▞▓▞▉▞▞░▉░▓▞▓░▓▉▞▉▉▓▓▚▞▚▉▓▞▚▚▓▚▒▒▞▚▞▒▞▒▞▒░░▓▉▒▚▉▓▓▓▒▚▞▓▒▞▓▓▒▞░▞▞▞▚▒▉▓▓▚▉▓░▒▞▞▓░▉░▓▞▓▒▞▓▞░▓░▉▚▚▞\n",
|
||
|
"░▚▞▚░▒▒▓▞▓▉░▓▚▓▉░▉▒░▉▞░▒▒▞▒▉▓▚▉▞▚▚▒▚░▚▞▓▞░▒▉▚▓▒▒▞▚▉░▞░▉▚░░▞▚▓░▞▚░▒▉▉▉▚▓▓▉▒▞▒▓▚░▉░░▚▞▓▞▞▞▚░▉░▓░▉▉▓▓▞░\n",
|
||
|
"▓▚▒░▓▒▞▒░▞▚░▞▉▉▒▞▚▓▞▉▚▓░▞▚▓▓▒▚▞▓▉▚▉▚▒▒▓▒▚░░▉▞▚░▒▞▞▚▓▞▉▓▉▚░▒▒▒▒▚▒▓▞▉▓▚▚▉▒▚░▒▓▉░░░░▉▚▞░▞░░▓░▓▒▚▒░░▓░▚▉\n",
|
||
|
"░▞▞▒▒▉▉▉▞▞▓▓▞▞░▒▒▞▚▚▚▚▞▚▚░▚▓▓▓▓▓▒▚▒▒▓▓▉▉▒▞░▚▓░▞▞▉▚▞▉▚▞▒▉▞▓▚░▓░▒▚▚▒▞▓▒▓▓▒▓▚▒▉▓▚▚▓░▓▉░▚▒▉░▓▒░░▞▓▞░▉▚▒▞\n",
|
||
|
"▒▚▒▞░▚▉░░▞░▓▞▞▚▉░░▞▞▉▞▓▒▚▉▒▉▓▒▓▒▓▒▞▉▞▚▞▉▞▒▓░▚▉▓▞▒▞▉▉▚▉▉▉▞▓▚▚▞▉▓░▒░▞▒▓▒▚▚▒▓▚▞▓▓▞▞▒▚▉▚▞▞▚▓▞▞▚▚▞▓▚░▉░░▉\n",
|
||
|
"▒▉▚▚░▞░░▚▓▞░▉▉▓▓░▓▓▞░▞░▒▚▒▒▉▓▚▞░▒▞▉▉▞▚▉▓▓░▓▚▒▒▒▒▒▚▉▞▚░▉▓▚▞▒░▞▞▒▞▞░▚▓▒▓░▚▚▓▚▞▒▉░▉▉▚░▚▒▚░▞▉▉▒▓▞▓▉▉▒▓▉▚\n"
|
||
|
]
|
||
|
}
|
||
|
],
|
||
|
"source": [
|
||
|
"# Let's continue a bit with this \"canvas-mode\" of working, \n",
|
||
|
"# and let's bring the random function to the table again.\n",
|
||
|
"\n",
|
||
|
"# In order to slowly build a canvas of characters, \n",
|
||
|
"# we will use a variable (called 'line' in this case) to temporary save our line ...\n",
|
||
|
"\n",
|
||
|
"import random\n",
|
||
|
"characters = ['░','▒','▓','▉','▚','▞']\n",
|
||
|
"width = 100\n",
|
||
|
"height = 30\n",
|
||
|
"line = ''\n",
|
||
|
"for y in range(height):\n",
|
||
|
" for x in range(width):\n",
|
||
|
" line += random.choice(characters)\n",
|
||
|
" print(line)\n",
|
||
|
" line = ''"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": 6,
|
||
|
"metadata": {
|
||
|
"scrolled": true
|
||
|
},
|
||
|
"outputs": [
|
||
|
{
|
||
|
"name": "stdout",
|
||
|
"output_type": "stream",
|
||
|
"text": [
|
||
|
"x = 0\n",
|
||
|
" y = 0\n",
|
||
|
" y = 1\n",
|
||
|
" y = 2\n",
|
||
|
" y = 3\n",
|
||
|
" y = 4\n",
|
||
|
" y = 5\n",
|
||
|
" y = 6\n",
|
||
|
" y = 7\n",
|
||
|
" y = 8\n",
|
||
|
" y = 9\n",
|
||
|
"x = 1\n",
|
||
|
" y = 0\n",
|
||
|
" y = 1\n",
|
||
|
" y = 2\n",
|
||
|
" y = 3\n",
|
||
|
" y = 4\n",
|
||
|
" y = 5\n",
|
||
|
" y = 6\n",
|
||
|
" y = 7\n",
|
||
|
" y = 8\n",
|
||
|
" y = 9\n",
|
||
|
"x = 2\n",
|
||
|
" y = 0\n",
|
||
|
" y = 1\n",
|
||
|
" y = 2\n",
|
||
|
" y = 3\n",
|
||
|
" y = 4\n",
|
||
|
" y = 5\n",
|
||
|
" y = 6\n",
|
||
|
" y = 7\n",
|
||
|
" y = 8\n",
|
||
|
" y = 9\n",
|
||
|
"x = 3\n",
|
||
|
" y = 0\n",
|
||
|
" y = 1\n",
|
||
|
" y = 2\n",
|
||
|
" y = 3\n",
|
||
|
" y = 4\n",
|
||
|
" y = 5\n",
|
||
|
" y = 6\n",
|
||
|
" y = 7\n",
|
||
|
" y = 8\n",
|
||
|
" y = 9\n",
|
||
|
"x = 4\n",
|
||
|
" y = 0\n",
|
||
|
" y = 1\n",
|
||
|
" y = 2\n",
|
||
|
" y = 3\n",
|
||
|
" y = 4\n",
|
||
|
" y = 5\n",
|
||
|
" y = 6\n",
|
||
|
" y = 7\n",
|
||
|
" y = 8\n",
|
||
|
" y = 9\n",
|
||
|
"x = 5\n",
|
||
|
" y = 0\n",
|
||
|
" y = 1\n",
|
||
|
" y = 2\n",
|
||
|
" y = 3\n",
|
||
|
" y = 4\n",
|
||
|
" y = 5\n",
|
||
|
" y = 6\n",
|
||
|
" y = 7\n",
|
||
|
" y = 8\n",
|
||
|
" y = 9\n",
|
||
|
"x = 6\n",
|
||
|
" y = 0\n",
|
||
|
" y = 1\n",
|
||
|
" y = 2\n",
|
||
|
" y = 3\n",
|
||
|
" y = 4\n",
|
||
|
" y = 5\n",
|
||
|
" y = 6\n",
|
||
|
" y = 7\n",
|
||
|
" y = 8\n",
|
||
|
" y = 9\n",
|
||
|
"x = 7\n",
|
||
|
" y = 0\n",
|
||
|
" y = 1\n",
|
||
|
" y = 2\n",
|
||
|
" y = 3\n",
|
||
|
" y = 4\n",
|
||
|
" y = 5\n",
|
||
|
" y = 6\n",
|
||
|
" y = 7\n",
|
||
|
" y = 8\n",
|
||
|
" y = 9\n",
|
||
|
"x = 8\n",
|
||
|
" y = 0\n",
|
||
|
" y = 1\n",
|
||
|
" y = 2\n",
|
||
|
" y = 3\n",
|
||
|
" y = 4\n",
|
||
|
" y = 5\n",
|
||
|
" y = 6\n",
|
||
|
" y = 7\n",
|
||
|
" y = 8\n",
|
||
|
" y = 9\n",
|
||
|
"x = 9\n",
|
||
|
" y = 0\n",
|
||
|
" y = 1\n",
|
||
|
" y = 2\n",
|
||
|
" y = 3\n",
|
||
|
" y = 4\n",
|
||
|
" y = 5\n",
|
||
|
" y = 6\n",
|
||
|
" y = 7\n",
|
||
|
" y = 8\n",
|
||
|
" y = 9\n"
|
||
|
]
|
||
|
}
|
||
|
],
|
||
|
"source": [
|
||
|
"# A loop in a loop?\n",
|
||
|
"width = 10\n",
|
||
|
"height = 10\n",
|
||
|
"for y in range(height):\n",
|
||
|
" print('x =', x)\n",
|
||
|
" for x in range(width):\n",
|
||
|
" print(' y =', y)"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": 29,
|
||
|
"metadata": {},
|
||
|
"outputs": [
|
||
|
{
|
||
|
"name": "stdout",
|
||
|
"output_type": "stream",
|
||
|
"text": [
|
||
|
"01234567891011121314151617181920212223242526272829\n",
|
||
|
"01234567891011121314151617181920212223242526272829\n",
|
||
|
"01234567891011121314151617181920212223242526272829\n",
|
||
|
"01234567891011121314151617181920212223242526272829\n",
|
||
|
"01234567891011121314151617181920212223242526272829\n",
|
||
|
"01234567891011121314151617181920212223242526272829\n",
|
||
|
"01234567891011121314151617181920212223242526272829\n",
|
||
|
"01234567891011121314151617181920212223242526272829\n",
|
||
|
"01234567891011121314151617181920212223242526272829\n",
|
||
|
"01234567891011121314151617181920212223242526272829\n",
|
||
|
"01234567891011121314151617181920212223242526272829\n",
|
||
|
"01234567891011121314151617181920212223242526272829\n",
|
||
|
"01234567891011121314151617181920212223242526272829\n",
|
||
|
"01234567891011121314151617181920212223242526272829\n",
|
||
|
"01234567891011121314151617181920212223242526272829\n",
|
||
|
"01234567891011121314151617181920212223242526272829\n",
|
||
|
"01234567891011121314151617181920212223242526272829\n",
|
||
|
"01234567891011121314151617181920212223242526272829\n",
|
||
|
"01234567891011121314151617181920212223242526272829\n",
|
||
|
"01234567891011121314151617181920212223242526272829\n"
|
||
|
]
|
||
|
}
|
||
|
],
|
||
|
"source": [
|
||
|
"# A loop in a loop + collecting characters in a line...\n",
|
||
|
"width = 30\n",
|
||
|
"height = 20\n",
|
||
|
"line = ''\n",
|
||
|
"for y in range(height):\n",
|
||
|
" for x in range(width):\n",
|
||
|
" line += str(x) \n",
|
||
|
" print(line)\n",
|
||
|
" line = '' # try to comment this line out, to see the difference"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": null,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": []
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": null,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": []
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": null,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": []
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": 10,
|
||
|
"metadata": {},
|
||
|
"outputs": [
|
||
|
{
|
||
|
"name": "stdout",
|
||
|
"output_type": "stream",
|
||
|
"text": [
|
||
|
"------------------------------------------------------------++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++\n",
|
||
|
"------------------------------------------------------------++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++\n",
|
||
|
"------------------------------------------------------------++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++\n",
|
||
|
"------------------------------------------------------------++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++\n",
|
||
|
"------------------------------------------------------------++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++\n",
|
||
|
"------------------------------------------------------------++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++\n",
|
||
|
"------------------------------------------------------------++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++\n",
|
||
|
"------------------------------------------------------------++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++\n",
|
||
|
"------------------------------------------------------------++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++\n",
|
||
|
"------------------------------------------------------------++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++\n",
|
||
|
"------------------------------------------------------------++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++\n",
|
||
|
"------------------------------------------------------------++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++\n",
|
||
|
"------------------------------------------------------------++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++\n",
|
||
|
"------------------------------------------------------------++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++\n",
|
||
|
"------------------------------------------------------------++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++\n",
|
||
|
"------------------------------------------------------------++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++\n",
|
||
|
"------------------------------------------------------------++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++\n",
|
||
|
"------------------------------------------------------------++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++\n",
|
||
|
"------------------------------------------------------------++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++\n",
|
||
|
"------------------------------------------------------------++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++\n"
|
||
|
]
|
||
|
}
|
||
|
],
|
||
|
"source": [
|
||
|
"# More patterns, more ways of drawing in this canvas ...\n",
|
||
|
"width = 60\n",
|
||
|
"height = 20\n",
|
||
|
"line = ''\n",
|
||
|
"for y in range(height):\n",
|
||
|
" # here i collect all the characters for one line\n",
|
||
|
" line += '-' * width\n",
|
||
|
" line += '+' * width\n",
|
||
|
" \n",
|
||
|
" # print & reset\n",
|
||
|
" print(line)\n",
|
||
|
" line = ''\n"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": null,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": []
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": 17,
|
||
|
"metadata": {},
|
||
|
"outputs": [
|
||
|
{
|
||
|
"name": "stdout",
|
||
|
"output_type": "stream",
|
||
|
"text": [
|
||
|
"??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? \n",
|
||
|
" !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!!\n",
|
||
|
"??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? \n",
|
||
|
" !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!!\n",
|
||
|
"??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? \n",
|
||
|
" !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!!\n",
|
||
|
"??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? \n",
|
||
|
" !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!!\n",
|
||
|
"??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? \n",
|
||
|
" !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!!\n",
|
||
|
"??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? \n",
|
||
|
" !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!!\n",
|
||
|
"??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? \n",
|
||
|
" !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!!\n",
|
||
|
"??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? \n",
|
||
|
" !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!!\n",
|
||
|
"??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? \n",
|
||
|
" !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!!\n",
|
||
|
"??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? ??? \n",
|
||
|
" !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!! !!!\n"
|
||
|
]
|
||
|
}
|
||
|
],
|
||
|
"source": [
|
||
|
"# or another one\n",
|
||
|
"width = 20\n",
|
||
|
"height = 20\n",
|
||
|
"line = ''\n",
|
||
|
"\n",
|
||
|
"for y in range(height):\n",
|
||
|
" \n",
|
||
|
" # here i collect all the characters for one line\n",
|
||
|
" for x in range(width):\n",
|
||
|
" \n",
|
||
|
" # check if the line \"number\" is odd or even\n",
|
||
|
" # to do this, you can use the \"%\", called the \"modulo\"\n",
|
||
|
" if y % 2 == 0:\n",
|
||
|
" line += '???'\n",
|
||
|
" line += ' '\n",
|
||
|
" else:\n",
|
||
|
" line += ' '\n",
|
||
|
" line += '!!!'\n",
|
||
|
" \n",
|
||
|
" # print & reset\n",
|
||
|
" print(line)\n",
|
||
|
" line = ''"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": null,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": []
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": null,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": []
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": null,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": []
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": null,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": []
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": null,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": []
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": null,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": []
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": 18,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": [
|
||
|
"# Let's also make a pattern that exceeds the width of a single line\n",
|
||
|
"# Here, we will first make one long line ...\n",
|
||
|
"sentence = 'weaving with words and code'\n",
|
||
|
"line = ''\n",
|
||
|
"for x in range(100):\n",
|
||
|
" line += sentence\n",
|
||
|
" line += ' ' * x"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": 23,
|
||
|
"metadata": {
|
||
|
"scrolled": true
|
||
|
},
|
||
|
"outputs": [
|
||
|
{
|
||
|
"name": "stdout",
|
||
|
"output_type": "stream",
|
||
|
"text": [
|
||
|
"weaving with words and codeweaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code weaving with words and code
|
||
|
]
|
||
|
}
|
||
|
],
|
||
|
"source": [
|
||
|
"print(line)"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": 59,
|
||
|
"metadata": {},
|
||
|
"outputs": [
|
||
|
{
|
||
|
"name": "stdout",
|
||
|
"output_type": "stream",
|
||
|
"text": [
|
||
|
"weaving with words and codeweaving with words and code weaving with words and code weaving with wo\n",
|
||
|
"ds and code weaving with words and code weaving with words and code weaving with words and\n",
|
||
|
"code weaving with words and code weaving with words and code weaving with words a\n",
|
||
|
"d code weaving with words and code weaving with words and code weaving w\n",
|
||
|
"th words and code weaving with words and code weaving with words and code \n",
|
||
|
" weaving with words and code weaving with words and code weav\n",
|
||
|
"ng with words and code weaving with words and code weaving with wo\n",
|
||
|
"ds and code weaving with words and code weaving with words and\n",
|
||
|
"code weaving with words and code weaving with words and co\n",
|
||
|
"e weaving with words and code weaving with words and c\n",
|
||
|
"de weaving with words and code weaving with words \n",
|
||
|
"nd code weaving with words and code weaving wi\n",
|
||
|
"h words and code weaving with words and code \n",
|
||
|
" weaving with words and code weaving with words and code \n",
|
||
|
" weaving with words and code weaving with words a\n",
|
||
|
"d code weaving with words and code \n",
|
||
|
" weaving with words and code weaving with words and code \n",
|
||
|
" weaving with words and code weavi\n",
|
||
|
"g with words and code weaving with words and code \n",
|
||
|
" weaving with words and code weav\n",
|
||
|
"ng with words and code weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code weaving with words and\n",
|
||
|
"code weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
"eaving with words and code weaving with words and c\n",
|
||
|
"de weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code weaving wi\n",
|
||
|
"h words and code weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code weaving w\n",
|
||
|
"th words and code weaving with words and\n",
|
||
|
"code weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
"weaving with words and code \n",
|
||
|
"eaving with words and code \n",
|
||
|
"eaving with words and code \n",
|
||
|
"weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and\n",
|
||
|
"code weaving w\n",
|
||
|
"th words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving wi\n",
|
||
|
"h words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and code \n",
|
||
|
" weaving with words and c\n",
|
||
|
"de \n",
|
||
|
"eaving with words and code \n",
|
||
|
" weaving with words and code \n"
|
||
|
]
|
||
|
}
|
||
|
],
|
||
|
"source": [
|
||
|
"# ... and then cut it up into lines of 100 characters, to show the pattern nicely, \n",
|
||
|
"tmp_line = ''\n",
|
||
|
"for character in line:\n",
|
||
|
" if len(tmp_line) < 99:\n",
|
||
|
" tmp_line += character\n",
|
||
|
" else: \n",
|
||
|
" print(tmp_line)\n",
|
||
|
" tmp_line = ''\n",
|
||
|
" count = 0"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": null,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": []
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": null,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": []
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": null,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": []
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": 20,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": [
|
||
|
"# Try to make some more patterns!"
|
||
|
]
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": null,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": []
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": null,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": []
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": null,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": []
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": null,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": []
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": null,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": []
|
||
|
},
|
||
|
{
|
||
|
"cell_type": "code",
|
||
|
"execution_count": null,
|
||
|
"metadata": {},
|
||
|
"outputs": [],
|
||
|
"source": []
|
||
|
}
|
||
|
],
|
||
|
"metadata": {
|
||
|
"kernelspec": {
|
||
|
"display_name": "Python 3",
|
||
|
"language": "python",
|
||
|
"name": "python3"
|
||
|
},
|
||
|
"language_info": {
|
||
|
"codemirror_mode": {
|
||
|
"name": "ipython",
|
||
|
"version": 3
|
||
|
},
|
||
|
"file_extension": ".py",
|
||
|
"mimetype": "text/x-python",
|
||
|
"name": "python",
|
||
|
"nbconvert_exporter": "python",
|
||
|
"pygments_lexer": "ipython3",
|
||
|
"version": "3.7.3"
|
||
|
}
|
||
|
},
|
||
|
"nbformat": 4,
|
||
|
"nbformat_minor": 4
|
||
|
}
|